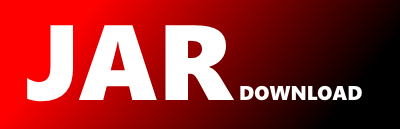
com.jelastic.api.environment.response.EnvironmentInfoResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: aa83cf583c5cb239a143dccc18342428*/
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.data.po.EnvsCriteria;
import com.jelastic.api.data.po.MountPoint;
import com.jelastic.api.system.service.utils.NodeUtils;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.jelastic.api.system.persistence.*;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class EnvironmentInfoResponse extends Response implements Comparable {
public final static String ENV = "env";
public final static String NODES = "nodes";
public final static String SOFT_NODE_GROUPS = "softNodeGroups";
public final static String ENV_GROUPS = "envGroups";
public final static String NODE_GROUPS = "nodeGroups";
public static final String MOUNTPOINTS = "mountpoints";
public static final String TRIGGERS = "triggers";
public static final String NODES_DATA = "nodesData";
public static final String NODE_ID = "nodeId";
public static final String URL = "url";
public static final String NODES_INTERNAL_DOMAINS = "nodesInternalDomains";
public static final String DOMAINS = "domains";
private boolean isExport = false;
private AppNodes env;
private Set nodes;
private Set softNodeGroups;
private Set envGroups = new HashSet<>();
private EnvsCriteria criteria;
private Integer uid;
private Set mountpoints;
private Set triggers;
private Map nodesData;
private Map> nodesInternalDomains;
public EnvironmentInfoResponse(Response response) {
super(response.getResult(), response.getError());
}
public EnvironmentInfoResponse() {
super(OK);
}
public EnvironmentInfoResponse(int result, String error) {
super(result, error);
}
public EnvironmentInfoResponse(int result, String error, String appid) {
super(result, error);
env = new AppNodes();
env.setAppid(appid);
}
public EnvironmentInfoResponse(AppNodes appNodes, Integer uid) {
this(appNodes, null, uid, null, false);
}
public EnvironmentInfoResponse(AppNodes appNodes, Integer uid, Collection sharedEnvGroups) {
this(appNodes, null, uid, sharedEnvGroups, false);
}
public EnvironmentInfoResponse(AppNodes appNodes, List regionResellers, Integer uid, Collection sharedEnvGroups, boolean lazy) {
super(Response.OK);
this.env = appNodes;
String zone = env.getZone();
long start = System.currentTimeMillis();
if (!lazy) {
for (SoftwareNode softwareNode : appNodes.getNodes()) {
String adminUrl = NodeUtils.makeAdminNodeUrl(softwareNode, env.getDomain().getDomain(), zone, env.getSslState(), softwareNode.isExtIpPresent());
softwareNode.setUrl(adminUrl);
softwareNode.setAdminUrl(NodeUtils.getAdminUrl(softwareNode, NodeUtils.createNodeUrl(env.getDomain().getDomain(), zone, softwareNode, softwareNode.isExtIpPresent(), env.getSslState())));
if (softwareNode.getEndpoints() != null) {
for (Endpoint endpoint : softwareNode.getEndpoints()) {
endpoint.setDomain(adminUrl);
}
}
}
}
long time = System.currentTimeMillis() - start;
start = System.currentTimeMillis();
if (lazy) {
this.env.setNodes(null);
} else {
this.nodes = appNodes.getNodes();
this.softNodeGroups = appNodes.getSoftNodeGroups();
}
this.uid = uid;
this.envGroups = new HashSet<>();
if (this.uid != null) {
for (EnvironmentGroup envGroup : appNodes.getGroups()) {
if (sharedEnvGroups != null && !sharedEnvGroups.contains(envGroup.getId())) {
continue;
}
envGroups.add(envGroup.getGroupFullName());
}
}
time = System.currentTimeMillis() - start;
}
public AppNodes getAppNodes() {
return env;
}
public void setAppNodes(AppNodes appNodes) {
this.env = appNodes;
}
public AppNodes getEnv() {
return env;
}
public void setEnv(AppNodes env) {
this.env = env;
}
public Set getNodes() {
return nodes;
}
public void setNodes(Set nodes) {
this.nodes = nodes;
}
public void setCriteria(EnvsCriteria criteria) {
this.criteria = criteria;
}
public boolean isExport() {
return isExport;
}
public void setExport(boolean export) {
isExport = export;
}
public Set getMountpoints() {
return mountpoints;
}
public void setMountpoints(Set mountpoints) {
this.mountpoints = mountpoints;
}
public Set getTriggers() {
return triggers;
}
public void setTriggers(Set triggers) {
this.triggers = triggers;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (this.env != null) {
json.put(ENV, this.env.toJSON(this.isExport));
}
if (nodes != null) {
JSONArray nodesJson = new JSONArray();
for (SoftwareNode node : nodes) {
if (node.isDeleted()) {
}
nodesJson.put(node.toJSON(this.isExport));
}
json.put(NODES, nodesJson);
}
if (softNodeGroups != null) {
JSONArray nodeGroupsArray = new JSONArray();
for (SoftNodeGroup softNodeGroup : softNodeGroups) {
JSONObject nodeGroupJson = softNodeGroup.toJSON(this.isExport);
nodeGroupsArray.put(nodeGroupJson);
}
json.put(NODE_GROUPS, nodeGroupsArray);
}
if (envGroups != null) {
JSONArray envGroupsArray = new JSONArray();
for (String envGroup : envGroups) {
envGroupsArray.put(envGroup);
}
json.put(ENV_GROUPS, envGroupsArray);
}
if (mountpoints != null) {
JSONArray mountpointsArray = new JSONArray();
for (MountPoint mountpoint : mountpoints) {
mountpointsArray.put(mountpoint._toJSON());
}
json.put(MOUNTPOINTS, mountpointsArray);
}
if (triggers != null) {
JSONArray triggersArray = new JSONArray();
for (Trigger trigger : triggers) {
triggersArray.put(trigger._toJSON());
}
json.put(TRIGGERS, triggersArray);
}
if (nodesData != null) {
JSONArray nodesDataArray = new JSONArray();
for (Integer nodeId : nodesData.keySet()) {
JSONObject nodeDataJsonObject = new JSONObject();
nodeDataJsonObject.put(NODE_ID, nodeId);
nodeDataJsonObject.put(URL, nodesData.get(nodeId));
nodesDataArray.put(nodeDataJsonObject);
}
json.put(NODES_DATA, nodesDataArray);
}
if (nodesInternalDomains != null) {
JSONArray nodesInternalDomainsArray = new JSONArray();
for (Integer nodeId : nodesInternalDomains.keySet()) {
JSONObject nodeInternalDomainsJsonObject = new JSONObject();
nodeInternalDomainsJsonObject.put(NODE_ID, nodeId);
nodeInternalDomainsJsonObject.put(DOMAINS, nodesInternalDomains.get(nodeId));
nodesInternalDomainsArray.put(nodeInternalDomainsJsonObject);
}
json.put(NODES_INTERNAL_DOMAINS, nodesInternalDomainsArray);
}
return json;
}
public Set getSoftNodeGroups() {
return softNodeGroups;
}
public void setSoftNodeGroups(Set softNodeGroups) {
this.softNodeGroups = softNodeGroups;
}
public Set getEnvGroups() {
return envGroups;
}
public void setEnvGroups(Set envGroups) {
this.envGroups = envGroups;
}
public Map getNodesData() {
return nodesData;
}
public void setNodesData(Map nodesData) {
this.nodesData = nodesData;
}
public Map> getNodesInternalDomains() {
return nodesInternalDomains;
}
public void setNodesInternalDomains(Map> nodesInternalDomains) {
this.nodesInternalDomains = nodesInternalDomains;
}
@Override
public EnvironmentInfoResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(ENV)) {
this.env = new AppNodes()._fromJSON(json.getJSONObject(ENV));
}
if (json.has(NODES)) {
JSONArray nodesJson = json.getJSONArray(NODES);
nodes = new HashSet();
for (int i = 0; i < nodesJson.length(); i++) {
JSONObject stat = nodesJson.getJSONObject(i);
nodes.add(new SoftwareNode()._fromJSON(stat));
}
}
if (json.has(NODE_GROUPS)) {
JSONArray nodeGroupsArray = json.getJSONArray(NODE_GROUPS);
softNodeGroups = new HashSet();
for (int i = 0; i < nodeGroupsArray.length(); i++) {
softNodeGroups.add(new SoftNodeGroup()._fromJSON(nodeGroupsArray.getJSONObject(i)));
}
}
if (json.has(ENV_GROUPS)) {
JSONArray envGroupsArray = json.getJSONArray(ENV_GROUPS);
envGroups = new HashSet<>();
for (int i = 0; i < envGroupsArray.length(); i++) {
envGroups.add(envGroupsArray.getString(i));
}
}
if (json.has(MOUNTPOINTS)) {
JSONArray mountpointsArray = json.getJSONArray(MOUNTPOINTS);
mountpoints = new HashSet<>();
for (int i = 0; i < mountpointsArray.length(); i++) {
mountpoints.add(new MountPoint()._fromJSON(mountpointsArray.getJSONObject(i)));
}
}
if (json.has(TRIGGERS)) {
JSONArray triggersArray = json.getJSONArray(TRIGGERS);
triggers = new HashSet<>();
for (int i = 0; i < triggersArray.length(); i++) {
triggers.add(new Trigger()._fromJSON(triggersArray.getJSONObject(i)));
}
}
if (json.has(NODES_DATA)) {
JSONArray nodesDataArray = json.getJSONArray(NODES_DATA);
nodesData = new HashMap<>();
for (int i = 0; i < nodesDataArray.length(); i++) {
JSONObject nodeDataJsonObject = nodesDataArray.getJSONObject(i);
if (nodeDataJsonObject.has(NODE_ID) && nodeDataJsonObject.has(URL)) {
nodesData.put(nodeDataJsonObject.getInt(NODE_ID), nodeDataJsonObject.getString(URL));
}
}
}
if (json.has(NODES_INTERNAL_DOMAINS)) {
JSONArray nodesDomainsArray = json.getJSONArray(NODES_INTERNAL_DOMAINS);
nodesInternalDomains = new HashMap<>();
for (int i = 0; i < nodesDomainsArray.length(); i++) {
JSONObject nodeDomainsJsonObject = nodesDomainsArray.getJSONObject(i);
if (nodeDomainsJsonObject.has(NODE_ID) && nodeDomainsJsonObject.has(DOMAINS)) {
JSONArray nodeDomainsJsonArray = nodeDomainsJsonObject.getJSONArray(DOMAINS);
Set domains = new HashSet<>();
for (int j = 0; j < nodeDomainsJsonArray.length(); j++) {
domains.add(nodeDomainsJsonArray.getString(j));
}
nodesInternalDomains.put(nodeDomainsJsonObject.getInt(NODE_ID), domains);
}
}
}
return this;
}
@Override
public int compareTo(EnvironmentInfoResponse o) {
if (criteria == null) {
return 1;
}
String firstValue;
String secondValue;
switch(criteria.getOrderType()) {
case NAME:
firstValue = StringUtils.coalesce(env.getDisplayName(), env.getDomain().getDomain());
secondValue = StringUtils.coalesce(o.env.getDisplayName(), o.env.getDomain().getDomain());
break;
case STATUS:
firstValue = env.getStatus().name();
secondValue = o.env.getStatus().name();
break;
default:
return 1;
}
if (firstValue == null || secondValue == null) {
return 1;
}
switch(criteria.getOrder()) {
case ASC:
return firstValue.compareTo(secondValue);
case DESC:
return secondValue.compareTo(firstValue);
default:
return 1;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy