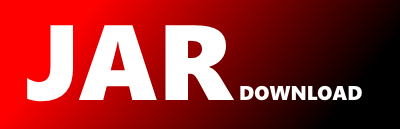
com.jelastic.api.system.persistence.Label Maven / Gradle / Ivy
The newest version!
/*Server class MD5: c077c2a82836608fa0ccfd0b27a0c9b4*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.Date;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Label extends ArrayItem implements Serializable {
private static final String NAME_REGEXP = "^[a-zA-Z0-9._\\-]+$";
private static final String VALUE_REGEXP = "^[a-zA-Z0-9._\\-]*$";
private static final int NAME_MAX_LENGTH = 63;
private static final int VALUE_MAX_LENGTH = 255;
public static final String ID = "id";
public static final String NAME = "name";
private static final String VALUE = "value";
private String name;
private String value;
private Date createdOn;
private HardwareNode hardwareNode;
public Label() {
}
public Label(Label label) {
this(label.getName(), label.getValue());
}
public Label(String name, String value) {
this.name = name;
this.value = StringUtils.stripToEmpty(value);
}
public HardwareNode getHardwareNode() {
return hardwareNode;
}
public void setHardwareNode(HardwareNode hardwareNode) {
this.hardwareNode = hardwareNode;
}
public String getName() {
return name;
}
public String getNameInLowerCase() {
return StringUtils.lowerCase(name);
}
public void setName(String name) {
this.name = StringUtils.lowerCase(name);
}
public String getValue() {
return value;
}
public String getValueInLowerCase() {
return StringUtils.lowerCase(value);
}
public void setValue(String value) {
this.value = StringUtils.stripToEmpty(value);
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NAME, name);
json.put(VALUE, value);
return json;
}
@Override
public Label _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(VALUE)) {
this.value = json.getString(VALUE);
}
return this;
}
public Response isValid() {
Response response = hasValidName(this.name);
if (response.isNotOK()) {
return response;
}
return hasValidValue();
}
public Response hasValidName() {
return hasValidName(this.name);
}
public Response hasValidValue() {
if (this.value != null && !this.value.matches(VALUE_REGEXP)) {
return new Response(Response.LABEL_VALUE_NOT_VALID, "Label's [" + name + "] value doesn't match the " + VALUE_REGEXP + " regex");
}
if (StringUtils.length(this.value) > VALUE_MAX_LENGTH) {
return new Response(Response.LABEL_VALUE_NOT_VALID, "Label's [" + name + "] value exceeds the max length of " + VALUE_MAX_LENGTH);
}
return Response.OK();
}
private static Response hasValidName(String name) {
if (StringUtils.isEmpty(name)) {
return new Response(Response.LABEL_NAME_NOT_VALID, "Label's name cannot be empty");
}
if (!name.matches(NAME_REGEXP)) {
return new Response(Response.LABEL_NAME_NOT_VALID, "Label's [" + name + "] name doesn't match the " + NAME_REGEXP + " regex");
}
if (name.length() > NAME_MAX_LENGTH) {
return new Response(Response.LABEL_NAME_NOT_VALID, "Label's [" + name + "] name exceeds the max length of " + NAME_MAX_LENGTH);
}
return Response.OK();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy