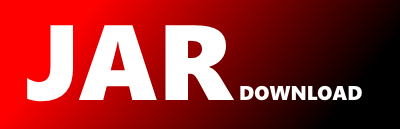
com.jelastic.api.system.persistence.GroupPricingModel Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 47627c70dd85c120d8220f2a1ececf83*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class GroupPricingModel extends ArrayItem {
public static final String ID = "id";
public static final String GROUP = "group";
public static final String PRICING_MODEL = "pricingModel";
public static final String HARD_NODE_GROUP = "hardNodeGroup";
public static final String IS_ENABLED = "isEnabled";
public static final String IS_DEFAULT = "isDefault";
private Group group;
private PricingModel pricingModel;
private String hardNodeGroup;
private boolean isEnabled;
private boolean isDefault;
public GroupPricingModel() {
}
public GroupPricingModel(Group group, PricingModel pricingModel, String hardNodeGroup, boolean isEnabled, boolean isDefault) {
this.group = group;
this.pricingModel = pricingModel;
this.hardNodeGroup = hardNodeGroup;
this.isEnabled = isEnabled;
this.isDefault = isDefault;
}
public GroupPricingModel(Group group, PricingModel pricingModel, String hardNodeGroup) {
this.group = group;
this.pricingModel = pricingModel;
this.hardNodeGroup = hardNodeGroup;
}
public GroupPricingModel clone(Group group) {
return new GroupPricingModel(group, pricingModel, hardNodeGroup, isEnabled, isDefault);
}
public GroupPricingModel clone() {
return new GroupPricingModel(group, pricingModel, hardNodeGroup, isEnabled, isDefault);
}
public Group getGroup() {
return group;
}
public void setGroup(Group group) {
this.group = group;
}
public PricingModel getPricingModel() {
return pricingModel;
}
public void setPricingModel(PricingModel pricingModel) {
this.pricingModel = pricingModel;
}
public String getHardNodeGroup() {
return hardNodeGroup;
}
public void setHardNodeGroup(String hardNodeGroup) {
this.hardNodeGroup = hardNodeGroup;
}
public boolean isEnabled() {
return isEnabled;
}
public void setEnabled(boolean isEnabled) {
this.isEnabled = isEnabled;
}
public boolean isDefault() {
return isDefault;
}
public void setDefault(boolean isDefault) {
this.isDefault = isDefault;
}
@Override
public GroupPricingModel _fromJSON(JSONObject json) throws JSONException {
if (json.has(GROUP)) {
if (json.get(GROUP) instanceof JSONObject) {
this.group = new Group()._fromJSON(json.getJSONObject(GROUP));
} else {
this.group = new Group();
this.group.setName(json.getString(GROUP));
}
}
if (json.has(HARD_NODE_GROUP)) {
this.hardNodeGroup = json.getString(HARD_NODE_GROUP);
}
if (json.has(IS_ENABLED)) {
this.isEnabled = json.getBoolean(IS_ENABLED);
}
if (json.has(IS_DEFAULT)) {
this.isDefault = json.getBoolean(IS_DEFAULT);
}
if (json.has(PRICING_MODEL)) {
this.pricingModel = new PricingModel()._fromJSON(json.getJSONObject(PRICING_MODEL));
}
return this;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(GROUP, group.getName());
json.put(PRICING_MODEL, pricingModel._toJSON());
json.put(HARD_NODE_GROUP, hardNodeGroup);
json.put(IS_ENABLED, isEnabled);
json.put(IS_DEFAULT, isDefault);
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy