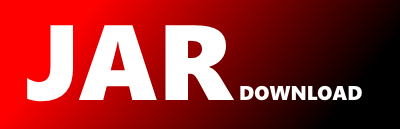
com.jelastic.api.system.persistence.Ipv6Subnet Maven / Gradle / Ivy
/*Server class MD5: 7895104a08edd891166ddd7bff2144e7*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.List;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Ipv6Subnet extends ArrayItem {
private AppNodes appNodes;
private int prefix;
private String network;
private boolean isBusy = false;
private List address;
private Network networkIpV6;
public Ipv6Subnet() {
}
public List getAddresses() {
return address;
}
public void setAddress(List address) {
this.address = address;
}
public Ipv6Subnet(AppNodes appNodes, int prefix, String network) {
this.appNodes = appNodes;
this.prefix = prefix;
this.network = network;
}
public AppNodes getAppNodes() {
return appNodes;
}
public void setAppNodes(AppNodes appNodes) {
this.appNodes = appNodes;
}
public int getPrefix() {
return prefix;
}
public void setPrefix(int prefix) {
this.prefix = prefix;
}
public String getNetwork() {
return network;
}
public void setNetwork(String network) {
this.network = network;
}
public boolean isBusy() {
return isBusy;
}
public void setBusy(boolean busy) {
isBusy = busy;
}
public String getNetworkString() {
return getNetwork() + "/" + getPrefix();
}
public Network getNetworkIpV6() {
return networkIpV6;
}
public void setNetworkIpV6(Network networkIpV6) {
this.networkIpV6 = networkIpV6;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("id", id);
json.put("subnet", network + "/" + prefix);
json.put("isBusy", isBusy);
if (appNodes != null) {
json.put("domain", appNodes.getDomain().getDomain());
json.put("appid", appNodes.getAppid());
json.put("region", appNodes.getHardwareNodeGroup().getRegion().getUniqueName());
}
if (!getAddresses().isEmpty()) {
json.put("region", getAddresses().get(0).getRegions().iterator().next().getUniqueName());
}
json.put("total", Math.pow(2, 128 - prefix));
long free = getFree();
json.put("free", free);
json.put("busy", getAddresses().size() - free);
return json;
}
public long getBusy() {
return getAddresses().size() - getFree();
}
public long getFree() {
long count = 0;
for (ExtIpAddress ip : getAddresses()) {
if (!ip.isBusy()) {
count++;
}
}
return count;
}
@Override
public String toString() {
return "Ipv6Subnet{" + "appNodes=" + appNodes + ", prefix=" + prefix + ", network='" + network + '\'' + ", isBusy=" + isBusy + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy