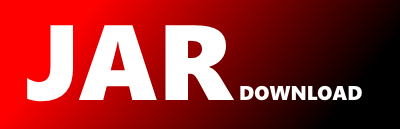
com.jelastic.api.environment.response.DomainsResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: dd026ccb20ca1666623091892f2466e5*/
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class DomainsResponse extends Response {
private static final String ENV = "env";
private static final String NODE_GROUPS = "nodeGroups";
private static final String NODE_GROUP = "nodeGroup";
private static final String NODES = "nodes";
private static final String NODE_ID = "nodeId";
private static final String DOMAINS = "domains";
private Set envDomains;
private Map> nodeGroupsDomains;
private Map> nodesDomains;
public DomainsResponse() {
super(OK);
}
public DomainsResponse(int result, String error) {
super(result, error);
}
public Set getEnvDomains() {
return envDomains;
}
public void setEnvDomains(Set envDomains) {
this.envDomains = envDomains;
}
public Map> getNodeGroupsDomains() {
return nodeGroupsDomains;
}
public void setNodeGroupsDomains(Map> nodeGroupsDomains) {
this.nodeGroupsDomains = nodeGroupsDomains;
}
public Map> getNodesDomains() {
return nodesDomains;
}
public void setNodesDomains(Map> nodesDomains) {
this.nodesDomains = nodesDomains;
}
@Override
public DomainsResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(ENV)) {
envDomains = convertJsonArrayToSet(json.getJSONArray(ENV));
}
nodeGroupsDomains = fillDomainsMap(json, NODE_GROUPS, NODE_GROUP);
nodesDomains = fillDomainsMap(json, NODES, NODE_ID);
return this;
}
private Map> fillDomainsMap(JSONObject json, String name, String idName) throws JSONException {
Map> domainsMap = new HashMap<>();
if (json.has(name)) {
JSONArray jsonArray = json.getJSONArray(name);
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
String key = jsonObject.getString(idName);
domainsMap.put(key, convertJsonArrayToSet(jsonObject.getJSONArray(DOMAINS)));
}
}
return domainsMap;
}
private JSONArray getDomainsJSONArray(Map> domains, String idName, boolean convertIdValuetoInt) throws JSONException {
JSONArray jsonArray = new JSONArray();
for (Map.Entry> entry : domains.entrySet()) {
JSONObject jsonObject = new JSONObject();
String idValue = entry.getKey();
jsonObject.put(idName, convertIdValuetoInt ? Integer.valueOf(idValue) : idValue);
jsonObject.put(DOMAINS, entry.getValue());
jsonArray.put(jsonObject);
}
return jsonArray;
}
private JSONArray getDomainsJSONArray(Map> domains, String idName) throws JSONException {
return getDomainsJSONArray(domains, idName, false);
}
private Set convertJsonArrayToSet(JSONArray jsonArray) {
return IntStream.range(0, jsonArray.length()).mapToObj(i -> {
try {
return jsonArray.getString(i);
} catch (JSONException e) {
return "";
}
}).filter(i -> !i.isEmpty()).collect(Collectors.toSet());
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (envDomains != null) {
json.put(ENV, envDomains);
}
if (nodeGroupsDomains != null) {
json.put(NODE_GROUPS, getDomainsJSONArray(nodeGroupsDomains, NODE_GROUP));
}
if (nodesDomains != null) {
json.put(NODES, getDomainsJSONArray(nodesDomains, NODE_ID, true));
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy