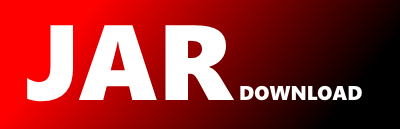
com.jelastic.api.system.persistence.PricingModel Maven / Gradle / Ivy
The newest version!
/*Server class MD5: affacd0cfeabcbd9f818a3b70d172a54*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.Response;
import com.jelastic.api.billing.response.ArrayResponse;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.annotation.Transform;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.system.persistence.pricing.TariffGrid;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.text.ParseException;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class PricingModel extends ArrayItem implements Serializable {
public static final String ID = "id";
public static final String UNIQUE_NAME = "uniqueName";
public static final String CREATED_ON = "createdOn";
public static final String UPDATED_ON = "updatedOn";
public static final String TYPE = "type";
public static final String NAME = "name";
public static final String DESCRIPTION = "description";
public static final String TARIFF_PLANS = "tariffPlans";
public static final String TARIFF_GRIDS = "tariffGrids";
public static final String TARIFFS = "tariffs";
public static final String RESELLER = "reseller";
private String uniqueName;
@Transform(required = true)
private String name;
@Transform(required = true)
private String description;
@Transform(required = true)
private PricingModelType type;
@Transform(required = true)
private Set tariffs;
private Map> groupLicTariffs = new HashMap<>();
private Map> plansByGridItemName = new HashMap<>();
@Transform(required = true)
private Set tariffGrids = new HashSet<>();
private Date createdOn;
private Date updatedOn;
private boolean isDeleted;
private Reseller reseller;
public String getUniqueName() {
return uniqueName;
}
public void setUniqueName(String uniqueName) {
this.uniqueName = uniqueName;
}
public PricingModelType getType() {
return type;
}
public void setType(PricingModelType type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Set getTariffPlans() {
return tariffs;
}
public void setTariffPlans(Set tariffPlans) {
this.tariffs = tariffPlans;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public Reseller getReseller() {
return reseller;
}
public void setReseller(Reseller reseller) {
this.reseller = reseller;
}
public Set getTariffGrids() {
return tariffGrids;
}
public void setTariffGrids(Set tariffGrids) {
this.tariffGrids = tariffGrids;
}
@Override
public PricingModel clone() {
PricingModel pricingModel = new PricingModel();
pricingModel.setUniqueName(this.uniqueName);
pricingModel.setType(this.type);
pricingModel.setName(this.name);
pricingModel.setCreatedOn(new Date());
pricingModel.setDescription(this.description);
pricingModel.setTariffPlans(this.tariffs);
pricingModel.setReseller(this.reseller);
return pricingModel;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
final PricingModel other = (PricingModel) obj;
if (this.getId() != other.getId()) {
return false;
}
return true;
}
@Override
public int hashCode() {
return id;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, this.getId());
json.put(UNIQUE_NAME, uniqueName);
json.put(TYPE, this.type);
json.put(NAME, this.name);
json.put(DESCRIPTION, this.description);
if (tariffs != null && !tariffs.isEmpty()) {
JSONArray jsonArray = new JSONArray();
for (TariffPlan tariffPlan : tariffs) {
jsonArray.put(tariffPlan._toJSON());
}
json.put(TARIFF_PLANS, jsonArray);
json.put(TARIFFS, jsonArray);
}
if (tariffGrids != null && !tariffGrids.isEmpty()) {
JSONArray jsonArray = new JSONArray();
for (TariffGrid grid : tariffGrids) {
jsonArray.put(grid._toJSON());
}
json.put(TARIFF_GRIDS, jsonArray);
}
if (this.createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(this.createdOn));
}
if (this.updatedOn != null) {
json.put(UPDATED_ON, DateUtils.formatSqlDateTime(this.updatedOn));
}
if (this.reseller != null) {
json.put(RESELLER, reseller._toJSON());
}
return json;
}
@Override
public PricingModel _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(UNIQUE_NAME)) {
this.uniqueName = json.getString(UNIQUE_NAME);
}
if (json.has(TYPE)) {
this.type = PricingModelType.valueOf(json.getString(TYPE).toUpperCase());
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
if (json.has(TARIFF_PLANS)) {
initTariffsFromJSON(TARIFF_PLANS, json);
} else if (json.has(TARIFFS)) {
initTariffsFromJSON(TARIFFS, json);
}
if (json.has(TARIFF_GRIDS)) {
tariffGrids = new HashSet<>();
JSONArray jsonArray = json.getJSONArray(TARIFF_GRIDS);
for (int i = 0; i < jsonArray.length(); i++) {
TariffGrid tariffGrid = (TariffGrid) new TariffGrid()._fromJSON(jsonArray.getJSONObject(i));
tariffGrids.add(tariffGrid);
}
}
if (json.has(CREATED_ON)) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString(CREATED_ON));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has(UPDATED_ON)) {
try {
this.updatedOn = DateUtils.parseSqlDateTime(json.getString(UPDATED_ON));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has(RESELLER)) {
this.reseller = new Reseller()._fromJSON(json.getJSONObject(RESELLER));
}
return this;
}
public Map> getGroupLicTariffs() {
return groupLicTariffs;
}
public List getListLicTariffs() {
return groupLicTariffs.values().stream().flatMap(Collection::stream).collect(Collectors.toList());
}
public void addUniqueResourcesFromGridItemsList(List tariffResources) {
for (Set plans : plansByGridItemName.values()) {
for (TariffPlan plan : plans) {
if (plan.getResource() != null && !tariffResources.contains(plan.getResource())) {
tariffResources.add(plan.getResource());
}
}
}
}
public Map> getPlansByGridItemName() {
return plansByGridItemName;
}
public void setPlansByGridItemName(Map> plansByGridItemName) {
this.plansByGridItemName = plansByGridItemName;
}
public void setGroupLicTariffs(Map> groupLicTariffs) {
this.groupLicTariffs = groupLicTariffs;
}
private void initTariffsFromJSON(String name, JSONObject json) throws JSONException {
JSONArray jsonArray = json.getJSONArray(name);
tariffs = new HashSet();
for (int i = 0; i < jsonArray.length(); i++) {
TariffPlan tariffPlan = new TariffPlan()._fromJSON(jsonArray.getJSONObject(i));
tariffs.add(tariffPlan);
}
}
public Response validateFields() {
if (this.getType() == null || this.getName() == null || this.getName().isEmpty()) {
return new ArrayResponse(Response.INVALID_PARAM, "Wrong name or type of the pricing model.");
}
return Response.OK();
}
public boolean addTariffGridByName(TariffGrid grid) {
TariffGrid existedGrid = tariffGrids.stream().filter(tariffGrid -> tariffGrid.getName().equals(grid.getName())).findAny().orElse(null);
if (existedGrid == null) {
tariffGrids.add(grid);
return true;
} else {
return false;
}
}
public boolean removeTariffGridByName(TariffGrid grid) {
TariffGrid existedGrid = tariffGrids.stream().filter(tariffGrid -> tariffGrid.getName().equals(grid.getName())).findAny().orElse(null);
if (existedGrid != null) {
tariffGrids.remove(grid);
return true;
} else {
return false;
}
}
@Override
public String toString() {
return "PricingModel{" + "uniqueName='" + uniqueName + '\'' + ", name='" + name + '\'' + ", isDeleted=" + isDeleted + ", reseller=" + reseller + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy