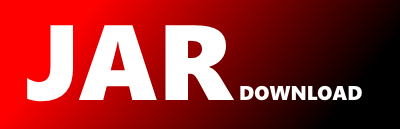
com.jelastic.api.statistic.response.EnvironmentStatisticResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 480e8535cb28ed36554a8e3d7dc7a0f9*/
package com.jelastic.api.statistic.response;
import com.jelastic.api.Response;
import com.jelastic.api.system.statistic.persistence.StatElement;
import java.util.ArrayList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.jelastic.api.system.persistence.AppNodes;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class EnvironmentStatisticResponse extends Response {
private int status = -1;
private String appid = null;
private Boolean isTransferring = null;
private List stats;
public EnvironmentStatisticResponse(List stats, AppNodes appNodes) {
super(OK);
this.stats = stats;
this.status = appNodes.getStatus().getValue();
this.appid = appNodes.getAppid();
this.isTransferring = appNodes.isTransferring();
}
public EnvironmentStatisticResponse(List stats, int status) {
super(OK);
this.stats = stats;
this.status = status;
}
public EnvironmentStatisticResponse(List stats, int status, String appid) {
super(OK);
this.stats = stats;
this.status = status;
this.appid = appid;
}
public EnvironmentStatisticResponse(List stats) {
super(OK);
this.stats = stats;
}
public EnvironmentStatisticResponse(StatElement stat) {
this();
this.stats.add(stat);
}
public EnvironmentStatisticResponse(StatElement stat, int status) {
this();
this.stats.add(stat);
this.status = status;
}
public EnvironmentStatisticResponse() {
super(OK);
this.stats = new ArrayList();
}
public EnvironmentStatisticResponse(int result, String error) {
super(result, error);
}
public int getStatus() {
return status;
}
public List getStats() {
return stats;
}
public void setStatus(int status) {
this.status = status;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (stats != null) {
JSONArray statsJson = new JSONArray();
for (StatElement statElement : stats) {
statsJson.put(statElement._toJSON());
}
json.put("stats", statsJson);
}
if (this.status != -1) {
json.put("status", status);
}
json.put("appid", appid);
json.put("isTransferring", isTransferring);
return json;
}
@Override
public EnvironmentStatisticResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
stats = new ArrayList();
if (json.has("stats")) {
JSONArray statsJson = json.getJSONArray("stats");
for (int i = 0; i < statsJson.length(); i++) {
JSONObject stat = statsJson.getJSONObject(i);
stats.add(new StatElement()._fromJSON(stat));
}
}
if (json.has("status")) {
this.status = json.getInt("status");
}
if (json.has("appid")) {
this.appid = json.getString("appid");
}
if (json.has("isTransferring")) {
this.isTransferring = json.getBoolean("isTransferring");
}
return this;
}
public EnvironmentStatisticResponse fromJSON(JSONObject json) {
try {
return _fromJSON(json);
} catch (JSONException ex) {
error = ex.toString();
result = ERROR_UNKNOWN;
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy