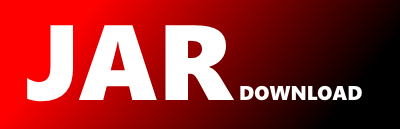
com.jelastic.api.environment.response.RepoResponse Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.DateUtils;
import org.json.JSONException;
import org.json.JSONObject;
import java.text.ParseException;
import java.util.Date;
/**
* @author Maksym Shevchuk
*/
public class RepoResponse extends Response {
public static final String ID = "id";
public static final String NAME = "name";
public static final String TYPE = "type";
public static final String BRANCH = "branch";
public static final String URL = "url";
public static final String LOGIN = "login";
public static final String KEY_ID = "keyId";
public static final String DESCRIPTION = "description";
public static final String CREATED_ON = "createdOn";
private int id;
private String type;
private String name;
private String url;
private String branch;
private Integer keyId;
private String login;
private String description;
private Date createdOn;
public RepoResponse() {
super(Response.OK());
}
public RepoResponse(int result, String error) {
super(result, error);
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getBranch() {
return branch;
}
public void setBranch(String branch) {
this.branch = branch;
}
public Integer getKeyId() {
return keyId;
}
public void setKeyId(Integer keyId) {
this.keyId = keyId;
}
public String getLogin() {
return login;
}
public void setLogin(String login) {
this.login = login;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(TYPE, type);
json.put(NAME, name);
json.put(URL, url);
json.put(BRANCH, branch);
json.put(KEY_ID, keyId);
json.put(DESCRIPTION, description);
if (createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(createdOn));
}
if (login != null) {
json.put(LOGIN, login);
}
return json;
}
@Override
public RepoResponse _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(URL)) {
this.url = json.getString(URL);
}
if (json.has(BRANCH)) {
this.branch = json.getString(BRANCH);
}
if (json.has(KEY_ID)) {
this.keyId = json.getInt(KEY_ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(TYPE) && !json.isNull(TYPE)) {
this.type = json.getString(TYPE).toUpperCase();
}
if (json.has(LOGIN)) {
this.login = json.getString(LOGIN);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
if (json.has(CREATED_ON)) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString(CREATED_ON));
} catch (ParseException e) {
}
}
if (createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(createdOn));
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy