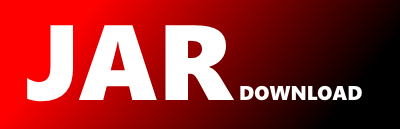
com.jelastic.api.environment.response.ArrayResponse Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Collection;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author Alexey Shponarsky
*/
public class ArrayResponse extends Response {
private Collection array;
private Class className;
public static final String ARRAY_JSON = "array";
public ArrayResponse() {
super(OK);
this.array = new ArrayList();
}
public ArrayResponse(int result, String error) {
super(result, error);
}
public ArrayResponse(Class generic, Collection extends ArrayItem> array) {
super(OK);
this.className = generic;
this.array = (Collection) array;
}
public Collection getArray() {
return array;
}
public void setArray(Collection extends ArrayItem> array) {
this.array = (Collection) array;
}
public Class getClassName() {
return className;
}
@Override
protected ArrayResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has("className")) {
String clazzName = json.getString("className").replace(".server", "").replace(".hivext", ".jelastic");
try {
className = Class.forName(clazzName);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
Logger.getLogger(ArrayResponse.class.getName()).log(Level.SEVERE, null, ex);
}
}
array = new ArrayList();
if (json.has(ARRAY_JSON)) {
JSONArray arrayJson = json.getJSONArray(ARRAY_JSON);
for (int i = 0; i < arrayJson.length(); i++) {
try {
Object item = className.newInstance();
array.add(((ArrayItem) item)._fromJSON(arrayJson.getJSONObject(i)));
} catch (Exception ex) {
ex.printStackTrace();
Logger.getLogger(ArrayResponse.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
return this;
}
@Override
protected JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (array != null) {
JSONArray arrayJson = new JSONArray();
for (ArrayItem item : array) {
arrayJson.put(item._toJSON());
}
if (className != null) {
json.put("className", className.getCanonicalName());
}
json.put(ARRAY_JSON, arrayJson);
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy