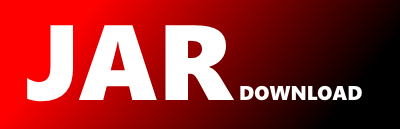
com.jelastic.api.system.persistence.Account Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 1513e846dddf014ecb8c3d027b186085*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.extern.exception.NullEbsUserIdException;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.core.utils.DateUtils;
import java.math.BigDecimal;
import java.text.ParseException;
import org.json.*;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Account extends ArrayItem {
public static final String ACCOUNT_COLUMN = "account";
public static final String TEST_LABEL_NAME = "test";
public static final String UID = "uid";
public static final String BALANCE = "balance";
public static final String BONUS = "bonus";
public static final String STATUS = "status";
public static final String CREATED_ON = "createdOn";
public static final String UPDATED_ON = "updatedOn";
public static final String QUOTAS_VALUES = "quotasValues";
public static final String ACCOUNT_DATA = "accountData";
public static final String UPDATED_STATUS_ON = "updatedStatusOn";
public static final String UPDATED_GROUP_ON = "updatedGroupOn";
public static final String PREVIOUS_UPDATED_GROUP_ON = "previousUpdatedGroupOn";
public static final String EBS_USER_ID = "ebsUserId";
public static final String AUTO_PAY_TYPE = "autoPayType";
public static final String DEFAULT_PAYMETHOD_ID = "defaultPayMethodId";
public static final String NOTE = "note";
public static final String GROUP = "group";
private int uid;
private BigDecimal balance;
private BigDecimal bonus;
private int status;
private Date createdOn;
private Date updatedOn;
private BigDecimal debit;
private BigDecimal credit;
private Group group;
private Set quotasValues;
private Set accountData;
private Date updatedStatusOn;
private Date updatedGroupOn;
private Date previousUpdatedGroupOn;
String email;
private Boolean isEnabled;
private String language;
private String ebsUserId;
private AutoPayType autoPayType;
private String defaultPayMethodId;
private String note;
private BigDecimal sumCostPerHour;
public Account() {
}
public Account(int id) {
this.id = id;
}
public Account(int uid, BigDecimal balance, BigDecimal cost) {
this.uid = uid;
this.balance = balance;
this.sumCostPerHour = cost;
}
public Set getAccountData() {
return accountData;
}
public void setAccountData(Set accountData) {
this.accountData = accountData;
}
public Integer getDefaultPayMethodId() {
return defaultPayMethodId != null ? Integer.parseInt(defaultPayMethodId) : null;
}
public String getDefaultPayMethodIdStr() {
return defaultPayMethodId;
}
public void setDefaultPayMethodId(Integer defaultPayMethodId) {
this.defaultPayMethodId = defaultPayMethodId.toString();
}
public void setDefaultPayMethodId(String defaultPayMethodId) {
this.defaultPayMethodId = defaultPayMethodId;
}
public AutoPayType getAutoPayType() {
return autoPayType;
}
public void setAutoPayType(AutoPayType payType) {
this.autoPayType = payType;
}
public Long getEbsUserId() throws NullEbsUserIdException {
if (ebsUserId == null || ebsUserId.length() == 0) {
throw new NullEbsUserIdException();
}
return Long.valueOf(ebsUserId);
}
public Long getEbsUserIdNullable() {
if (ebsUserId != null) {
try {
return Long.valueOf(ebsUserId);
} catch (Exception ignore) {
ignore.printStackTrace();
}
}
return null;
}
public void setEbsUserId(Long ebsUserId) {
this.ebsUserId = String.valueOf(ebsUserId);
}
public void setEbsUserIdAsString(String ebsUserId) {
this.ebsUserId = ebsUserId;
}
public String getEbsUserIdAsString() throws NullEbsUserIdException {
if (ebsUserId == null || ebsUserId.length() == 0) {
throw new NullEbsUserIdException();
}
return ebsUserId;
}
public String getEbsUserIdAsStringNullable() {
return ebsUserId;
}
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public BigDecimal getBonus() {
return bonus;
}
public void setBonus(BigDecimal bonus) {
this.bonus = bonus;
}
public AccountStatus getStatus() {
return AccountStatus.parse(status);
}
public void setStatus(AccountStatus status) {
this.status = status.getValue();
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public BigDecimal getCredit() {
return credit;
}
public void setCredit(BigDecimal credit) {
this.credit = credit;
}
public BigDecimal getDebit() {
return debit;
}
public void setDebit(BigDecimal debit) {
this.debit = debit;
}
public Group getGroup() {
return group;
}
public void setGroup(Group group) {
this.group = group;
}
public Set getQuotasValues() {
return quotasValues;
}
public void setQuotasValues(Set quotasValues) {
this.quotasValues = quotasValues;
}
public Date getUpdatedStatusOn() {
return updatedStatusOn;
}
public void setUpdatedStatusOn(Date updatedStatusOn) {
this.updatedStatusOn = updatedStatusOn;
}
public Date getUpdatedGroupOn() {
return updatedGroupOn;
}
public void setUpdatedGroupOn(Date updatedGroupOn) {
this.updatedGroupOn = updatedGroupOn;
}
public Date getPreviousUpdatedGroupOn() {
return previousUpdatedGroupOn;
}
public void setPreviousUpdatedGroupOn(Date previousUpdatedGroupOn) {
this.previousUpdatedGroupOn = previousUpdatedGroupOn;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Boolean getEnabled() {
return isEnabled;
}
public void setEnabled(Boolean enabled) {
isEnabled = enabled;
}
public Boolean isRegistered() {
return ebsUserId != null || group.getType().isCommercial();
}
public Reseller getReseller() {
return group.getReseller();
}
public String getNote() {
return note;
}
public void setNote(String note) {
this.note = note;
}
public BigDecimal getSumCostPerHour() {
return sumCostPerHour;
}
public void setSumCostPerHour(BigDecimal sumCostPerHour) {
this.sumCostPerHour = sumCostPerHour;
}
@Override
public Account _fromJSON(JSONObject json) throws JSONException {
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has("uid")) {
this.uid = json.getInt("uid");
}
if (json.has("balance")) {
this.balance = new BigDecimal(json.getString("balance"));
}
if (json.has("bonus")) {
this.bonus = new BigDecimal(json.getString("bonus"));
}
if (json.has("debit")) {
this.debit = new BigDecimal(json.getString("debit"));
}
if (json.has("status")) {
this.status = json.getInt("status");
}
if (json.has("createdOn")) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString("createdOn"));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has("group")) {
this.group = new Group()._fromJSON(json.getJSONObject("group"));
}
if (json.has("updatedOn")) {
try {
this.updatedOn = DateUtils.parseSqlDateTime(json.getString("updatedOn"));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has("quotasValues")) {
this.quotasValues = new HashSet();
JSONArray array = json.getJSONArray("quotasValues");
for (int i = 0; i < array.length(); i++) {
quotasValues.add((QuotaValue) new QuotaValue()._fromJSON(array.getJSONObject(i)));
}
}
if (json.has("updatedStatusOn")) {
try {
this.updatedStatusOn = DateUtils.parseSqlDateTime(json.getString("updatedStatusOn"));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has("updatedGroupOn")) {
try {
this.updatedGroupOn = DateUtils.parseSqlDateTime(json.getString("updatedGroupOn"));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has("email")) {
this.email = json.getString("email");
}
if (json.has("note")) {
this.note = json.getString("note");
}
if (json.has("isEnabled")) {
this.isEnabled = json.getInt("isEnabled") == 1;
}
return this;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("id", id);
json.put("uid", uid);
json.put("balance", balance);
json.put("bonus", bonus);
json.put("debit", debit);
json.put("status", status);
if (createdOn != null) {
json.put("createdOn", DateUtils.formatSqlDateTime(createdOn));
}
if (updatedOn != null) {
json.put("updatedOn", DateUtils.formatSqlDateTime(updatedOn));
}
if (group != null) {
json.put("group", group._toJSON());
}
if (quotasValues != null) {
JSONArray array = new JSONArray();
for (QuotaValue quotaValue : quotasValues) {
array.put(quotaValue._toJSON());
}
json.put("quotasValues", array);
}
if (updatedStatusOn != null) {
json.put("updatedStatusOn", DateUtils.formatSqlDateTime(updatedStatusOn));
}
if (updatedGroupOn != null) {
json.put("updatedGroupOn", DateUtils.formatSqlDateTime(updatedGroupOn));
}
if (note != null) {
json.put("note", note);
}
json.put("email", email);
if (isEnabled != null) {
json.put("isEnabled", isEnabled ? 1 : 0);
}
return json;
}
public long getEndOfTrialPeriod(long trialDays) {
return updatedGroupOn.getTime() + DateUtils.ONE_DAY_MSEC * trialDays;
}
public double getTotalBalance() {
return getBalance().doubleValue() + getBonus().doubleValue();
}
public String getLanguage() {
return language;
}
public void setLanguage(String language) {
this.language = language;
}
public boolean isPersonalQuotaExists(String quotaName) {
for (QuotaValue quotaValue : this.getQuotasValues()) {
if (quotaValue.getQuota().getName().equals(quotaName)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy