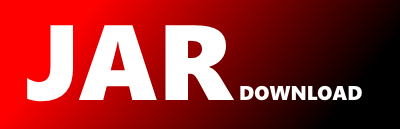
com.jelastic.api.ssh.SSHResponse Maven / Gradle / Ivy
/*Server class MD5: 7b908740f03c62ff71fc7f42e6d1cec2*/
package com.jelastic.api.ssh;
import com.jelastic.api.Response;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SSHResponse extends Response {
private static final String OUT = "out";
private static final String ERROR = "error";
private static final String EXIT_STATUS = "exitStatus";
private static final String ERR_OUT = "errOut";
private static final String ERROR_SEPARATOR = ". ";
private String out;
private String errOut;
private int exitStatus = -1;
public SSHResponse() {
super(Response.OK);
}
public SSHResponse(int result, String error) {
super(result, error);
}
public String getOut() {
return out;
}
public void setOut(String out) {
this.out = out;
}
public int getExitStatus() {
return exitStatus;
}
public void setExitStatus(int exitStatus) {
this.exitStatus = exitStatus;
}
public String getErrOut() {
return errOut;
}
public void setErrOut(String errOut) {
if (isNotOK()) {
this.error += errOut != null ? ERROR_SEPARATOR + errOut : "";
}
this.errOut = errOut;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
if (out != null) {
json.put(OUT, out);
}
if (error != null) {
json.put(ERROR, error);
}
if (exitStatus > -1) {
json.put(EXIT_STATUS, exitStatus);
}
json.put(ERR_OUT, errOut);
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
@Override
public SSHResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(OUT)) {
out = json.getString(OUT);
}
if (json.has(ERROR)) {
error = json.getString(ERROR);
}
if (json.has(EXIT_STATUS)) {
try {
exitStatus = json.getInt(EXIT_STATUS);
} catch (JSONException ex) {
exitStatus = -1;
}
}
if (json.has(ERR_OUT)) {
errOut = json.getString(ERR_OUT);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy