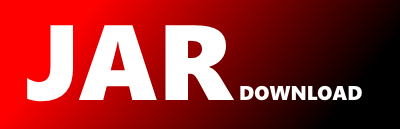
com.jelastic.api.environment.response.FilesListResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: d932b17142f4ded201abdc910e4c75a8*/
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.environment.po.RemouteFile;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class FilesListResponse extends Response {
public static final String KEYWORDS = "keywords";
public static final String KEYWORD = "keyword";
public static final String PATH = "path";
public static final String PERMISSION = "permission";
public static final String LAST_MODIFIED = "lastModified";
public static final String IS_DIR = "isdir";
public static final String IS_EXPORTED = "isExported";
public static final String NAME = "name";
public static final String SIZE = "size";
public static final String SOURCE_PATH = "sourcePath";
public static final String SOURCE_HOST = "sourceHost";
public static final String PROTOCOL = "protocol";
public static final String SOURCE_NODE_ID = "sourceNodeId";
public static final String FILES = "files";
public static final String FILE_TYPE = "fileType";
private Map> keywords;
private String coreRootPath;
private HashSet directoryPathList;
public FilesListResponse(Map> filesMap) {
super(Response.OK);
this.keywords = filesMap;
}
public FilesListResponse(Map> filesMap, String rootPath) {
super(Response.OK);
this.keywords = filesMap;
this.coreRootPath = rootPath;
}
public FilesListResponse(Map> filesMap, String rootPath, HashSet directoryPathList) {
super(Response.OK);
this.keywords = filesMap;
this.coreRootPath = rootPath;
this.directoryPathList = directoryPathList;
}
public FilesListResponse(int result, String error) {
super(result, error);
}
public FilesListResponse() {
super(Response.OK);
}
public Map> getFilesMap() {
return keywords;
}
public void setFilesMap(Map> filesMap) {
this.keywords = filesMap;
}
@Override
public FilesListResponse fromJSON(JSONObject json) {
Response response = super.fromJSON(json);
try {
if (json.has(KEYWORDS)) {
this.keywords = new HashMap>();
JSONArray keywords = json.getJSONArray(KEYWORDS);
for (int i = 0; i < keywords.length(); i++) {
JSONObject keywordObject = keywords.getJSONObject(i);
String keyword = "";
if (keywordObject.has(KEYWORD)) {
keyword = keywordObject.getString(KEYWORD);
}
String path = "";
if (keywordObject.has(PATH)) {
path = keywordObject.getString(PATH);
}
String permissions = "";
if (keywordObject.has(PERMISSION)) {
permissions = keywordObject.getString(PERMISSION);
}
String lastModified = "";
if (keywordObject.has(LAST_MODIFIED)) {
lastModified = keywordObject.getString(LAST_MODIFIED);
}
Integer sourceNodeId = null;
if (keywordObject.has(SOURCE_NODE_ID)) {
sourceNodeId = keywordObject.getInt(SOURCE_NODE_ID);
}
String sourceHost = null;
if (keywordObject.has(SOURCE_HOST)) {
sourceHost = keywordObject.getString(SOURCE_HOST);
}
Boolean isExported = null;
if (keywordObject.has(IS_EXPORTED)) {
isExported = keywordObject.getBoolean(IS_EXPORTED);
}
String protocol = null;
if (keywordObject.has(PROTOCOL)) {
protocol = keywordObject.getString(PROTOCOL);
}
List remoteFiles = new ArrayList();
if (keywordObject.has(FILES)) {
JSONArray filesArray = keywordObject.getJSONArray(FILES);
for (int j = 0; j < filesArray.length(); j++) {
JSONObject fileJson = filesArray.getJSONObject(j);
RemouteFile file = new RemouteFile();
if (fileJson.has(IS_DIR)) {
file.setIsDir(fileJson.getBoolean(IS_DIR));
}
if (fileJson.has(IS_EXPORTED)) {
file.setExported(fileJson.getBoolean(IS_EXPORTED));
}
if (fileJson.has(PATH)) {
file.setPath(fileJson.getString(PATH));
}
if (fileJson.has(NAME)) {
file.setName(fileJson.getString(NAME));
}
if (fileJson.has(SIZE)) {
file.setLength(fileJson.getLong(SIZE));
}
if (fileJson.has(PERMISSION)) {
file.setPermission(fileJson.getString(PERMISSION));
}
if (fileJson.has(LAST_MODIFIED)) {
file.setLastModified(fileJson.getString(LAST_MODIFIED));
}
if (fileJson.has(FILE_TYPE)) {
file.setFileType(fileJson.getString(FILE_TYPE));
}
remoteFiles.add(file);
}
}
if (isExported != null) {
remoteFiles.add(new RemouteFile(path, keyword, permissions, true, 0L, lastModified, sourceHost, protocol, sourceNodeId, isExported));
} else {
remoteFiles.add(new RemouteFile(path, keyword, permissions, true, 0L, lastModified, sourceHost, protocol, sourceNodeId));
}
this.keywords.put(keyword, remoteFiles);
}
}
} catch (JSONException ex) {
response = new Response(ERROR_UNKNOWN, ex.toString());
}
setResult(response.getResult());
setError(response.getError());
return this;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
JSONArray keywords = new JSONArray();
try {
if (this.keywords != null) {
for (String keyword : this.keywords.keySet()) {
JSONArray filesArray = new JSONArray();
String rootPath = "";
String permission = "";
String sourcePath = null;
String sourceHost = null;
String protocol = null;
Integer sourceNodeId = 0;
Boolean isExported = null;
List sortedFiles = new ArrayList(this.keywords.get(keyword));
for (RemouteFile file : sortedFiles) {
if (coreRootPath == null) {
if (keyword.equals(file.getName()) && file.isRoot()) {
rootPath = file.getPath();
sourcePath = file.getSourcePath();
sourceHost = file.getSourceHost();
protocol = file.getProtocol();
sourceNodeId = file.getSourceNodeId();
permission = file.getPermission();
isExported = file.isExported();
if (file.isDirectory()) {
continue;
}
}
} else {
if (file.getPath().equals(coreRootPath)) {
rootPath = file.getPath();
permission = file.getPermission();
sourcePath = file.getSourcePath();
sourceHost = file.getSourceHost();
protocol = file.getProtocol();
sourceNodeId = file.getSourceNodeId();
isExported = file.isExported();
if (file.isDirectory()) {
continue;
}
}
}
JSONObject fileJson = new JSONObject();
fileJson.put(IS_DIR, file.isDirectory());
fileJson.put(IS_EXPORTED, file.isExported());
fileJson.put(PATH, file.getPath());
fileJson.put(NAME, file.getName());
fileJson.put(PERMISSION, file.getPermission());
fileJson.put(LAST_MODIFIED, file.getLastModified());
fileJson.put(SOURCE_PATH, file.getSourcePath());
fileJson.put(SOURCE_HOST, file.getSourceHost());
fileJson.put(PROTOCOL, file.getProtocol());
fileJson.put(SOURCE_NODE_ID, file.getSourceNodeId());
fileJson.put(FILE_TYPE, file.getFileType());
if (!file.isDirectory()) {
fileJson.put(SIZE, file.length());
}
filesArray.put(fileJson);
}
JSONObject keywordJson = new JSONObject();
keywordJson.put(KEYWORD, keyword);
keywordJson.put(PATH, rootPath);
keywordJson.put(PERMISSION, permission);
keywordJson.put(SOURCE_PATH, sourcePath);
keywordJson.put(SOURCE_HOST, sourceHost);
keywordJson.put(PROTOCOL, protocol);
keywordJson.put(SOURCE_NODE_ID, sourceNodeId);
keywordJson.put(IS_EXPORTED, isExported);
keywordJson.put(FILES, filesArray);
if (directoryPathList != null && !directoryPathList.isEmpty()) {
for (String directoryPathItem : directoryPathList) {
Path directoryPath = Paths.get(directoryPathItem);
Path directoryRootPath = Paths.get(coreRootPath);
if (!directoryPath.equals(directoryRootPath)) {
String directoryName = directoryPath.getName(directoryPath.getNameCount() - 1).toString();
if (directoryName.equals(keyword)) {
keywordJson.put("isDir", true).put(PATH, directoryPath.toString());
}
}
directoryPath.getName(directoryPath.getNameCount() - 1);
}
}
keywords.put(keywordJson);
}
json.put(KEYWORDS, keywords);
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy