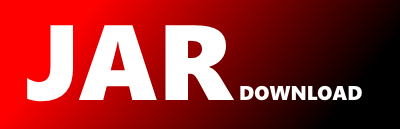
com.jelastic.api.response.StubResponse Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.response;
import com.google.gson.*;
import java.lang.reflect.Modifier;
import java.lang.reflect.Type;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.jelastic.api.Response;
/**
* @author Dima Hutsalo
*/
public class StubResponse extends Response {
private static final String SCRIPT_RESPONSE_KEY = "response";
private static GsonBuilder gsonBuilder = new GsonBuilder();
private static Gson GSON = null;
static {
gsonBuilder.excludeFieldsWithModifiers(Modifier.STATIC);
gsonBuilder.enableComplexMapKeySerialization();
gsonBuilder.registerTypeAdapter(JSONObject.class, new JsonDeserializer() {
@Override
public JSONObject deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) throws JsonParseException {
try {
return new JSONObject(json.toString());
} catch (JSONException e) {
System.out.println(json);
return new JSONObject();
}
}
});
gsonBuilder.registerTypeAdapter(JSONArray.class, new JsonDeserializer() {
@Override
public JSONArray deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) throws JsonParseException {
try {
return new JSONArray(json.toString());
} catch (JSONException e) {
System.out.println(json);
return new JSONArray();
}
}
});
GSON = gsonBuilder.create();
}
@Override
protected JSONObject _toJSON() throws JSONException {
String jsonStr = GSON.toJson(this);
JSONObject json = new JSONObject(jsonStr);
if (getResult() == -1) {
json.remove("result");
}
if (getReason() == -1) {
json.remove("reason");
}
if (isOK()) {
json.remove("source");
}
return json;
}
@Override
protected StubResponse _fromJSON(JSONObject json) throws JSONException {
if (json.has(SCRIPT_RESPONSE_KEY)) {
Object responseObj = json.get(SCRIPT_RESPONSE_KEY);
if (responseObj instanceof JSONObject) {
json = (JSONObject) responseObj;
}
}
super._fromJSON(json);
return this;
}
}