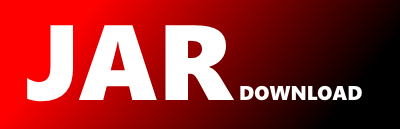
com.jelastic.api.environment.response.LogsListResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 4fcc66c27aed9833d002f89d56ca3657*/
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.environment.po.RemouteFile;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class LogsListResponse extends Response {
private static final String ARRAY = "array";
private static final String PATH = "path";
private static final String PERMISSION = "permission";
private static final String NAME = "name";
private static final String SIZE = "size";
private static final String ITEMS = "items";
private static final String TYPE = "type";
private Map> directories;
private Map> groups;
private Map> rotatedLogs;
private String path;
public LogsListResponse(Map> directories, Map> groups, Map> rotatedLogs, String path) {
super(Response.OK);
this.directories = directories;
this.groups = groups;
this.rotatedLogs = rotatedLogs;
this.path = path;
}
public LogsListResponse(int result, String error) {
super(result, error);
}
public LogsListResponse() {
super(Response.OK);
}
public Map> getDirectories() {
return directories;
}
public Map> getGroups() {
return groups;
}
public Map> getRotatedLogs() {
return rotatedLogs;
}
public void setDirectories(Map> directories) {
this.directories = directories;
}
public void setGroups(Map> groups) {
this.groups = groups;
}
public void setRotatedLogs(Map> rotatedLogs) {
this.rotatedLogs = rotatedLogs;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
@Override
public LogsListResponse fromJSON(JSONObject json) {
Response response = super.fromJSON(json);
try {
if (json.has(ARRAY)) {
this.directories = new HashMap<>();
this.groups = new HashMap<>();
this.rotatedLogs = new HashMap<>();
JSONArray itemArray = json.getJSONArray(ARRAY);
for (int i = 0; i < itemArray.length(); i++) {
JSONObject itemObject = itemArray.getJSONObject(i);
String name = "";
if (itemObject.has(NAME)) {
name = itemObject.getString(NAME);
}
String path = "";
if (itemObject.has(PATH)) {
path = itemObject.getString(PATH);
}
String permissions = "";
if (itemObject.has(PERMISSION)) {
permissions = itemObject.getString(PERMISSION);
}
ItemType itemType = ItemType.FILE;
if (itemObject.has(TYPE)) {
itemType = ItemType.valueOf(itemObject.getString(TYPE));
}
List remoteFiles = new ArrayList<>();
if (itemObject.has(ITEMS)) {
JSONArray itemsArray = itemObject.getJSONArray(ITEMS);
for (int j = 0; j < itemsArray.length(); j++) {
JSONObject itemJSON = itemsArray.getJSONObject(j);
RemouteFile file = new RemouteFile();
if (itemJSON.has(TYPE)) {
file.setIsDir(itemJSON.getString(TYPE).equalsIgnoreCase(ItemType.DIRECTORY.toString()));
}
if (itemJSON.has(PATH)) {
file.setPath(itemJSON.getString(PATH));
}
if (itemJSON.has(NAME)) {
file.setName(itemJSON.getString(NAME));
}
if (itemJSON.has(SIZE)) {
file.setLength(itemJSON.getLong(SIZE));
}
if (itemJSON.has(PERMISSION)) {
file.setPermission(itemJSON.getString(PERMISSION));
}
remoteFiles.add(file);
}
}
remoteFiles.add(new RemouteFile(path, name, permissions, true, 0l));
if (itemType == ItemType.DIRECTORY) {
directories.put(name, remoteFiles);
} else if (itemType == ItemType.GROUP) {
groups.put(name, remoteFiles);
} else if (itemType == ItemType.ROTATED_LOGS) {
rotatedLogs.put(name, remoteFiles);
}
}
}
} catch (JSONException ex) {
response = new Response(ERROR_UNKNOWN, ex.toString());
}
setResult(response.getResult());
setError(response.getError());
return this;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
JSONArray items = new JSONArray();
try {
addItems(items, directories, ItemType.DIRECTORY);
addItems(items, groups, ItemType.GROUP);
addItems(items, rotatedLogs, ItemType.ROTATED_LOGS);
json.put(ARRAY, items);
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
private void addItems(JSONArray items, Map> itemsMap, ItemType itemType) throws JSONException {
if (itemsMap != null) {
for (String name : itemsMap.keySet()) {
JSONArray itemArray = new JSONArray();
String permission = "";
List sortedFiles = new ArrayList<>(itemsMap.get(name));
for (RemouteFile file : sortedFiles) {
if (itemType == ItemType.DIRECTORY && file.getPath().equals(this.path)) {
permission = file.getPermission();
if (file.isDirectory()) {
continue;
}
}
JSONObject itemJson = new JSONObject();
itemJson.put(TYPE, file.isDirectory() ? ItemType.DIRECTORY : ItemType.FILE);
itemJson.put(PATH, file.getPath());
itemJson.put(NAME, file.getName());
itemJson.put(PERMISSION, file.getPermission());
if (!file.isDirectory()) {
itemJson.put(SIZE, file.length());
}
itemArray.put(itemJson);
}
JSONObject itemJson = new JSONObject();
itemJson.put(NAME, name);
itemJson.put(ITEMS, itemArray);
itemJson.put(TYPE, itemType);
if (itemType == ItemType.DIRECTORY) {
itemJson.put(PATH, this.path);
itemJson.put(PERMISSION, permission);
}
items.put(itemJson);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy