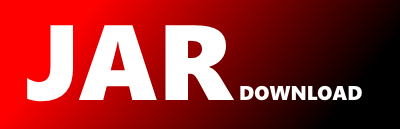
com.jelastic.api.marketplace.response.InstallResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 0063a746f7cff753261a0fa6ddfc30bf*/
package com.jelastic.api.marketplace.response;
import com.jelastic.api.response.ScriptEvalProxyResponse;
import org.json.JSONException;
import org.json.JSONObject;
import java.lang.String;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class InstallResponse extends ScriptEvalProxyResponse {
private String reason = null;
private String uniqueName = null;
private String appid = null;
private String successText = null;
private String type = null;
private String message = null;
private String startPage = null;
private String action = null;
private JSONObject data = null;
private Object response = null;
public InstallResponse() {
}
public InstallResponse(int result, String error) {
super(result, error);
}
public InstallResponse(String uniqueName, String appid, String successText) {
super();
this.uniqueName = uniqueName;
this.appid = appid;
this.successText = successText;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
public String getUniqueName() {
return uniqueName;
}
public void setUniqueName(String uniqueName) {
this.uniqueName = uniqueName;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public String getStartPage() {
return startPage;
}
public void setStartPage(String startPage) {
this.startPage = startPage;
}
public String getAction() {
return action;
}
public void setAction(String action) {
this.action = action;
}
public JSONObject getData() {
return data;
}
public void setData(JSONObject data) {
this.data = data;
}
@Override
public JSONObject _toJSON(JSONObject responseJson) throws JSONException {
JSONObject json = super._toJSON();
if (reason != null) {
json.put("reason", reason);
}
if (uniqueName != null) {
json.put("uniqueName", uniqueName);
}
if (appid != null) {
json.put("appid", appid);
}
if (successText != null) {
json.put("successText", successText);
}
if (type != null) {
json.put("type", type);
}
if (message != null) {
json.put("message", message);
}
if (startPage != null) {
json.put("startPage", startPage);
}
if (action != null) {
json.put("action", action);
}
if (data != null) {
json.put("data", data);
}
if (!JSONObject.NULL.equals(responseJson)) {
json.put("response", responseJson);
}
return json;
}
@Override
public InstallResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (!(getResponse() instanceof JSONObject)) {
return this;
}
JSONObject responseJson = (JSONObject) getResponse();
if (responseJson.has("reason")) {
this.reason = String.valueOf(responseJson.get("reason"));
}
if (responseJson.has("uniqueName")) {
this.uniqueName = responseJson.getString("uniqueName");
}
if (responseJson.has("appid")) {
this.appid = responseJson.getString("appid");
}
if (responseJson.has("successText")) {
this.successText = responseJson.getString("successText");
}
if (responseJson.has("type")) {
this.type = responseJson.getString("type");
}
if (responseJson.has("message")) {
this.message = responseJson.getString("message");
}
if (responseJson.has("startPage")) {
this.startPage = responseJson.getString("startPage");
}
if (responseJson.has("action")) {
this.action = responseJson.getString("action");
}
if (responseJson.has("data")) {
this.data = responseJson.getJSONObject("data");
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy