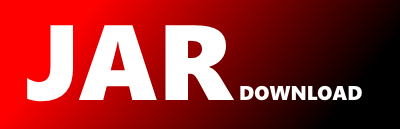
com.jelastic.api.system.persistence.IpAddress Maven / Gradle / Ivy
The newest version!
/*Server class MD5: b638752c03f7a12429a20196d41dd9e1*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import java.text.ParseException;
import java.util.Date;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class IpAddress extends ArrayItem {
public static final String ID = "id";
public static final String UPDATED_ON = "updatedOn";
public static final String IP_ADDRESS = "ipAddress";
private String ipAddress;
private boolean isBusy;
private Region region;
private String regionUniqueName;
private String domain;
private String appid;
private Date updatedOn = new Date();
public IpAddress() {
}
public IpAddress(String ipAddress, Region region) {
this.ipAddress = ipAddress;
this.region = region;
}
public IpAddress(String ipAddress, boolean isBusy, Region region) {
this.ipAddress = ipAddress;
this.isBusy = isBusy;
this.region = region;
}
public boolean isBusy() {
return isBusy;
}
public void setBusy(boolean isBusy) {
this.isBusy = isBusy;
}
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
public Region getRegion() {
return region;
}
public void setRegion(Region region) {
this.region = region;
}
public String getRegionUniqueName() {
return regionUniqueName;
}
public void setRegionUniqueName(String regionUniqueName) {
this.regionUniqueName = regionUniqueName;
}
public String getKey(String prefix) {
String key = this.ipAddress;
if (prefix != null) {
key = prefix + key;
}
return key;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("id", id);
json.put("isBusy", isBusy);
json.put("ipAddress", ipAddress);
if (region != null) {
json.put("region", region.getUniqueName());
}
json.put("domain", domain);
json.put("appid", appid);
json.put(UPDATED_ON, updatedOn);
return json;
}
@Override
public IpAddress _fromJSON(JSONObject json) throws JSONException {
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has("isBusy")) {
this.isBusy = json.getBoolean("isBusy");
}
if (json.has("ipAddress")) {
this.ipAddress = json.getString("ipAddress");
}
if (json.has("region")) {
this.regionUniqueName = json.getString("region");
}
if (json.has("domain")) {
this.domain = json.getString("domain");
}
if (json.has("appid")) {
this.appid = json.getString("appid");
}
if (json.has(UPDATED_ON)) {
try {
this.updatedOn = DateUtils.parseSqlDateTime(json.getString(UPDATED_ON));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
return this;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
@Override
public String toString() {
return "IpAddress{" + "ipAddress='" + ipAddress + '\'' + ", isBusy=" + isBusy + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy