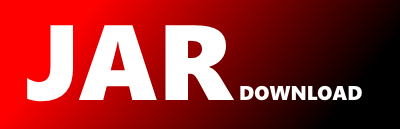
com.jelastic.api.development.response.interfaces.ArrayResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: a471f7ce9566b4814966db0e01d15aca*/
package com.jelastic.api.development.response.interfaces;
import com.jelastic.api.Response;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ArrayResponse extends Response {
public static final String PRIVATE_LIB_PACKAGE_PREFIX = "com." + "hivext";
public static final String PUBLIC_LIB_PACKAGE_PREFIX = "com.jelastic";
public static final String SERVER_PACKAGE = ".server";
public static final String CLASS_NAME = "className";
private Collection array;
private Class className;
private String name = "array";
public ArrayResponse() {
super(OK);
this.array = new ArrayList();
}
public ArrayResponse(int result, String error) {
super(result, error);
}
public ArrayResponse(Class generic, Collection extends ArrayItem> array) {
super(OK);
this.className = generic;
this.array = (Collection) array;
}
public ArrayResponse(String name, Class generic, Collection extends ArrayItem> array) {
super(OK);
this.name = name;
this.className = generic;
this.array = (Collection) array;
}
public Collection getArray() {
return array;
}
public void setArray(Collection extends ArrayItem> array) {
this.array = (Collection) array;
}
public Class getClassName() {
return className;
}
public void setClassName(Class className) {
this.className = className;
}
@Override
protected ArrayResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(CLASS_NAME)) {
String clazzName = getClazzName(json);
try {
className = Class.forName(clazzName);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
}
}
array = new ArrayList();
if (!json.has(name)) {
Iterator i = json.keys();
while (i.hasNext()) {
String key = String.valueOf(i.next());
Object value = json.get(key);
if (value instanceof JSONArray) {
name = key;
break;
}
}
}
if (json.has(name)) {
JSONArray arrayJson = json.getJSONArray(name);
for (int i = 0; i < arrayJson.length(); i++) {
try {
Object item = className.newInstance();
array.add(((ArrayItem) item)._fromJSON(arrayJson.getJSONObject(i)));
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
return this;
}
private String getClazzName(JSONObject json) throws JSONException {
boolean isServerClass = this.getClass().getPackage().toString().contains(SERVER_PACKAGE);
String classNameFromJSON = json.getString(CLASS_NAME);
if (this.getClass().getPackage().getName().startsWith(PUBLIC_LIB_PACKAGE_PREFIX)) {
return classNameFromJSON.replace(PRIVATE_LIB_PACKAGE_PREFIX, PUBLIC_LIB_PACKAGE_PREFIX).replace(SERVER_PACKAGE, "");
}
return isServerClass ? classNameFromJSON : classNameFromJSON.replace(SERVER_PACKAGE, "");
}
@Override
protected JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (array != null) {
JSONArray arrayJson = new JSONArray();
for (ArrayItem item : array) {
arrayJson.put(item._toJSON());
}
if (className != null) {
json.put(CLASS_NAME, className.getCanonicalName());
}
json.put(name, arrayJson);
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy