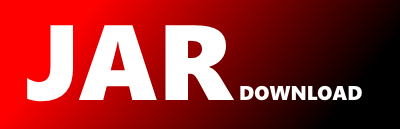
com.jelastic.api.system.persistence.AppNodes Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 6c321697bf6b609474d2681ccf1474ab*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.common.Constants;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.core.utils.JSONUtils;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import com.jelastic.api.system.service.utils.EnvironmentStatus;
import com.jelastic.api.system.service.utils.NodeUtils;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.text.ParseException;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class AppNodes extends ArrayItem implements Serializable {
public static final String ALIASES = "aliases";
public static final String APPID = "appid";
public static final String HARDWARE_NODE_GROUP = "hardwareNodeGroup";
public static final String EXT_DOMAINS = "extDomains";
public static final String UID = "uid";
public static final String OWNER_UID = "ownerUid";
public static final String HOST_GROUP = "hostGroup";
public static final String HOST_GROUP_UNIQUE_NAME = "uniqueName";
public static final String HOST_GROUP_DISPLAY_NAME = "displayName";
public static final String ENV_COLUMN = "env";
public static final String DOMAIN = "domain";
public static final String REGION = "region";
public static final String STATUS = "status";
public static final String SSLSTATE = "sslstate";
public static final String SHORTDOMAIN = "shortdomain";
public static final String ENV_NAME = "envName";
public static final String CREATOR_UID = "creatorUid";
public static final String APPNAME = "appname";
public static final String ENGINE = "engine";
public static final String WINDOMAIN = "windomain";
public static final String CONTEXTS = "contexts";
public static final String CUSTOM_SSL = "customSSL";
public static final String EXTDOMAINS = "extdomains";
public static final String IS_HA_ENABLED = "ishaenabled";
public static final String IS_FIREWALL_ENABLED = "isFirewallEnabled";
public static final String CREATED_ON = "createdOn";
public static final String NOTE = "note";
public static final String DESCRIPTION = "description";
public static final String IS_BROKEN = "isBroken";
public static final String IS_TRANSFERRING = "isTransferring";
public static final String DISPLAY_NAME = "displayName";
public static final String IS_SHARED = "isShared";
public static final String ENV_GROUPS = "groups";
public static final String IS_REMOTE = "isRemote";
public static final String CREDENTIAL_LOGIN = "login";
public static final String FEATURE_NAME = "name";
public static final String IS_LOCKED = "isLocked";
public static final String ATTRIBUTES = "attributes";
public static final String PROPERTIES = "properties";
public static final String PROJECT = "project";
private String appid;
private int uid;
private int creatorUid;
private Domain domain;
private Set domains = new HashSet<>();
private Set nodes = new HashSet<>();
private Set contexts = new HashSet<>();
private Set extDomains = new HashSet<>();
private SSLCustom customSSL;
private int status = EnvironmentStatus.ENV_STATUS_TYPE_RUNNING.getValue();
private EnvironmentStatus originalStatus;
private String appname;
private Engine engine;
private boolean sslState;
private boolean isRemote = false;
private Date createdOn;
private Date updatedOn;
private boolean isDeleted;
private boolean isHAEnabled;
private boolean isFirewallEnabled;
private boolean isProtected;
private int isUpToDate;
private Boolean isBroken;
private String fullDomain;
private String zone;
private boolean isTransferring;
private String displayName;
private String note;
private String description;
private HardwareNodeGroup hardwareNodeGroup;
private WinDomain winDomain;
private Boolean isShared;
private Set groups = new HashSet<>();
private Ipv6Subnet subnet;
private String attributes;
private JSONObject attributesJson;
private String properties;
private Project project;
public AppNodes() {
}
public AppNodes(String appid) {
this.appid = appid;
}
public AppNodes(int id, String appid) {
this.id = id;
this.appid = appid;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public Ipv6Subnet getSubnet() {
return subnet;
}
public void setSubnet(Ipv6Subnet subnet) {
this.subnet = subnet;
}
public Set getNodes() {
return nodes;
}
public boolean isFirewallEnabled() {
return isFirewallEnabled;
}
public void setFirewallEnabled(boolean firewallEnabled) {
isFirewallEnabled = firewallEnabled;
}
public void setNodes(Set nodes) {
this.nodes = nodes;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
public int getUid() {
return uid;
}
public int getOwnerUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public int getCreatorUid() {
return creatorUid;
}
public void setCreatorUid(int creatorUid) {
this.creatorUid = creatorUid;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public EnvironmentStatus getStatus() {
return EnvironmentStatus.getById(status);
}
public void setStatus(int status) {
this.updatedOn = new Date();
this.status = status;
}
public void setEnvironmentStatus(EnvironmentStatus status) {
setStatus(status.getValue());
}
public boolean isProtected() {
return isProtected;
}
public void setProtected(boolean aProtected) {
isProtected = aProtected;
}
public String getAppname() {
return appname;
}
public void setAppname(String appname) {
this.appname = appname;
}
public Set getContexts() {
return contexts;
}
public Domain getDomain() {
return domain;
}
public void setDomain(Domain domain) {
this.domain = domain;
}
public void setContexts(Set contexts) {
this.contexts = contexts;
}
public void setFullDomain(String fullDomain) {
this.fullDomain = fullDomain;
}
public String getFullDomain() {
if (StringUtils.isNotBlank(fullDomain)) {
return this.fullDomain;
}
if (hardwareNodeGroup == null) {
return this.fullDomain;
}
if (zone != null) {
return domain.getDomain() + "." + zone;
}
return domain.getDomain() + "." + hardwareNodeGroup.getRegion().getDomain();
}
public Set getExtDomains() {
return extDomains;
}
public void setExtDomains(Set extDomains) {
this.extDomains = extDomains;
}
public SSLCustom getCustomSSL() {
return customSSL;
}
public void setCustomSSL(SSLCustom customSSL) {
this.customSSL = customSSL;
}
public Engine getEngine() {
return engine;
}
public void setEngine(Engine engine) {
this.engine = engine;
}
public boolean getSslState() {
return sslState;
}
public void setSslState(boolean sslState) {
this.sslState = sslState;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
}
public WinDomain getWinDomain() {
return winDomain;
}
public void setWinDomain(WinDomain winDomain) {
this.winDomain = winDomain;
}
public boolean isHAEnabled() {
return isHAEnabled;
}
public void setHAEnabled(boolean isHAEnabled) {
this.isHAEnabled = isHAEnabled;
}
public int getIsUpToDate() {
return isUpToDate;
}
public void setIsUpToDate(int isUpToDate) {
this.isUpToDate = isUpToDate;
}
public Boolean getBroken() {
return isBroken;
}
public void setBroken(Boolean broken) {
isBroken = broken;
}
public Boolean isTransferring() {
return isTransferring;
}
public void setTransferring(Boolean isTransferring) {
this.isTransferring = isTransferring;
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public HardwareNodeGroup getHardwareNodeGroup() {
return hardwareNodeGroup;
}
public void setHardwareNodeGroup(HardwareNodeGroup hardwareNodeGroup) {
this.hardwareNodeGroup = hardwareNodeGroup;
}
public String getZone() {
if (zone != null) {
return zone;
}
return hardwareNodeGroup.getRegion().getDomain();
}
public Set getZones() {
if (zone != null) {
return new HashSet<>(Collections.singletonList(zone));
}
return hardwareNodeGroup.getRegion().getAllActiveDomainNames();
}
public SoftNodeGroup findSoftNodeGroup(String nodeGroup) {
SoftNodeGroup softNodeGroup = null;
for (SoftNodeGroup softNodeGroupItem : this.getSoftNodeGroups()) {
if (softNodeGroupItem.getName().equals(nodeGroup)) {
softNodeGroup = softNodeGroupItem;
break;
}
}
return softNodeGroup;
}
public SoftwareNode findSoftNode(int nodeId) {
for (SoftwareNode node : this.getNodes()) {
if (node.getId() == nodeId) {
return node;
}
}
return null;
}
public Set getSoftNodeGroups() {
Set softNodeGroups = new HashSet<>();
if (nodes != null) {
for (SoftwareNode node : nodes) {
SoftNodeGroup softNodeGroup = node.getSoftNodeGroup();
if (softNodeGroup != null) {
softNodeGroups.add(softNodeGroup);
}
}
}
return softNodeGroups;
}
public SoftNodeGroup getSoftNodeGroup(String name) {
if (nodes == null) {
return null;
}
for (SoftwareNode node : nodes) {
SoftNodeGroup softNodeGroup = node.getSoftNodeGroup();
if (softNodeGroup != null && softNodeGroup.getName().equals(name)) {
return softNodeGroup;
}
}
return null;
}
public SoftNodeGroup getSoftNodeGroup(int id) {
for (SoftNodeGroup softNodeGroup : getSoftNodeGroups()) {
if (softNodeGroup.getId() == id) {
return softNodeGroup;
}
}
return null;
}
public boolean isRemote() {
return isRemote;
}
public void setRemote(boolean remote) {
isRemote = remote;
}
public void setZone(String zone) {
this.zone = zone;
}
public boolean isShared() {
return isShared;
}
public void setShared(boolean shared) {
isShared = shared;
}
public String getNote() {
return note;
}
public void setNote(String note) {
this.note = note;
}
public Set getGroups() {
return groups;
}
public void setGroups(Set groups) {
this.groups = groups;
}
public Map getAttributesAsMap() {
return JSONUtils.toMap(attributes);
}
public void addAttribute(String key, String value) {
try {
getAttributesJson().putOpt(key, value);
this.attributes = getAttributesJson().toString();
} catch (JSONException e) {
e.printStackTrace();
}
}
public void addAttributes(Map attributesMap) {
if (attributesMap == null || attributesMap.isEmpty()) {
return;
}
try {
JSONObject json = getAttributesJson();
for (Map.Entry entry : attributesMap.entrySet()) {
json.putOpt(entry.getKey(), entry.getValue());
}
this.attributes = getAttributesJson().toString();
} catch (JSONException e) {
e.printStackTrace();
}
}
public void removeAttribute(String key) {
getAttributesJson().remove(key);
this.attributes = getAttributesJson().toString();
}
public JSONObject getAttributesJson() {
if (attributesJson == null) {
if (StringUtils.isNotBlank(attributes)) {
try {
attributesJson = new JSONObject(attributes);
} catch (JSONException e) {
e.printStackTrace();
}
}
if (attributesJson == null) {
attributesJson = new JSONObject();
}
}
return attributesJson;
}
public EnvironmentStatus getOriginalStatus() {
if (originalStatus == null) {
originalStatus = getStatus();
}
return originalStatus;
}
public void setOriginalStatus(EnvironmentStatus originalStatus) {
this.originalStatus = originalStatus;
}
public Set getDomains() {
return domains;
}
public Set getAllDomains() {
Set allDomains = new LinkedHashSet<>(domains.size() + 1);
allDomains.add(domain);
allDomains.addAll(domains);
return allDomains;
}
public void setDomains(Set domains) {
this.domains = domains;
}
private String getZoneByRegion() {
if (hardwareNodeGroup == null || hardwareNodeGroup.getRegion() == null) {
return null;
}
return hardwareNodeGroup.getRegion().getDomain();
}
public String getProperty(String key) {
if (StringUtils.isBlank(key)) {
return null;
}
return getPropertiesJson().optString(key, null);
}
public Project getProject() {
return project;
}
public void setProject(Project project) {
this.project = project;
}
public boolean hasProperty(String key) {
if (StringUtils.isBlank(key)) {
return false;
}
return getPropertiesJson().has(key);
}
public void setProperty(String key, Object value) throws JSONException {
if (StringUtils.isBlank(key)) {
return;
}
JSONObject propertyJson = getPropertiesJson();
propertyJson.put(key, value);
setProperties(propertyJson);
}
public Map getPropertiesAsMap() {
return JSONUtils.toMap(properties);
}
public void removeProperty(String... keys) {
JSONObject propertyJson = getPropertiesJson();
for (String key : keys) {
propertyJson.remove(key);
}
setProperties(propertyJson);
}
public JSONObject getPropertiesJson() {
if (StringUtils.isBlank(properties)) {
return new JSONObject();
}
try {
return new JSONObject(properties);
} catch (JSONException e) {
e.printStackTrace();
return null;
}
}
private void setProperties(JSONObject propertyJson) {
properties = propertyJson.toString();
}
public JSONObject toJSON(boolean isExport) throws JSONException {
JSONObject json = new JSONObject();
json.put(STATUS, this.status);
json.put(APPID, this.appid);
json.put(SSLSTATE, this.sslState);
if (this.getFullDomain() != null) {
json.put(DOMAIN, this.getFullDomain().toLowerCase());
if (this.domain != null) {
json.put(SHORTDOMAIN, this.domain.getDomain());
json.put(ENV_NAME, this.domain.getDomain());
}
} else if (domain != null) {
json.put(DOMAIN, this.domain.getDomain().toLowerCase());
json.put(SHORTDOMAIN, this.domain.getDomain());
json.put(ENV_NAME, this.domain.getDomain());
}
if (domains != null && !domains.isEmpty()) {
json.put(ALIASES, domains.stream().map(Domain::getDomain).collect(Collectors.toSet()));
}
if (this.uid != 0) {
json.put(UID, this.uid);
json.put(OWNER_UID, this.uid);
}
if (this.creatorUid != 0) {
json.put(CREATOR_UID, this.creatorUid);
}
if (this.appname != null) {
json.put(APPNAME, this.appname);
}
if (this.engine != null) {
json.put(ENGINE, this.engine._toJSON());
}
if (this.winDomain != null) {
json.put(WINDOMAIN, this.winDomain._toJSON());
}
if (nodes != null) {
SoftwareNode masterSoftNode = NodeUtils.selectSoftwareNodesByNodeGroup(nodes, NodeUtils.NODE_MISSION_COMPUTE, true);
JSONArray contextsJson = new JSONArray();
if (masterSoftNode != null) {
SoftNodeGroup softNodeGroup = masterSoftNode.getSoftNodeGroup();
if (softNodeGroup != null) {
for (AppContext context : softNodeGroup.getContexts()) {
JSONObject contextJson = context._toJSON();
contextsJson.put(contextJson);
}
}
}
json.put(CONTEXTS, contextsJson);
}
if (customSSL != null) {
json.put(CUSTOM_SSL, customSSL.toJSON(isExport));
}
if (extDomains != null) {
JSONArray domainsJson = new JSONArray();
for (ExtDomain extDomain : extDomains) {
domainsJson.put(extDomain.getDomain());
}
json.put(EXTDOMAINS, domainsJson);
}
json.put(IS_HA_ENABLED, this.isHAEnabled);
json.put(IS_FIREWALL_ENABLED, this.isFirewallEnabled);
if (createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(createdOn));
}
if (note != null) {
json.put(NOTE, note);
}
json.put(IS_BROKEN, isBroken);
json.put(IS_REMOTE, isRemote);
json.put(IS_TRANSFERRING, isTransferring);
json.put(DISPLAY_NAME, displayName);
if (hardwareNodeGroup != null) {
json.put(HARDWARE_NODE_GROUP, hardwareNodeGroup.getUniqueName());
JSONObject hostGroupJson = new JSONObject();
hostGroupJson.put(HOST_GROUP_UNIQUE_NAME, hardwareNodeGroup.getUniqueName());
hostGroupJson.put(HOST_GROUP_DISPLAY_NAME, hardwareNodeGroup.getDisplayName());
json.put(HOST_GROUP, hostGroupJson);
}
if (isShared != null) {
json.put(IS_SHARED, isShared);
}
if (getAttributesJson() != null) {
json.put(ATTRIBUTES, getAttributesJson());
if (getAttributesJson().has(Constants.POLICIES)) {
json.put(Constants.POLICIES, new JSONArray(getAttributesJson().getString(Constants.POLICIES)));
}
}
if (properties != null) {
json.put(PROPERTIES, getPropertiesJson());
}
return json;
}
@Override
public JSONObject _toJSON() throws JSONException {
return toJSON(false);
}
@Override
public AppNodes _fromJSON(JSONObject json) throws JSONException {
if (json.has(STATUS)) {
this.status = json.getInt(STATUS);
}
if (json.has(APPID)) {
this.appid = json.getString(APPID);
}
if (json.has(APPNAME)) {
this.appname = json.getString(APPNAME);
}
if (json.has(DOMAIN)) {
this.fullDomain = json.getString(DOMAIN);
}
if (json.has(ENV_NAME) || json.has(SHORTDOMAIN)) {
this.domain = json.has(ENV_NAME) ? new Domain(json.getString(ENV_NAME)) : new Domain(json.getString(SHORTDOMAIN));
}
if (json.has(SSLSTATE)) {
this.sslState = json.getBoolean(SSLSTATE);
}
if (json.has(UID)) {
this.uid = json.getInt(UID);
}
if (json.has(CREATOR_UID)) {
this.creatorUid = json.getInt(CREATOR_UID);
}
if (json.has(ENGINE)) {
this.engine = new Engine()._fromJSON(json.getJSONObject(ENGINE));
}
if (json.has(WINDOMAIN)) {
this.winDomain = (WinDomain) new WinDomain()._fromJSON(json.getJSONObject(WINDOMAIN));
}
if (json.has(CONTEXTS)) {
JSONArray contextsJson = json.getJSONArray(CONTEXTS);
for (int i = 0; i < contextsJson.length(); i++) {
JSONObject contextJson = contextsJson.getJSONObject(i);
AppContext context = new AppContext()._fromJSON(contextJson);
contexts.add(context);
}
}
if (json.has(CUSTOM_SSL)) {
this.customSSL = new SSLCustom()._fromJSON(json.getJSONObject(CUSTOM_SSL));
}
if (json.has(EXTDOMAINS)) {
JSONArray domainsJson = json.getJSONArray(EXTDOMAINS);
for (int i = 0; i < domainsJson.length(); i++) {
extDomains.add(new ExtDomain(domainsJson.getString(i)));
}
}
if (json.has(IS_HA_ENABLED)) {
this.isHAEnabled = json.getBoolean(IS_HA_ENABLED);
}
if (json.has(CREATED_ON)) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString(CREATED_ON));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has(IS_BROKEN)) {
this.isBroken = json.getBoolean(IS_BROKEN);
}
if (json.has(IS_TRANSFERRING)) {
isTransferring = json.getBoolean(IS_TRANSFERRING);
}
if (json.has(IS_REMOTE)) {
isRemote = json.getBoolean(IS_REMOTE);
}
if (json.has(DISPLAY_NAME)) {
displayName = json.getString(DISPLAY_NAME);
}
if (json.has(NOTE)) {
note = json.getString(NOTE);
}
if (json.has(IS_SHARED)) {
isShared = json.getBoolean(IS_SHARED);
}
if (json.has(HARDWARE_NODE_GROUP)) {
hardwareNodeGroup = new HardwareNodeGroup();
hardwareNodeGroup.setUniqueName(json.getString(HARDWARE_NODE_GROUP));
}
if (json.has(HOST_GROUP)) {
JSONObject hostGroup = json.getJSONObject(HOST_GROUP);
hardwareNodeGroup = new HardwareNodeGroup();
if (hostGroup.has(HOST_GROUP_UNIQUE_NAME)) {
hardwareNodeGroup.setUniqueName(hostGroup.getString(HOST_GROUP_UNIQUE_NAME));
}
if (hostGroup.has(HOST_GROUP_DISPLAY_NAME)) {
hardwareNodeGroup.setDisplayName(hostGroup.getString(HOST_GROUP_DISPLAY_NAME));
}
}
if (json.has(IS_FIREWALL_ENABLED)) {
this.isFirewallEnabled = json.getBoolean(IS_FIREWALL_ENABLED);
}
if (json.has(ALIASES)) {
JSONArray domainsJson = json.getJSONArray(ALIASES);
for (int i = 0; i < domainsJson.length(); i++) {
String domainStr = domainsJson.getString(i);
domains.add(new Domain(domainStr));
}
}
if (json.has(PROPERTIES)) {
JSONObject propertiesJsonObject = json.getJSONObject(PROPERTIES);
properties = propertiesJsonObject.toString();
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy