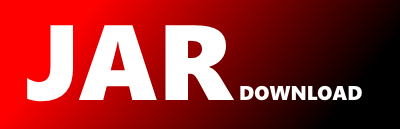
com.jelastic.api.system.persistence.Group Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 97d90589b8ea4a95422538c7de5bc74a*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.core.utils.DateUtils;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.text.ParseException;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Group extends ArrayItem implements Serializable {
public static final String IS_DELETED_FILTER = "isDeletedFilter";
public static final String IS_DELETED_FILTER_PARAM = "isDeleted";
public static final String NAME = "name";
public static final String ID = "id";
public static final String DESCRIPTION = "description";
public static final String IS_DEFAULT = "isDefault";
public static final String IS_SIGNUP = "isSignup";
public static final String IS_COLLABORATION = "isCollaboration";
public static final String QUOTAS_VALUES = "quotasValues";
public static final String TYPE = "type";
public static final String CREATED_ON = "createdOn";
public static final String UPDATED_ON = "updatedOn";
public static final String WIN_DOMAIN_ID = "winDomainId";
public static final String RESELLER = "reseller";
public static final String GROUP_COLUMN = "group";
public static final String CONVERSION_GROUP = "conversionGroup";
private GroupType type;
private String name;
private String description;
private Set quotasValues;
private boolean isDefault;
private boolean isSignup;
private boolean isCollaboration;
private Set groupPricingModels = new HashSet();
private Date createdOn;
private Date updatedOn;
private boolean isDeleted;
private Integer winDomainId;
private Reseller reseller;
private Group conversionGroup;
private String conversionGroupName;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GroupType getType() {
return type;
}
public void setType(GroupType type) {
this.type = type;
}
public Set getQuotasValues() {
return quotasValues;
}
public void setQuotasValues(Set quotasValues) {
this.quotasValues = quotasValues;
}
public boolean isDefault() {
return isDefault;
}
public void setDefault(boolean aDefault) {
this.isDefault = aDefault;
}
public boolean isSignup() {
return isSignup;
}
public void setSignup(boolean signup) {
this.isSignup = signup;
}
public boolean isCollaboration() {
return isCollaboration;
}
public void setCollaboration(boolean isCollaboration) {
this.isCollaboration = isCollaboration;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set getGroupPricingModels() {
return groupPricingModels;
}
public void setGroupPricingModels(Set groupPricingModels) {
this.groupPricingModels = groupPricingModels;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
this.isDeleted = deleted;
}
public Integer getWinDomainId() {
return winDomainId;
}
public void setWinDomainId(Integer winDomainId) {
this.winDomainId = winDomainId;
}
public Reseller getReseller() {
return reseller;
}
public void setReseller(Reseller reseller) {
this.reseller = reseller;
}
@Override
public Group _fromJSON(JSONObject json) throws JSONException {
if (json.has(TYPE)) {
this.type = GroupType.fromString(json.getString(TYPE));
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
if (json.has(IS_DEFAULT)) {
this.isDefault = json.getBoolean(IS_DEFAULT);
}
if (json.has(IS_SIGNUP)) {
this.isSignup = json.getBoolean(IS_SIGNUP);
}
if (json.has(IS_COLLABORATION)) {
this.isCollaboration = json.getBoolean(IS_COLLABORATION);
}
if (json.has(CREATED_ON)) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString(CREATED_ON));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has(UPDATED_ON)) {
try {
this.updatedOn = DateUtils.parseSqlDateTime(json.getString(UPDATED_ON));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has(WIN_DOMAIN_ID)) {
winDomainId = json.getInt(WIN_DOMAIN_ID);
}
if (json.has("reseller")) {
this.reseller = new Reseller()._fromJSON(json.getJSONObject("reseller"));
}
conversionGroupName = json.optString(CONVERSION_GROUP, null);
return this;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(TYPE, type.toString());
json.put(NAME, name);
json.put(DESCRIPTION, description);
json.put(IS_DEFAULT, isDefault);
json.put(IS_SIGNUP, isSignup);
json.put(IS_COLLABORATION, isCollaboration);
json.put(ID, id);
if (winDomainId != null) {
json.put(WIN_DOMAIN_ID, winDomainId);
}
if (this.createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(this.createdOn));
}
if (this.updatedOn != null) {
json.put(UPDATED_ON, DateUtils.formatSqlDateTime(this.updatedOn));
}
if (this.reseller != null) {
json.put("reseller", reseller._toJSON());
}
if (this.conversionGroup != null) {
json.put(CONVERSION_GROUP, this.conversionGroup.getName());
} else if (this.conversionGroupName != null) {
json.put(CONVERSION_GROUP, this.conversionGroupName);
}
return json;
}
public Group getConversionGroup() {
return conversionGroup;
}
public void setConversionGroup(Group conversionGroup) {
this.conversionGroup = conversionGroup;
}
@Override
public String toString() {
return "Group{" + "name='" + name + '\'' + ", reseller=" + reseller + ", id=" + id + '}';
}
}