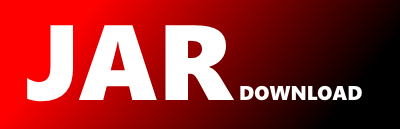
com.jelastic.api.environment.response.StringArrayResponse Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.environment.response;
import com.jelastic.api.Response;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Collection;
import java.util.HashSet;
/**
*
* @author Alexey Shponarsky
*/
public class StringArrayResponse extends Response {
private Collection array;
public static final String ARRAY_JSON = "array";
public StringArrayResponse() {
super(OK);
this.array = new HashSet();
}
public StringArrayResponse(int result, String error) {
super(result, error);
}
public StringArrayResponse(Collection array) {
super(OK);
this.array = array;
}
public Collection getArray() {
return array;
}
public void setArray(Collection array) {
this.array = array;
}
@Override
protected StringArrayResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
array = new HashSet();
if (json.has(ARRAY_JSON)) {
JSONArray arrayJson = json.getJSONArray(ARRAY_JSON);
for (int i = 0; i < arrayJson.length(); i++) {
array.add(arrayJson.getString(i));
}
}
return this;
}
@Override
protected JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (array != null) {
JSONArray arrayJson = new JSONArray();
for (String item : array) {
arrayJson.put(item);
}
json.put(ARRAY_JSON, arrayJson);
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy