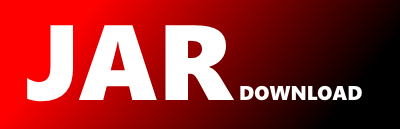
com.jelastic.api.system.persistence.Network Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 006df732d8aec756a4a96050c42d4e09*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.List;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Network extends ArrayItem {
public static final String NETWORK_COLUMN = "networkIpV6";
private String network;
private List subnets;
private Region region;
public Network() {
}
public Network(String mask) {
this.network = mask;
}
public String getNetwork() {
return network;
}
public void setNetwork(String network) {
this.network = network;
}
public List getSubnets() {
return subnets;
}
public Ipv6Subnet getFirstFreeSubnet() {
for (Ipv6Subnet s : getSubnets()) {
if (!s.isBusy() && s.getAddresses().stream().noneMatch(ExtIpAddress::isBusy)) {
return s;
}
}
return null;
}
public Ipv6Subnet getLastAllocatedSubnet() {
if (subnets == null || subnets.isEmpty()) {
return null;
}
Ipv6Subnet max = subnets.get(0);
for (Ipv6Subnet s : getSubnets()) {
if (s.getId() > max.getId()) {
max = s;
}
}
return max;
}
public void setSubnets(List subnets) {
this.subnets = subnets;
}
public Region getRegion() {
return region;
}
public void setRegion(Region region) {
this.region = region;
}
private int getPrefix() {
String[] array = network.split("/");
if (array.length != 2) {
return -1;
}
return Integer.parseInt(array[1]);
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("id", id);
json.put("network", network);
long total = getTotal();
json.put("total", total);
if (subnets.isEmpty()) {
json.put("free", total);
json.put("busy", 0);
} else {
json.put("allocated", subnets.size());
long busy = getBusy();
json.put("free", subnets.size() - busy);
json.put("busy", busy);
}
if (region != null) {
json.put("region", region.getUniqueName());
}
return json;
}
private long getBusy() {
long count = 0;
for (Ipv6Subnet s : getSubnets()) {
if (s.isBusy()) {
count++;
}
}
return count;
}
public long getTotal() {
int lastSubnetPrefix = 128;
if (!subnets.isEmpty()) {
Ipv6Subnet subnet = subnets.get(subnets.size() - 1);
lastSubnetPrefix = subnet.getPrefix();
}
return (long) Math.pow(2, lastSubnetPrefix - getPrefix());
}
public long getFree() {
return getTotal() - getBusy();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy