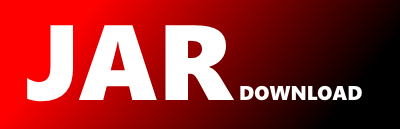
com.jelastic.api.environment.response.ArrayExtIPAddressResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: fc451953f38c2a76487ce49048ca81ce*/
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.system.persistence.ExtIpAddress;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ArrayExtIPAddressResponse extends Response {
private static final String IPS = "ips";
private static final String DETACHED_IPS = "detachedIps";
private static final String ATTACHED_IPS = "attachedIps";
private Map> ipAddresses = new HashMap<>();
public ArrayExtIPAddressResponse() {
}
public ArrayExtIPAddressResponse(List ips) {
super(Response.OK);
this.ipAddresses = new HashMap<>();
this.ipAddresses.put(IPS, ips);
}
public ArrayExtIPAddressResponse(List resultIps, List changedIps, boolean isAttached) {
this(resultIps);
String key = (isAttached ? ATTACHED_IPS : DETACHED_IPS);
this.ipAddresses.put(key, changedIps);
}
public ArrayExtIPAddressResponse(int result, String error) {
super(result, error);
}
public ArrayExtIPAddressResponse(Response response) {
super(response.getResult(), response.getError());
}
public List getValues() {
return ipAddresses.get(IPS);
}
@Override
protected JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
Set>> set = ipAddresses.entrySet();
for (Map.Entry> value : set) {
JSONArray array = new JSONArray();
String key = value.getKey();
List ips = value.getValue();
if (key != null && ips != null && !ips.isEmpty()) {
for (ExtIpAddress ip : ips) {
array.put(ip.getIpAddress());
}
json.put(key, array);
}
}
return json;
}
@Override
protected ArrayExtIPAddressResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
ipAddresses = new HashMap<>();
if (json.has(IPS)) {
fillIpsMap(json, IPS);
}
if (json.has(ATTACHED_IPS)) {
fillIpsMap(json, ATTACHED_IPS);
}
if (json.has(DETACHED_IPS)) {
fillIpsMap(json, DETACHED_IPS);
}
return this;
}
private void fillIpsMap(JSONObject json, String key) throws JSONException {
JSONArray array = json.getJSONArray(key);
ArrayList values = new ArrayList<>();
for (int i = 0; i < array.length(); i++) {
values.add(new ExtIpAddress(array.getString(i), true));
}
if (values.size() > 0) {
ipAddresses.put(key, values);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy