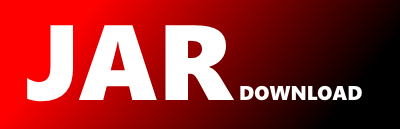
com.jelastic.api.environment.response.ExtDomainResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 9ebd73f6fd13056453bf5b91f8e76ad7*/
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.system.persistence.ExtDomain;
import com.jelastic.api.system.persistence.SSLCert;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Objects;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ExtDomainResponse extends Response {
private ExtDomain extDomain = new ExtDomain();
public ExtDomainResponse() {
super(OK);
}
public ExtDomainResponse(int result, String error) {
super(result, error);
}
public ExtDomainResponse(int result, String error, String extDomain) {
super(result, error);
this.extDomain = new ExtDomain(extDomain);
}
public ExtDomainResponse(Response response, ExtDomain extDomain) {
super(response);
this.extDomain = extDomain;
}
public ExtDomainResponse(ExtDomain extDomain) {
this();
this.extDomain = extDomain;
}
@Override
public JSONObject toJSON() {
try {
JSONObject json = super._toJSON();
if (extDomain == null) {
return json;
}
int id = extDomain.getId();
if (id > 0) {
json.put(ExtDomain.ID, id);
}
String domain = extDomain.getDomain();
if (StringUtils.isNotBlank(domain)) {
json.put(ExtDomain.DOMAIN, domain);
}
SSLCert sslCert = extDomain.getSslCert();
if (sslCert != null) {
json.put(ExtDomain.SSL_CERT, sslCert.getId());
}
json.put(ExtDomain.SSL_ENABLED, extDomain.isSslEnabled());
return json;
} catch (JSONException e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
@Override
public ExtDomainResponse fromJSON(JSONObject json) {
super.fromJSON(json);
extDomain.setId(json.optInt(ExtDomain.ID));
extDomain.setDomain(json.optString(ExtDomain.DOMAIN, null));
extDomain.setSslEnabled(json.optBoolean(ExtDomain.SSL_ENABLED));
return this;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
ExtDomainResponse that = (ExtDomainResponse) o;
return Objects.equals(extDomain, that.extDomain);
}
@Override
public int hashCode() {
return Objects.hash(extDomain);
}
public ExtDomain getExtDomain() {
return extDomain;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy