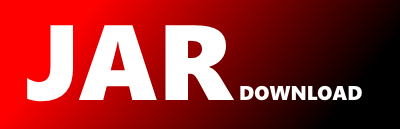
com.jelastic.api.system.persistence.FirewallRule Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 8e7041296127c2ed3bcfeb8a1cc7a847*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.common.Constants;
import com.jelastic.api.core.utils.CollectionUtils;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class FirewallRule extends ArrayItem implements Comparable, Cloneable {
private static final String IPV4_REGEX = "^(((25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\\.){3}(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)(\\/([0-9]|[1-9][0-9]|1[0-2][0-8]|1[0-1][0-9]))?|,)+$";
private static final String IPV6_REGEX = "^((([0-9a-fA-F]{1,4}:){7,7}[0-9a-fA-F]{1,4}|([0-9a-fA-F]{1,4}:){1,7}:|([0-9a-fA-F]{1,4}:){1,6}:[0-9a-fA-F]{1,4}|([0-9a-fA-F]{1,4}:){1,5}(:[0-9a-fA-F]{1,4}){1,2}|([0-9a-fA-F]{1,4}:){1,4}(:[0-9a-fA-F]{1,4}){1,3}|([0-9a-fA-F]{1,4}:){1,3}(:[0-9a-fA-F]{1,4}){1,4}|([0-9a-fA-F]{1,4}:){1,2}(:[0-9a-fA-F]{1,4}){1,5}|[0-9a-fA-F]{1,4}:((:[0-9a-fA-F]{1,4}){1,6})|:((:[0-9a-fA-F]{1,4}){1,7}|:)|fe80:(:[0-9a-fA-F]{0,4}){0,4}%[0-9a-zA-Z]{1,}|::(ffff(:0{1,4}){0,1}:){0,1}((25[0-5]|(2[0-4]|1{0,1}[0-9]){0,1}[0-9])\\.){3,3}(25[0-5]|(2[0-4]|1{0,1}[0-9]){0,1}[0-9])|([0-9a-fA-F]{1,4}:){1,4}:((25[0-5]|(2[0-4]|1{0,1}[0-9]){0,1}[0-9])\\.){3,3}(25[0-5]|(2[0-4]|1{0,1}[0-9]){0,1}[0-9]))(\\/(1[0-1][0-9]|1[0-2][0-8]|[1-9][0-9]|[0-9]))?|,)+$";
private static final String DOMAIN_REGEX = "(([a-zA-Z]{1})|([a-zA-Z]{1}[a-zA-Z]{1})|([a-zA-Z]{1}[0-9]{1})|([0-9]{1}[a-zA-Z]{1})|([a-zA-Z0-9][a-zA-Z0-9-_]{1,61}[a-zA-Z0-9]))\\.([a-zA-Z]{2,6}|[a-zA-Z0-9-]{2,30}\\.[a-zA-Z]{2,3})";
private static final String PORTS_REGEX = "^([1-9][0-9]{0,3}|[1-5][0-9]{4}|6[0-4][0-9]{3}|65[0-4][0-9]{2}|655[0-2][0-9]|6553[0-5])(?:-([1-9][0-9]{0,3}|[1-5][0-9]{4}|6[0-4][0-9]{3}|65[0-4][0-9]{2}|655[0-2][0-9]|6553[0-5]))?$|^$";
public static final String ALL = "ALL";
public static final String ALL_IPV4 = "ALL_IPV4";
public static final String ALL_IPV6 = "ALL_IPV6";
public static final String LOCAL = "LOCAL";
public static final String PUBLIC_ACCESS = "PUBLIC_ACCESS";
public static final String IPV4_ZERO = "0.0.0.0/0";
public static final int FIREWALL_PRIORITY_INCREMENT_STEP = 10;
public static final int MAX_PRIORITY = 65535;
public static final int DEFAULT_PRIORITY = 1000;
private String name;
private Date updatedOn;
private FirewallRuleDirection direction;
private FirewallRuleAction action;
private String src = ALL;
private String dst = ALL;
private String ports = "";
private String protocol = "ALL";
private int priority = DEFAULT_PRIORITY;
private SoftNodeGroup softNodeGroup;
private boolean isDeleted;
private Date createdOn;
private boolean isEnabled = true;
private FirewallRuleType type = FirewallRuleType.CUSTOM;
private SoftNodeGroup relatedSoftNodeGroup;
private Integer uid;
private String email;
private String relatedSoftNodeGroupName = null;
private String relatedEnvName = null;
private boolean isInfra = false;
public static final String NAME = "name";
public static final String UPDATED_ON = "updatedOn";
public static final String TYPE = "type";
public static final String DIRECTION = "direction";
public static final String ACTION = "action";
public static final String SRC = "src";
public static final String DST = "dst";
public static final String PORTS = "ports";
public static final String PROTOCOL = "protocol";
public static final String PRIORITY = "priority";
public static final String NODE_GROUP = "nodeGroup";
public static final String RELATED_NODE_GROUP = "relatedNodeGroup";
public static final String RELATED_ENV_NAME = "relatedEnvName";
public static final String CREATED_ON = "createdOn";
public static final String IS_ENABLED = "isEnabled";
public static final String EMAIL = "email";
public FirewallRule(String name, FirewallRuleDirection direction, FirewallRuleAction action, String ports, String protocol, FirewallRuleType type) {
this();
this.name = name;
this.direction = direction;
this.action = action;
this.ports = ports;
this.protocol = protocol;
this.type = type;
}
public FirewallRule(String name, FirewallRuleDirection direction, FirewallRuleAction action, String ports, String protocol, FirewallRuleType type, int priority, SoftNodeGroup softNodeGroup) {
this();
this.name = name;
this.direction = direction;
this.action = action;
this.ports = ports;
this.protocol = protocol;
this.type = type;
this.priority = priority;
this.softNodeGroup = softNodeGroup;
}
public FirewallRule() {
createdOn = new Date();
updatedOn = createdOn;
}
public FirewallRule(SoftwareNode cache) {
super();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public FirewallRuleDirection getDirection() {
return direction;
}
public void setDirection(FirewallRuleDirection direction) {
this.direction = direction;
}
public FirewallRuleAction getAction() {
return action;
}
public void setAction(FirewallRuleAction action) {
this.action = action;
}
public String getSrc() {
return src;
}
public void setSrc(String src) {
this.src = src;
}
public String getDst() {
return dst;
}
public void setDst(String dst) {
this.dst = dst;
}
public String getPorts() {
return ports;
}
public void setPorts(String ports) {
this.ports = ports;
}
public String getProtocol() {
return protocol;
}
public void setProtocol(String protocol) {
this.protocol = protocol;
}
public int getPriority() {
return priority;
}
public void setPriority(int priority) {
this.priority = priority;
}
public boolean isInfra() {
return isInfra;
}
public void setInfra(boolean infra) {
if (infra) {
this.priority = 1;
}
isInfra = infra;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public SoftNodeGroup getSoftNodeGroup() {
return softNodeGroup;
}
public void setSoftNodeGroup(SoftNodeGroup softNodeGroup) {
this.softNodeGroup = softNodeGroup;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public boolean isEnabled() {
return isEnabled;
}
public void setEnabled(boolean enabled) {
isEnabled = enabled;
}
private boolean isPortsContainsRange() {
return StringUtils.contains(ports, "-");
}
public FirewallRuleType getType() {
return type;
}
public void setType(FirewallRuleType type) {
this.type = type;
}
public SoftNodeGroup getRelatedSoftNodeGroup() {
return relatedSoftNodeGroup;
}
public void setRelatedSoftNodeGroup(SoftNodeGroup relatedSoftNodeGroup) {
this.relatedSoftNodeGroup = relatedSoftNodeGroup;
}
public String getRelatedSoftNodeGroupName() {
if (relatedEnvName == null && relatedSoftNodeGroup != null) {
return relatedSoftNodeGroup.getName();
}
return relatedSoftNodeGroupName;
}
public String getRelatedEnvName() {
return relatedEnvName;
}
public void setRelatedEnvName(String relatedEnvName) {
this.relatedEnvName = relatedEnvName;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public Integer getUid() {
return uid;
}
public void setEmail(String email) {
this.email = email;
}
private IpType getTransportProtocol() {
if (StringUtils.contains(src, Constants.COLON_SEPARATOR) || StringUtils.contains(dst, Constants.COLON_SEPARATOR) || StringUtils.equals(dst, "ALL_IPV6") || StringUtils.equals(src, "ALL_IPV6")) {
return IpType.IPV6;
}
return IpType.IPV4;
}
public void merge(FirewallRule entity) {
if (!StringUtils.equals(name, entity.getName())) {
this.name = entity.getName();
}
if (!StringUtils.equals(src, entity.getSrc())) {
this.src = entity.getSrc();
}
if (!StringUtils.equals(dst, entity.getDst())) {
this.dst = entity.getDst();
}
if (!StringUtils.equals(ports, entity.getPorts())) {
this.ports = entity.getPorts();
}
if (!StringUtils.equals(protocol, entity.getProtocol())) {
this.protocol = entity.getProtocol();
}
if (direction != entity.getDirection()) {
this.direction = entity.getDirection();
}
if (action != entity.getAction()) {
this.action = entity.getAction();
}
if (priority != entity.getPriority()) {
priority = entity.getPriority();
}
if (isEnabled != entity.isEnabled()) {
this.isEnabled = entity.isEnabled();
}
if (type != entity.getType()) {
type = FirewallRuleType.CUSTOM;
}
if (entity.getRelatedSoftNodeGroup() != null && !entity.getRelatedSoftNodeGroup().equals(getRelatedSoftNodeGroup())) {
setRelatedSoftNodeGroup(entity.getRelatedSoftNodeGroup());
} else if (entity.getRelatedSoftNodeGroup() == null) {
setRelatedSoftNodeGroup(null);
setRelatedEnvName(null);
relatedSoftNodeGroupName = null;
}
this.updatedOn = new Date();
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
if (id != 0) {
json.put("id", id);
}
json.put(NAME, name);
json.put(DIRECTION, direction);
json.put(ACTION, action);
json.put(SRC, src);
json.put(DST, dst);
json.put(PORTS, ports);
json.put(PROTOCOL, protocol);
json.put(PRIORITY, priority);
if (type != null) {
json.put(TYPE, type);
}
if (softNodeGroup != null) {
json.put(NODE_GROUP, softNodeGroup.getName());
}
if (createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(createdOn));
}
if (updatedOn != null) {
json.put(UPDATED_ON, DateUtils.formatSqlDateTime(updatedOn));
}
json.put(IS_ENABLED, isEnabled);
if (relatedSoftNodeGroup != null) {
json.put(RELATED_NODE_GROUP, relatedSoftNodeGroup.getName());
}
if (relatedEnvName != null) {
json.put(RELATED_ENV_NAME, relatedEnvName);
}
if (uid != null) {
json.put(EMAIL, email);
}
return json;
}
@Override
public FirewallRule _fromJSON(JSONObject json) throws JSONException {
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(UPDATED_ON)) {
this.name = json.getString(NAME);
}
if (json.has(DIRECTION)) {
this.direction = FirewallRuleDirection.value(json.getString(DIRECTION));
}
if (json.has(ACTION)) {
this.action = FirewallRuleAction.valueOf(FirewallRuleAction.class, json.getString(ACTION).toUpperCase());
}
if (direction == FirewallRuleDirection.INPUT) {
if (json.has(SRC)) {
this.src = json.getString(SRC);
}
} else {
if (json.has(DST)) {
this.dst = json.getString(DST);
}
}
if (json.has(PORTS)) {
this.ports = json.getString(PORTS).replaceAll("\\s+", "");
}
if (json.has(PROTOCOL)) {
this.protocol = json.getString(PROTOCOL);
}
if (json.has(PRIORITY)) {
this.priority = json.getInt(PRIORITY);
}
if (json.has(IS_ENABLED)) {
this.isEnabled = json.getBoolean(IS_ENABLED);
}
if (json.has(RELATED_NODE_GROUP)) {
this.relatedSoftNodeGroupName = json.getString(RELATED_NODE_GROUP);
this.src = "";
this.dst = "";
}
if (json.has(RELATED_ENV_NAME)) {
this.relatedEnvName = json.getString(RELATED_ENV_NAME);
}
return this;
}
public List getRuleCommands(Collection privateNetworks) {
List list = new ArrayList<>();
if (StringUtils.equalsIgnoreCase(src, LOCAL) || StringUtils.equalsIgnoreCase(dst, LOCAL)) {
privateNetworks.forEach(network -> list.add(getCommand(getDirection(), network, null, IpType.IPV4, getAction())));
return list;
} else if (StringUtils.startsWith(src, ALL) && StringUtils.isEmpty(dst) || StringUtils.startsWith(dst, ALL) && StringUtils.isEmpty(src) || StringUtils.startsWith(src, ALL) && StringUtils.startsWith(dst, ALL)) {
switch(direction) {
case INPUT:
if (StringUtils.equals(src, ALL_IPV4) || StringUtils.equals(src, ALL)) {
list.add(getCommand(FirewallRuleDirection.INPUT, IPV4_ZERO, null, IpType.IPV4, action));
}
if (StringUtils.equals(src, ALL_IPV6) || StringUtils.equals(src, ALL)) {
list.add(getCommand(FirewallRuleDirection.INPUT, null, null, IpType.IPV6, action));
}
break;
case OUTPUT:
if (StringUtils.equals(dst, ALL_IPV4) || StringUtils.equals(dst, ALL)) {
list.add(getCommand(FirewallRuleDirection.OUTPUT, null, IPV4_ZERO, IpType.IPV4, action));
}
if (StringUtils.equals(dst, ALL_IPV6) || StringUtils.equals(dst, ALL)) {
list.add(getCommand(FirewallRuleDirection.OUTPUT, null, null, IpType.IPV6, action));
}
break;
}
return list;
} else if (StringUtils.contains(src, Constants.COMMA_SEPARATOR) || StringUtils.contains(dst, Constants.COMMA_SEPARATOR)) {
switch(direction) {
case INPUT:
Arrays.stream(src.split(Constants.COMMA_SEPARATOR)).filter(host -> StringUtils.isNotEmpty(host)).forEach(host -> list.add(getCommand(FirewallRuleDirection.INPUT, host, null, host.contains(Constants.COLON_SEPARATOR) ? IpType.IPV6 : IpType.IPV4, getAction())));
break;
case OUTPUT:
Arrays.stream(dst.split(Constants.COMMA_SEPARATOR)).filter(host -> StringUtils.isNotEmpty(host)).forEach(host -> list.add(getCommand(FirewallRuleDirection.OUTPUT, null, host, host.contains(Constants.COLON_SEPARATOR) ? IpType.IPV6 : IpType.IPV4, getAction())));
break;
}
return list;
} else if (StringUtils.equals(src, PUBLIC_ACCESS) || StringUtils.equals(dst, PUBLIC_ACCESS)) {
switch(direction) {
case INPUT:
privateNetworks.forEach(network -> list.add(getCommand(FirewallRuleDirection.INPUT, Constants.EXCLAMATION + network, null, IpType.IPV4, getAction())));
if (this.action == FirewallRuleAction.DENY) {
list.add(getCommand(FirewallRuleDirection.INPUT, IPV4_ZERO, null, IpType.IPV4, FirewallRuleAction.DENY));
}
break;
case OUTPUT:
privateNetworks.forEach(network -> list.add(getCommand(FirewallRuleDirection.OUTPUT, null, Constants.EXCLAMATION + network, IpType.IPV4, getAction())));
if (this.action == FirewallRuleAction.DENY) {
list.add(getCommand(FirewallRuleDirection.INPUT, IPV4_ZERO, null, IpType.IPV4, FirewallRuleAction.DENY));
}
break;
}
return list;
} else if (relatedSoftNodeGroup != null && !CollectionUtils.isEmpty(this.relatedSoftNodeGroup.getSoftwareNodes())) {
for (SoftwareNode node : relatedSoftNodeGroup.getSoftwareNodes()) {
list.addAll(node.getExtIpV4Addresses().stream().map(ip -> getCommand(direction, ip.getIpAddress(), null, IpType.IPV4, action)).collect(Collectors.toList()));
list.addAll(node.getExtIpV6Addresses().stream().map(ip -> getCommand(direction, ip.getIpAddress(), null, IpType.IPV6, action)).collect(Collectors.toList()));
if (node.getIntIP() != null) {
list.add(getCommand(direction, node.getIntIP(), null, IpType.IPV4, action));
}
}
return list;
}
list.add(getCommand(direction, src, dst, getTransportProtocol(), action));
return list;
}
@Override
public int compareTo(FirewallRule o) {
if (softNodeGroup != null && o.getSoftNodeGroup() != null) {
int comparation = Integer.compare(softNodeGroup.getId(), o.getSoftNodeGroup().getId());
if (comparation != 0) {
return comparation;
}
}
int value = Integer.compare(getPriority(), o.getPriority());
if (value == 0) {
if (this.getType() == FirewallRuleType.SYSTEM) {
return 1;
}
if (o.getType() == FirewallRuleType.SYSTEM) {
return -1;
}
return Integer.compare(getId(), o.getId());
}
return value;
}
public boolean compare(FirewallRule rule) {
boolean isEqual = true;
isEqual &= this.getDirection() == rule.getDirection();
isEqual &= this.getAction() == rule.getAction();
isEqual &= StringUtils.equals(this.getProtocol(), rule.getProtocol());
isEqual &= StringUtils.equals(this.getSrc(), rule.getSrc());
isEqual &= StringUtils.equals(this.getDst(), rule.getDst());
isEqual &= StringUtils.equals(this.getPorts(), rule.getPorts());
if (this.getSoftNodeGroup() != null && rule.getSoftNodeGroup() != null) {
isEqual &= this.getSoftNodeGroup().getId() == rule.getSoftNodeGroup().getId();
}
return isEqual;
}
private String getCommand(FirewallRuleDirection direction, String src, String dst, IpType ipType, FirewallRuleAction action) {
String cmd = Optional.ofNullable(protocol).orElse("") + "," + (isPortsContainsRange() ? "range" : "single") + "," + Optional.of(ports.replace(Constants.HYPHEN_SEPARATOR, Constants.COLON_SEPARATOR)).orElse("") + "," + Optional.ofNullable(src).orElse("") + "," + Optional.ofNullable(dst).orElse("") + "," + direction + "," + action.getJemName() + "," + ipType + "," + (isInfra ? "I" : "A");
return cmd;
}
@Override
public String toString() {
return "FirewallRule{" + "name='" + name + '\'' + ", updatedOn=" + updatedOn + ", direction=" + direction + ", action=" + action + ", src='" + src + '\'' + ", dst='" + dst + '\'' + ", ports='" + ports + '\'' + ", protocol='" + protocol + '\'' + ", priority=" + priority + ", softNodeGroup=" + softNodeGroup + ", isDeleted=" + isDeleted + ", createdOn=" + createdOn + ", isEnabled=" + isEnabled + ", type=" + type + ", relatedSoftNodeGroup=" + relatedSoftNodeGroup + ", uid=" + uid + ", email='" + email + '\'' + ", relatedSoftNodeGroupName='" + relatedSoftNodeGroupName + '\'' + ", relatedEnvName='" + relatedEnvName + '\'' + ", isInfra=" + isInfra + '}';
}
@Override
public FirewallRule clone() {
FirewallRule rule = new FirewallRule();
rule.setName(name);
rule.setUpdatedOn(updatedOn);
rule.setDirection(direction);
rule.setAction(action);
rule.setSrc(src);
rule.setDst(dst);
rule.setPorts(ports);
rule.setProtocol(protocol);
rule.setPriority(priority);
rule.setEnabled(isEnabled);
rule.setType(type);
rule.setInfra(isInfra);
rule.setRelatedSoftNodeGroup(relatedSoftNodeGroup);
rule.setUid(rule.getUid());
return rule;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
return super.equals(o) && compare((FirewallRule) o);
}
@Override
public int hashCode() {
return super.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy