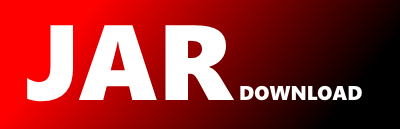
com.jelastic.api.system.persistence.ExtIpAddress Maven / Gradle / Ivy
The newest version!
/*Server class MD5: a3bf7f5afc2e148c6d8c4d33a645abd9*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ExtIpAddress extends ArrayItem {
public static final String IP_ADDRESS = "ipAddress";
public static final String ID = "id";
public static final String IS_BUSY = "isBusy";
public static final String REGIONS = "regions";
public static final String DOMAIN = "domain";
public static final String APPID = "appid";
public static final String TYPE = "type";
private String ipAddress;
private boolean isBusy;
private String domain;
private String appid;
private Set regions = new HashSet<>();
private IpType type;
private Ipv6Subnet subnet;
private OsNode osNode;
private Date updatedBusyOn;
private Date updatedOn;
private boolean shortInfoMode = false;
public ExtIpAddress() {
}
public ExtIpAddress(String ipAddress, boolean isBusy) {
this.ipAddress = ipAddress;
this.isBusy = isBusy;
}
public OsNode getOsNode() {
return osNode;
}
public void setOsNode(OsNode osNode) {
this.updatedOn = new Date();
this.osNode = osNode;
}
public boolean isBusy() {
return isBusy;
}
public void setBusy(boolean isBusy) {
this.updatedBusyOn = new Date();
this.isBusy = isBusy;
}
public Date getUpdatedBusyOn() {
return updatedBusyOn;
}
public void setUpdatedBusyOn(Date updatedBusyOn) {
this.updatedBusyOn = updatedBusyOn;
}
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public IpType getType() {
return type;
}
public void setType(IpType type) {
this.type = type;
}
public Ipv6Subnet getSubnet() {
return subnet;
}
public void setSubnet(Ipv6Subnet subnet) {
this.subnet = subnet;
}
public void setShortInfoMode(boolean mode) {
this.shortInfoMode = mode;
}
public Set getRegions() {
return regions;
}
public void setRegions(Set regions) {
this.regions = regions;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public String getKey(String prefix) {
String key = this.ipAddress;
if (prefix != null) {
key = prefix + key;
}
return key;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(IP_ADDRESS, ipAddress);
if (shortInfoMode) {
return json;
}
json.put(ID, id);
json.put(IS_BUSY, isBusy);
JSONArray regionUniqueNames = new JSONArray();
for (Region region : regions) {
regionUniqueNames.put(region.getUniqueName());
}
json.put(REGIONS, regionUniqueNames);
json.put(DOMAIN, domain);
json.put(APPID, appid);
json.put(TYPE, type.name());
return json;
}
@Override
public ExtIpAddress _fromJSON(JSONObject jsonObject) throws JSONException {
if (jsonObject.has(IP_ADDRESS)) {
this.ipAddress = jsonObject.getString(IP_ADDRESS);
}
if (jsonObject.has(ID)) {
this.id = jsonObject.getInt(ID);
}
if (jsonObject.has(IS_BUSY)) {
this.isBusy = jsonObject.getBoolean(IS_BUSY);
}
if (jsonObject.has(REGIONS)) {
JSONArray regions = jsonObject.getJSONArray(REGIONS);
for (int i = 0; i < regions.length(); i++) {
if (regions.get(i) instanceof String) {
Region region = new Region();
region.setUniqueName(regions.getString(i));
this.regions.add(region);
}
if (regions.get(i) instanceof JSONObject) {
this.regions.add(new Region()._fromJSON(regions.getJSONObject(i)));
}
}
}
if (jsonObject.has(DOMAIN)) {
this.domain = jsonObject.getString(DOMAIN);
}
if (jsonObject.has(APPID)) {
this.appid = jsonObject.getString(APPID);
}
if (jsonObject.has(TYPE)) {
this.type = IpType.valueOf(jsonObject.getString(TYPE));
}
return this;
}
@Override
public String toString() {
return "ExtIpAddress{" + "ipAddress='" + ipAddress + '\'' + ", isBusy=" + isBusy + ", domain='" + domain + '\'' + ", appid='" + appid + '\'' + ", regions=" + regions + ", type=" + type + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy