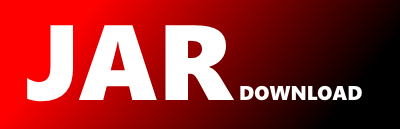
com.jelastic.api.environment.po.RemouteFile Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 22cd772f146860c60c9d82b242d830eb*/
package com.jelastic.api.environment.po;
import java.io.File;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class RemouteFile extends File {
private boolean isDir;
private String name;
private String absolutePath;
private String permission;
private long length = -1;
private boolean isRoot;
private String sourcePath;
private String sourceHost;
private String protocol;
private Integer sourceNodeId;
private boolean isExported;
private String lastModified;
private String fileType;
public RemouteFile() {
super("/default");
}
public RemouteFile(String path, String name, String permission, boolean isDir, long length) {
super(path);
this.absolutePath = path;
this.name = name;
this.isDir = isDir;
this.length = length;
this.permission = permission;
this.lastModified = "";
}
public RemouteFile(String path, String name, String permission, boolean isDir, long length, String lastModified, String fileType) {
super(path);
this.absolutePath = path;
this.name = name;
this.isDir = isDir;
this.length = length;
this.permission = permission;
this.lastModified = lastModified;
this.fileType = fileType;
}
public RemouteFile(String path, String name, String permission, boolean isDir, long length, String sourcePath, String sourceHost, String protocol, Integer sourceNodeId, boolean isExported) {
super(path);
this.absolutePath = path;
this.name = name;
this.isDir = isDir;
this.length = length;
this.permission = permission;
this.sourcePath = sourcePath;
this.sourceHost = sourceHost;
this.protocol = protocol;
this.sourceNodeId = sourceNodeId;
this.isExported = isExported;
}
public RemouteFile(String path, String name, String permission, boolean isDir, long length, String sourcePath, String sourceHost, String protocol, Integer sourceNodeId) {
super(path);
this.absolutePath = path;
this.name = name;
this.isDir = isDir;
this.length = length;
this.permission = permission;
this.sourcePath = sourcePath;
this.sourceHost = sourceHost;
this.protocol = protocol;
this.sourceNodeId = sourceNodeId;
}
public RemouteFile(String path, String name, String permission, boolean isDir, long length, boolean isRoot) {
super(path);
this.absolutePath = path;
this.name = name;
this.isDir = isDir;
this.length = length;
this.permission = permission;
this.isRoot = isRoot;
}
@Override
public String getName() {
return name;
}
@Override
public String getAbsolutePath() {
return getPath();
}
@Override
public String getPath() {
return this.absolutePath;
}
public String getPermission() {
return permission;
}
public void setPermission(String permissions) {
this.permission = permissions;
}
@Override
public boolean isDirectory() {
return isDir;
}
@Override
public long length() {
return length;
}
public void setName(String name) {
this.name = name;
}
public void setPath(String path) {
this.absolutePath = path;
}
public boolean isDir() {
return isDir;
}
public void setIsDir(boolean isDir) {
this.isDir = isDir;
}
public void setLength(long length) {
this.length = length;
}
public boolean isRoot() {
return isRoot;
}
public void setRoot(boolean isRoot) {
this.isRoot = isRoot;
}
public String getSourceHost() {
return sourceHost;
}
public void setSourceHost(String sourceHost) {
this.sourceHost = sourceHost;
}
public String getSourcePath() {
return sourcePath;
}
public void setSourcePath(String sourcePath) {
this.sourcePath = sourcePath;
}
public String getProtocol() {
return protocol;
}
public void setProtocol(String protocol) {
this.protocol = protocol;
}
public Integer getSourceNodeId() {
return sourceNodeId;
}
public void setSourceNodeId(Integer sourceNodeId) {
this.sourceNodeId = sourceNodeId;
}
public boolean isExported() {
return isExported;
}
public void setExported(boolean isExported) {
this.isExported = isExported;
}
public String getLastModified() {
return lastModified;
}
public void setLastModified(String lastModified) {
this.lastModified = lastModified;
}
public String getFileType() {
return fileType;
}
public void setFileType(String fileType) {
this.fileType = fileType;
}
@Override
public String toString() {
return length() + " path:" + getPath() + " isDir:" + isDirectory() + " name:" + getName() + " permission:" + getPermission() + " isExported:" + isExported();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy