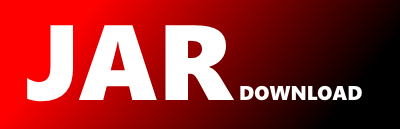
com.jelastic.api.environment.response.DependenciesResponse Maven / Gradle / Ivy
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.data.po.MountPoint;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author Maksym Shevchuk
*/
public class DependenciesResponse extends Response {
public static final String EXPORTS = "exports";
public static final String MOUNTS = "mounts";
public static final String ARRAY = "array";
public static final String NODE_ID = "nodeId";
Map> exports = new HashMap<>();
Map> mounts = new HashMap<>();
public DependenciesResponse() {
super(OK);
this.exports = new HashMap>();
}
public DependenciesResponse(int result, String error) {
super(result, error);
}
public DependenciesResponse(Map> exports, Map> mounts) {
super(OK);
this.exports = exports;
this.mounts = mounts;
}
public Map> getExports() {
return exports;
}
public void setExports(Map> exports) {
this.exports = exports;
}
public Map> getMounts() {
return exports;
}
public void setMounts(Map> mounts) {
this.mounts = mounts;
}
@Override
protected DependenciesResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(EXPORTS)) {
JSONArray exportsJsonArray = json.getJSONArray(EXPORTS);
for (int i = 0; i < exportsJsonArray.length(); i++) {
JSONObject exportJsonObject = exportsJsonArray.getJSONObject(i);
if (exportJsonObject.has(NODE_ID) && exportJsonObject.has(ARRAY)) {
List exportsPerNode = new ArrayList<>();
Integer nodeId = exportJsonObject.getInt(NODE_ID);
JSONArray arrayJson = exportJsonObject.getJSONArray(ARRAY);
for (int j = 0; j < arrayJson.length(); j++) {
exportsPerNode.add(new MountPoint()._fromJSON(arrayJson.getJSONObject(i)));
}
if (!exportsPerNode.isEmpty()) {
exports.put(nodeId, exportsPerNode);
}
}
}
}
if (json.has(MOUNTS)) {
JSONArray mountsJsonArray = json.getJSONArray(MOUNTS);
for (int i = 0; i < mountsJsonArray.length(); i++) {
JSONObject mountJsonObject = mountsJsonArray.getJSONObject(i);
if (mountJsonObject.has(NODE_ID) && mountJsonObject.has(ARRAY)) {
List mountsPerNode = new ArrayList<>();
Integer nodeId = mountJsonObject.getInt(NODE_ID);
JSONArray arrayJson = mountJsonObject.getJSONArray(ARRAY);
for (int j = 0; j < arrayJson.length(); j++) {
mountsPerNode.add(new MountPoint()._fromJSON(arrayJson.getJSONObject(i)));
}
if (!mountsPerNode.isEmpty()) {
mounts.put(nodeId, mountsPerNode);
}
}
}
}
return this;
}
@Override
protected JSONObject _toJSON() throws JSONException {
JSONObject responseJson = super._toJSON();
if (exports != null) {
JSONArray exportsJsonArray = new JSONArray();
for (Integer nodeId : exports.keySet()) {
List exportsPerNode = exports.get(nodeId);
if (exportsPerNode == null) {
continue;
}
JSONObject exportJsonObject = new JSONObject();
exportJsonObject.put(NODE_ID, nodeId);
JSONArray arrayJson = new JSONArray();
for (MountPoint item : exportsPerNode) {
arrayJson.put(item._toJSON());
}
exportJsonObject.put(ARRAY, arrayJson);
exportsJsonArray.put(exportJsonObject);
}
responseJson.put(EXPORTS, exportsJsonArray);
}
if (mounts != null) {
JSONArray mountsJsonArray = new JSONArray();
for (Integer nodeId : mounts.keySet()) {
List mountsPerNode = mounts.get(nodeId);
if (mountsPerNode == null) {
continue;
}
JSONObject mountJsonObject = new JSONObject();
mountJsonObject.put(NODE_ID, nodeId);
JSONArray arrayJson = new JSONArray();
for (MountPoint item : mountsPerNode) {
arrayJson.put(item._toJSON());
}
mountJsonObject.put(ARRAY, arrayJson);
mountsJsonArray.put(mountJsonObject);
}
responseJson.put(MOUNTS, mountsJsonArray);
}
return responseJson;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy