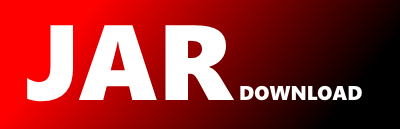
com.jelastic.api.system.persistence.CrossNetworkIpAddress Maven / Gradle / Ivy
The newest version!
/*Server class MD5: b3de89cd4af418a4ada4720add9077ca*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Date;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class CrossNetworkIpAddress extends ArrayItem {
public static final String IP_ADDRESS = "ipAddress";
public static final String ID = "id";
public static final String IS_BUSY = "isBusy";
public static final String REGION = "region";
public static final String APPID = "appid";
public static final String UPDATED_ON = "updatedOn";
public static final String DOMAIN = "domain";
private String ipAddress;
private boolean isBusy;
private Region region;
private OsNode osNode;
private Date updatedBusyOn;
private Date updatedOn;
private String domain;
private String appid;
public CrossNetworkIpAddress() {
}
public CrossNetworkIpAddress(String ipAddress, Region region) {
this.ipAddress = ipAddress;
this.region = region;
}
public OsNode getOsNode() {
return osNode;
}
public void setOsNode(OsNode osNode) {
this.updatedOn = new Date();
this.osNode = osNode;
}
public boolean isBusy() {
return isBusy;
}
public void setBusy(boolean isBusy) {
this.updatedBusyOn = new Date();
this.isBusy = isBusy;
}
public void setRegion(Region region) {
this.region = region;
}
public Region getRegion() {
return region;
}
public Date getUpdatedBusyOn() {
return updatedBusyOn;
}
public void setUpdatedBusyOn(Date updatedBusyOn) {
this.updatedBusyOn = updatedBusyOn;
}
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public void setDomain(String domain) {
this.domain = domain;
}
public void setAppid(String appid) {
this.appid = appid;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(IP_ADDRESS, ipAddress);
json.put(ID, id);
json.put(IS_BUSY, isBusy);
json.put(REGION, region.getUniqueName());
json.put(UPDATED_ON, updatedOn);
if (appid != null) {
json.put(APPID, appid);
}
if (domain != null) {
json.put(DOMAIN, domain);
}
return json;
}
@Override
public ArrayItem _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(IP_ADDRESS)) {
this.ipAddress = json.getString(IP_ADDRESS);
}
if (json.has(IS_BUSY)) {
this.isBusy = json.getBoolean(IS_BUSY);
}
if (json.has(REGION)) {
this.region = new Region();
this.region.setUniqueName(json.getString(REGION));
}
if (json.has(UPDATED_ON)) {
this.updatedOn = new Date(json.getLong(UPDATED_ON));
}
if (json.has(APPID)) {
this.appid = json.getString(APPID);
}
if (json.has(DOMAIN)) {
this.domain = json.getString(DOMAIN);
}
return this;
}
@Override
public String toString() {
return "ExtIpAddress{" + "ipAddress='" + ipAddress + '\'' + ", isBusy=" + isBusy + ", appid='" + appid + '\'' + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy