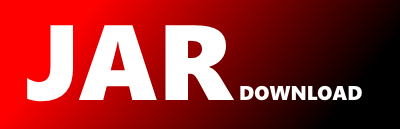
com.jelastic.api.system.persistence.Distributor Maven / Gradle / Ivy
The newest version!
/*Server class MD5: ed4cd7862980052f174758f90bf5feb3*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Distributor extends ArrayItem implements Serializable {
public static final String ID = "id";
public static final String NAME = "name";
public static final String EMAIL = "email";
public static final String DESCRIPTION = "description";
public static final String CREATED_ON = "createdOn";
public static final String RESELLERS = "resellers";
private String name;
private String email;
private String description;
private Date createdOn;
private boolean isDeleted;
private Set resellers = new HashSet<>();
public Distributor() {
this.createdOn = new Date();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public Set getResellers() {
return resellers;
}
public void setResellers(Set resellers) {
this.resellers = resellers;
}
@Override
public ArrayItem _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(EMAIL)) {
this.email = json.getString(EMAIL);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
return this;
}
@Override
public JSONObject _toJSON() throws JSONException {
return this._toJSON(true);
}
public JSONObject _toJSON(boolean includeResellers) throws JSONException {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NAME, name);
json.put(EMAIL, email);
json.putOpt(DESCRIPTION, description);
json.put(CREATED_ON, dateFormat.format(createdOn));
if (includeResellers && resellers != null) {
JSONArray array = new JSONArray();
for (Reseller reseller : resellers) {
array.put(reseller._toJSON());
}
json.put(RESELLERS, array);
}
return json;
}
@Override
public String toString() {
return "Distributor{" + "name='" + name + '\'' + ", email='" + email + '\'' + ", description='" + description + '\'' + ", createdOn=" + createdOn + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy