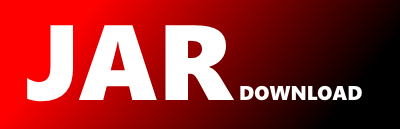
com.jelastic.api.system.persistence.Engine Maven / Gradle / Ivy
/*Server class MD5: 3ffe68c8482b3c654f88ea9be4ff2da4*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Engine extends ArrayItem {
public static final String ID = "id";
public static final String NAME = "name";
public static final String TYPE = "type";
public static final String VERSION = "version";
public static final String KEYWORD = "keyword";
public static final String VCS_SUPPORT = "vcsSupport";
private String name;
private String type;
private String version;
private String keyword;
private boolean vcsSupport;
private String packageName;
public Engine(int id, String name, String type, String version, String keyword, boolean vcsSupport, String packageName) {
this.id = id;
this.name = name;
this.type = type;
this.version = version;
this.keyword = keyword;
this.vcsSupport = vcsSupport;
this.packageName = packageName;
}
public Engine() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getKeyword() {
return keyword;
}
public void setKeyword(String keyword) {
this.keyword = keyword;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getPackagesName() {
return packageName;
}
public void setPackagesName(String packageName) {
this.packageName = packageName;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NAME, name);
json.put(TYPE, type);
json.put(VERSION, version);
json.put(KEYWORD, keyword);
json.put(VCS_SUPPORT, vcsSupport);
return json;
}
@Override
public Engine _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(TYPE)) {
this.type = json.getString(TYPE);
}
if (json.has(VERSION)) {
this.version = json.getString(VERSION);
}
if (json.has(KEYWORD)) {
this.keyword = json.getString(KEYWORD);
}
if (json.has(VCS_SUPPORT)) {
this.vcsSupport = json.getBoolean(VCS_SUPPORT);
}
return this;
}
public boolean isVcsSupport() {
return vcsSupport;
}
public void setVcsSupport(boolean vcsSupport) {
this.vcsSupport = vcsSupport;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy