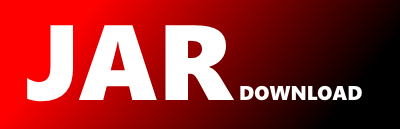
com.jelastic.api.system.persistence.Quota Maven / Gradle / Ivy
/*Server class MD5: 346f5c475c4e37e89ce5d1df34100086*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Quota extends ArrayItem implements Serializable {
public static final String ID = "id";
public static final String NAME = "name";
public static final String DESCRIPTION = "description";
public static final String IS_BUSINESS = "isBusiness";
public static final String IS_HIDDEN = "isHidden";
public static final String REFERENCE_ID = "referenceId";
public static final String IS_CUSTOM = "isCustom";
private String name;
private String description;
private Boolean isBusiness = true;
private Boolean isHidden = false;
private Boolean isCustom = false;
private String referenceId;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Boolean isBusiness() {
return isBusiness;
}
public void setBusiness(Boolean business) {
isBusiness = business;
}
public Boolean isHidden() {
return isHidden;
}
public void setHidden(Boolean hidden) {
isHidden = hidden;
}
public String getReferenceId() {
return referenceId;
}
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
public Boolean isCustom() {
return isCustom;
}
public void setCustom(Boolean custom) {
isCustom = custom;
}
@Override
public Quota _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
if (json.has(IS_HIDDEN)) {
this.isHidden = json.getBoolean(IS_HIDDEN);
}
if (json.has(IS_CUSTOM)) {
this.isCustom = json.getBoolean(IS_CUSTOM);
}
if (json.has(REFERENCE_ID)) {
this.referenceId = json.getString(REFERENCE_ID);
}
return this;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NAME, name);
json.put(DESCRIPTION, description);
json.put(IS_HIDDEN, isHidden);
json.put(IS_CUSTOM, isCustom);
if (this.referenceId != null) {
json.put(REFERENCE_ID, referenceId);
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy