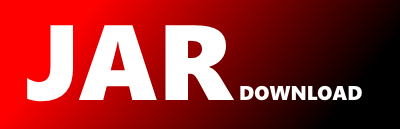
com.jelastic.api.system.persistence.HardwareNodeGroup Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 8cfbef1d2716e954d490991375db0160*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.common.annotation.Transform;
import com.jelastic.api.core.utils.JSONUtils;
import com.jelastic.api.data.HardwareNodeStatus;
import com.jelastic.api.data.po.VType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import com.jelastic.api.system.po.VzType;
import com.jelastic.api.system.engine.HostGroupEngine;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class HardwareNodeGroup extends ArrayItem implements Serializable {
public static final String HARDWARE_NODE_GROUP_COLUMN = "hardwareNodeGroup";
public static final String HOST_GROUP_COLUMN = "hostGroup";
public static final String DATA = "data";
public interface Add {
}
public interface Edit {
}
public static final String ID = "id";
public static final String UNIQUE_NAME = "uniqueName";
public static final String DISPLAY_NAME = "displayName";
public static final String DESCRIPTION = "description";
public static final String STATUS = "status";
public static final String DOMAIN = "domain";
public static final String OS_TYPES = "osTypes";
public static final String IS_DEFAULT = "isDefault";
public static final String IS_ENABLED = "isEnabled";
public static final String REGION_UNIQUE_NAME = "regionUniqueName";
public static final String VZ_TYPE = "vzType";
public static final String VZ_TYPES = "vzTypes";
public static final String V_TYPES = "vTypes";
public static final String ENDPOINTS = "endpoints";
public static final String ENGINE = "engine";
public static final String L3_GROUP = "l3Group";
public static final String ICON_16_URL_KEY = "icon16Url";
public static final String ICON_32_URL_KEY = "icon32Url";
public static final String ICON_64_URL_KEY = "icon64Url";
public static final String ICONS_DATA = "iconsData";
public static final String METADATA = "metadata";
public static final String SHORT_DESCRIPTION = "shortDescription";
public static final String COMMENT = "comment";
private String uniqueName;
private String displayName;
private String description;
private HardwareNodeGroupStatus status;
private Region region;
private Set hardwareNodes = new HashSet();
private Date createdOn;
private Date deletedOn;
private HostGroupEngine engine = HostGroupEngine.PAAS;
private Set endpoints;
private boolean isDeleted;
private String data;
private String l3Group;
private String metadata;
private String iconsData;
private String shortDescription;
private String comment;
private Set osTypes = new HashSet();
@Transform(skip = true)
private String regionUniqueName;
@Transform(skip = true)
private Boolean isDefault;
@Transform(skip = true)
private Boolean isEnabled;
private Integer vzType;
private Set vTypes = new HashSet<>();
private Set vzTypes = new HashSet<>();
public String getUniqueName() {
return uniqueName;
}
public void setUniqueName(String uniqueName) {
this.uniqueName = uniqueName;
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public HardwareNodeGroupStatus getStatus() {
return status;
}
public Region getRegion() {
return region;
}
public void setRegion(Region region) {
this.region = region;
}
public void setStatus(HardwareNodeGroupStatus status) {
this.status = status;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getDeletedOn() {
return deletedOn;
}
public void setDeletedOn(Date deletedOn) {
this.deletedOn = deletedOn;
}
public Set getOsTypes() {
return osTypes;
}
public void setOsTypes(Set osTypes) {
this.osTypes = osTypes;
}
public String getRegionUniqueName() {
return regionUniqueName;
}
public Boolean isDefault() {
return isDefault;
}
public void setDefault(Boolean isDefault) {
this.isDefault = isDefault;
}
public Set getHardwareNodes() {
return hardwareNodes;
}
public void setHardwareNodes(Set hardwareNodes) {
this.hardwareNodes = hardwareNodes;
}
public Boolean IsEnabled() {
return isEnabled;
}
public void setEnabled(Boolean isEnabled) {
this.isEnabled = isEnabled;
}
public Set getvTypes() {
this.vTypes.add(VType.CT);
HardwareNode bareMetal = hardwareNodes.stream().filter(hw -> hw.getHwType() == HWType.BARE_METAL).findAny().orElse(null);
if (bareMetal != null) {
this.vTypes.add(VType.VM);
}
return vTypes;
}
public Integer getVzType() {
if (!hardwareNodes.isEmpty()) {
return hardwareNodes.iterator().next().getVzType();
}
return null;
}
public Set getVzTypes() {
if (!hardwareNodes.isEmpty()) {
List vzTypes = hardwareNodes.stream().filter(h -> !h.equalsAnyStatus(HardwareNodeStatus.INFRASTRUCTURE_NODE, HardwareNodeStatus.BROKEN) && h.getOsType() != null && !h.getOsType().isWin()).map(HardwareNode::getVzType).collect(Collectors.toList());
if (vzTypes.size() == 0) {
return new HashSet<>();
}
int min = Collections.min(vzTypes);
return Collections.singleton(min);
}
return new HashSet<>();
}
public HostGroupEngine getEngine() {
return engine;
}
public void setEngine(HostGroupEngine hostGroupEngine) {
this.engine = hostGroupEngine;
}
public Set getEndpoints() {
return endpoints;
}
public void setEndpoints(Set endpoints) {
this.endpoints = endpoints;
}
public HostGroupEndpoint getEndpoint(HostGroupEndpointType type) {
for (HostGroupEndpoint endpoint : this.getEndpoints()) {
if (endpoint.getType() == type) {
return endpoint;
}
}
return null;
}
public String getL3Group() {
return l3Group;
}
public void setL3Group(String l3Group) {
this.l3Group = l3Group;
}
public Map getIconsDataMap() {
return JSONUtils.toMap(iconsData);
}
public String getIconsData() {
return iconsData;
}
public void setIconsData(String iconsData) {
this.iconsData = iconsData;
}
public void setComment(String comment) {
this.comment = comment;
}
public String getComment() {
return comment;
}
public void setShortDescription(String shortDescription) {
this.shortDescription = shortDescription;
}
public String getShortDescription() {
return shortDescription;
}
public void setMetadata(String metadata) {
this.metadata = metadata;
}
public String getMetadata() {
return metadata;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(UNIQUE_NAME, uniqueName);
json.put(DISPLAY_NAME, displayName);
json.put(DESCRIPTION, description);
json.put(STATUS, status);
if (region != null) {
json.put(REGION_UNIQUE_NAME, region.getUniqueName());
}
for (OSType osType : osTypes) {
JSONArray osTypesJsonArray = new JSONArray();
osTypesJsonArray.put(osType);
json.put(OS_TYPES, osTypesJsonArray);
}
json.put(IS_DEFAULT, isDefault);
json.put(IS_ENABLED, isEnabled);
json.put(ENGINE, this.engine.toString());
Set minVzType = getVzTypes();
Integer vzType = minVzType.isEmpty() ? null : minVzType.iterator().next();
if (vzType != null && VzType.isVz7(vzType)) {
json.put(VZ_TYPE, vzType);
} else if (this.vzType != null) {
json.put(VZ_TYPE, this.vzType);
}
Set vzTypes = getVzTypes();
if (!vzTypes.isEmpty()) {
json.put(VZ_TYPES, vzTypes);
} else {
json.put(VZ_TYPES, this.vzTypes);
}
Set types = getvTypes();
JSONArray typesArray = new JSONArray();
for (VType type : types) {
typesArray.put(type.toString());
}
json.put(V_TYPES, typesArray);
if (endpoints != null) {
JSONArray endpointsArray = new JSONArray();
for (HostGroupEndpoint endpoint : endpoints) {
endpointsArray.put(endpoint._toJSON());
}
json.put(ENDPOINTS, endpointsArray);
}
if (!StringUtils.isEmpty(this.data)) {
json.put(DATA, new JSONObject(this.data));
}
if (StringUtils.isNotBlank(this.l3Group)) {
json.put(L3_GROUP, this.l3Group);
} else if (region != null) {
json.put(L3_GROUP, this.region.getUniqueName());
}
if (StringUtils.isNotBlank(iconsData)) {
json.put(ICONS_DATA, new JSONObject(iconsData));
}
if (StringUtils.isNotBlank(metadata)) {
json.put(METADATA, new JSONObject(metadata));
}
if (StringUtils.isNotBlank(shortDescription)) {
json.put(SHORT_DESCRIPTION, shortDescription);
}
if (StringUtils.isNotBlank(comment)) {
json.put(COMMENT, comment);
}
return json;
}
@Override
public HardwareNodeGroup _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(UNIQUE_NAME)) {
this.uniqueName = json.getString(UNIQUE_NAME).toLowerCase();
}
if (json.has(DISPLAY_NAME)) {
this.displayName = json.getString(DISPLAY_NAME);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
if (json.has(STATUS)) {
this.status = HardwareNodeGroupStatus.valueOf(HardwareNodeGroupStatus.class, json.getString(STATUS));
}
if (json.has(ENGINE)) {
this.engine = HostGroupEngine.valueOf(json.getString(ENGINE));
}
if (json.has(OS_TYPES)) {
this.osTypes.clear();
JSONArray osTypesJsonArray = json.getJSONArray(OS_TYPES);
for (int i = 0; i < osTypesJsonArray.length(); i++) {
this.osTypes.add(OSType.valueOf(OSType.class, osTypesJsonArray.getString(i)));
}
}
if (json.has(REGION_UNIQUE_NAME)) {
this.regionUniqueName = json.getString(REGION_UNIQUE_NAME);
}
if (json.has(IS_DEFAULT)) {
this.isDefault = json.getBoolean(IS_DEFAULT);
}
if (json.has(IS_ENABLED)) {
this.isEnabled = json.getBoolean(IS_ENABLED);
}
if (json.has(VZ_TYPE)) {
this.vzType = json.getInt(VZ_TYPE);
}
if (json.has(VZ_TYPES)) {
this.vzTypes.clear();
JSONArray vzTypesJsonArray = json.getJSONArray(VZ_TYPES);
for (int i = 0; i < vzTypesJsonArray.length(); i++) {
this.vzTypes.add(vzTypesJsonArray.getInt(i));
}
}
if (json.has(V_TYPES)) {
this.vTypes.clear();
JSONArray vTypesJsonArray = json.getJSONArray(V_TYPES);
for (int i = 0; i < vTypesJsonArray.length(); i++) {
this.vTypes.add(VType.valueOf(vTypesJsonArray.getString(i)));
}
}
if (json.has(ENDPOINTS)) {
this.endpoints = new HashSet<>();
JSONArray endpointsArray = json.getJSONArray(ENDPOINTS);
for (int i = 0; i < endpointsArray.length(); i++) {
this.endpoints.add((HostGroupEndpoint) new HostGroupEndpoint()._fromJSON(endpointsArray.getJSONObject(i)));
}
}
if (json.has(DATA)) {
this.data = json.getString(DATA);
}
if (json.has(L3_GROUP)) {
String l3group = json.getString(L3_GROUP);
if (StringUtils.isNotBlank(l3group)) {
this.l3Group = l3group;
}
}
if (json.has(ICONS_DATA)) {
JSONObject jsonObject = json.getJSONObject(ICONS_DATA);
JSONObject iconDataJsonObject = new JSONObject();
if (jsonObject.has(ICON_16_URL_KEY)) {
iconDataJsonObject.put(ICON_16_URL_KEY, jsonObject.getString(ICON_16_URL_KEY));
}
if (jsonObject.has(ICON_32_URL_KEY)) {
iconDataJsonObject.put(ICON_32_URL_KEY, jsonObject.getString(ICON_32_URL_KEY));
}
if (jsonObject.has(ICON_64_URL_KEY)) {
iconDataJsonObject.put(ICON_64_URL_KEY, jsonObject.getString(ICON_64_URL_KEY));
}
this.iconsData = iconDataJsonObject.toString();
}
if (json.has(METADATA)) {
this.metadata = json.getJSONObject(METADATA).toString();
}
if (json.has(COMMENT)) {
this.comment = json.getString(COMMENT);
}
if (json.has(SHORT_DESCRIPTION)) {
this.shortDescription = json.getString(SHORT_DESCRIPTION);
}
return this;
}
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy