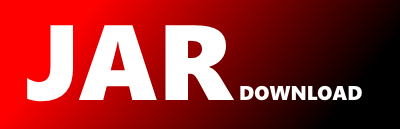
com.jelastic.api.system.persistence.Region Maven / Gradle / Ivy
The newest version!
/*Server class MD5: cafaf55d90a5b69b9669362e9583c572*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.EnumSet;
import java.util.Date;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
import java.util.stream.Collectors;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Region extends ArrayItem implements Serializable, Cloneable {
public static final String REGION_COLUMN = "region";
public interface Add {
}
public interface Edit {
}
public static final String IP_RANGE_SEPARATOR = "-";
public static final String ID = "id";
public static final String UNIQUE_NAME = "uniqueName";
public static final String DISPLAY_NAME = "displayName";
public static final String COMMENT = "comment";
public static final String STATUS = "status";
public static final String SUBNET = "subnet";
public static final String DOMAIN = "domain";
public static final String IS_DEFAULT = "isDefault";
public static final String HARDWARE_NODE_GROUPS = "hardNodeGroups";
public static final String IS_MIGRATION_ALLOWED = "isMigrationAllowed";
public static final String IP_RANGE = "ipRange";
public static final String SSL_ENABLED = "sslEnabled";
public static final String SSL_EXPIRE_DATE = "sslExpireDate";
public static final String SSL_TYPE = "sslType";
public static final String DOCKER_HOST = "dockerHost";
public static final String DOCKER_SSH_PORT = "dockerSSHPort";
public static final String DOCKER_LOGIN = "dockerLogin";
public static final String DOCKER_PASSWORD = "dockerPassword";
public static final String DOCKER_TCP_PORT = "dockerTCPPort";
private static final String DOCKER_SSH_KEY = "dockerSshKey";
private static final String CONNECTED = "connected";
public static final String STUBNETS = "stubnets";
public static final String OSPF_AREA = "ospfArea";
public static final String OSPF_KEY = "ospfKey";
public static final String CROSS_NETWORK_DEDICATED_SUBNET = "crossNetworkDedicatedSubnet";
public static final String CROSS_NETWORK_CONTAINERS_PREFIX = "crossNetworkContainersPrefix";
public static final String IS_CROSS_NETWORK_ENABLED = "isCrossNetworkEnabled";
private String uniqueName;
private String displayName;
private String ipRange;
private RegionStatus status;
private String subnet;
private String crossNetworkDedicatedSubnet;
private Integer crossNetworkContainersPrefix;
private boolean isCrossNetworkEnabled;
private String domain = "";
private Date createdOn;
private Date deletedOn;
private boolean isDefault;
private boolean isMigrationAllowed;
private boolean isDeleted;
private String comment;
private String dockerHost;
private Integer dockerSSHPort = 22;
private String dockerLogin = "root";
private String dockerPassword;
private Integer dockerTCPPort = 5555;
private String dockerSshKey;
private Set hardwareNodeGroups = new HashSet();
private String shortUniqueName;
private String stubnets;
private String ospfKey;
private Integer ospfArea;
private Set domains = new HashSet<>();
private boolean sslEnabled;
private boolean connected = false;
private Date sslExpireDate;
private SslType sslType;
public Region() {
}
public Region(int id, String uniqueName, String displayName, String ipRange, RegionStatus status, String subnet, String domain, Date createdOn, Date deletedOn, boolean isDefault, boolean isMigrationAllowed, boolean isDeleted, String comment, Set hardwareNodeGroups, String dockerSshKey, String crossNetworkDedicatedSubnet, Integer crossNetworkContainersPrefix, boolean isCrossNetworkEnabled) {
this.id = id;
this.uniqueName = uniqueName;
this.displayName = displayName;
this.ipRange = ipRange;
this.status = status;
this.subnet = subnet;
this.domain = domain;
this.createdOn = createdOn;
this.deletedOn = deletedOn;
this.isDefault = isDefault;
this.isMigrationAllowed = isMigrationAllowed;
this.isDeleted = isDeleted;
this.comment = comment;
this.hardwareNodeGroups = hardwareNodeGroups;
this.dockerSshKey = dockerSshKey;
this.crossNetworkDedicatedSubnet = crossNetworkDedicatedSubnet;
this.crossNetworkContainersPrefix = crossNetworkContainersPrefix;
this.isCrossNetworkEnabled = isCrossNetworkEnabled;
}
public String getUniqueName() {
return uniqueName;
}
public void setUniqueName(String uniqueName) {
this.uniqueName = uniqueName;
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public RegionStatus getStatus() {
return status;
}
public void setStatus(RegionStatus status) {
this.status = status;
}
public String getSubnet() {
return subnet;
}
public void setSubnet(String subnet) {
this.subnet = subnet;
}
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain.toLowerCase();
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getDeletedOn() {
return deletedOn;
}
public void setDeletedOn(Date deletedOn) {
this.deletedOn = deletedOn;
}
public boolean isDefault() {
return isDefault;
}
public void setDefault(boolean isDefault) {
this.isDefault = isDefault;
}
public String getLowAddress() {
String[] ips = ipRange.split(IP_RANGE_SEPARATOR);
return ips[0];
}
public String getHighAddress() {
String[] ips = ipRange.split(IP_RANGE_SEPARATOR);
return ips[1];
}
public Set getHardwareNodeGroups() {
return hardwareNodeGroups;
}
public void setHardwareNodeGroups(Set hardwareNodeGroups) {
this.hardwareNodeGroups = hardwareNodeGroups;
}
public boolean isMigrationAllowed() {
return isMigrationAllowed;
}
public void setMigrationAllowed(boolean isMigrationAllowed) {
this.isMigrationAllowed = isMigrationAllowed;
}
public String getIpRange() {
return ipRange;
}
public void setIpRange(String ipRange) {
this.ipRange = ipRange;
}
public Date getSslExpireDate() {
return sslExpireDate;
}
public void setSslExpireDate(Date sslExpireDate) {
this.sslExpireDate = sslExpireDate;
}
public boolean isSslEnabled() {
return sslEnabled;
}
public void setSslEnabled(boolean sslEnabled) {
this.sslEnabled = sslEnabled;
}
public SslType getSslType() {
return sslType;
}
public void setSslType(SslType sslType) {
this.sslType = sslType;
}
public String getDockerHost() {
return dockerHost;
}
public void setDockerHost(String dockerHost) {
this.dockerHost = dockerHost;
}
public Integer getDockerSSHPort() {
return dockerSSHPort;
}
public void setDockerSSHPort(Integer dockerSSHPort) {
this.dockerSSHPort = dockerSSHPort;
}
public String getDockerLogin() {
return dockerLogin;
}
public void setDockerLogin(String dockerLogin) {
this.dockerLogin = dockerLogin;
}
public String getDockerPassword() {
return dockerPassword;
}
public void setDockerPassword(String dockerPassword) {
this.dockerPassword = dockerPassword;
}
public Integer getDockerTCPPort() {
return dockerTCPPort;
}
public void setDockerTCPPort(Integer dockerTCPPort) {
this.dockerTCPPort = dockerTCPPort;
}
public String getDockerSshKey() {
return dockerSshKey;
}
public void setDockerSshKey(String dockerSshKey) {
this.dockerSshKey = dockerSshKey;
}
public String getShortUniqueName() {
return shortUniqueName;
}
public void setShortUniqueName(String shortUniqueName) {
this.shortUniqueName = shortUniqueName;
}
public String getStubnets() {
return stubnets;
}
public void setStubnets(String stubnets) {
this.stubnets = stubnets;
}
public String getOspfKey() {
return ospfKey;
}
public void setOspfKey(String ospfKey) {
this.ospfKey = ospfKey;
}
public Integer getOspfArea() {
return ospfArea;
}
public void setOspfArea(Integer ospfArea) {
this.ospfArea = ospfArea;
}
public Set getDomains() {
return domains;
}
public Set getAllDomainNames() {
Set domainSet = new LinkedHashSet<>();
domainSet.add(domain);
domainSet.addAll(domains.stream().map(RegionDomain::getDomain).collect(Collectors.toSet()));
return domainSet;
}
public Set getAllActiveDomainNames() {
Set domainSet = new LinkedHashSet<>();
domainSet.add(domain);
domainSet.addAll(domains.stream().filter(Region::checkEligibleStatus).map(RegionDomain::getDomain).collect(Collectors.toSet()));
return domainSet;
}
private static boolean checkEligibleStatus(RegionDomain regionDomain) {
EnumSet eligibleStatus = EnumSet.of(RegionDomainStatus.ACTIVE, RegionDomainStatus.CONFIGURING, RegionDomainStatus.SET_PRIMARY_FAILED, RegionDomainStatus.CONFIGURING_FAILED);
return eligibleStatus.contains(regionDomain.getStatus());
}
public void setDomains(Set regionDomainHistories) {
this.domains = regionDomainHistories;
}
public boolean isCrossNetworkEnabled() {
return isCrossNetworkEnabled;
}
public void setCrossNetworkEnabled(boolean crossNetworkEnabled) {
isCrossNetworkEnabled = crossNetworkEnabled;
}
public void setCrossNetworkDedicatedSubnet(String crossNetworkDedicatedSubnet) {
this.crossNetworkDedicatedSubnet = crossNetworkDedicatedSubnet;
}
public String getCrossNetworkDedicatedSubnet() {
return crossNetworkDedicatedSubnet;
}
public void setCrossNetworkContainersPrefix(Integer crossNetworkContainersMask) {
this.crossNetworkContainersPrefix = crossNetworkContainersMask;
}
public Integer getCrossNetworkContainersPrefix() {
return crossNetworkContainersPrefix;
}
@Override
public int hashCode() {
return id;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(UNIQUE_NAME, uniqueName);
json.put(DISPLAY_NAME, displayName);
json.put(COMMENT, comment);
json.put(STATUS, status);
json.put(SUBNET, subnet);
json.put(DOMAIN, domain);
json.put(IS_DEFAULT, isDefault);
json.put(IS_MIGRATION_ALLOWED, isMigrationAllowed);
json.put(IP_RANGE, ipRange);
json.put(SSL_ENABLED, sslEnabled);
json.put(SSL_TYPE, sslType != null ? sslType.name() : SslType.CUSTOM.name());
json.put(CONNECTED, connected);
if (sslExpireDate != null) {
json.put(SSL_EXPIRE_DATE, DateUtils.formatSqlDateTime(sslExpireDate));
}
if (dockerHost != null) {
json.put(DOCKER_HOST, dockerHost);
}
if (dockerLogin != null) {
json.put(DOCKER_LOGIN, dockerLogin);
}
if (dockerSSHPort != null) {
json.put(DOCKER_SSH_PORT, dockerSSHPort);
}
if (dockerTCPPort != null) {
json.put(DOCKER_TCP_PORT, dockerTCPPort);
}
JSONArray hardwareNodeGroupsJsonArray = new JSONArray();
for (HardwareNodeGroup hardwareNodeGroup : hardwareNodeGroups) {
hardwareNodeGroupsJsonArray.put(hardwareNodeGroup._toJSON());
}
json.put(HARDWARE_NODE_GROUPS, hardwareNodeGroupsJsonArray);
if (stubnets != null) {
json.put(STUBNETS, stubnets);
}
if (ospfKey != null) {
json.put(OSPF_KEY, ospfKey);
}
if (ospfArea != null) {
json.put(OSPF_AREA, ospfArea);
}
if (crossNetworkDedicatedSubnet != null) {
json.put(CROSS_NETWORK_DEDICATED_SUBNET, crossNetworkDedicatedSubnet);
}
if (crossNetworkContainersPrefix != null) {
json.put(CROSS_NETWORK_CONTAINERS_PREFIX, crossNetworkContainersPrefix);
}
json.put(IS_CROSS_NETWORK_ENABLED, isCrossNetworkEnabled);
return json;
}
@Override
public Region _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(UNIQUE_NAME)) {
this.uniqueName = json.getString(UNIQUE_NAME).toLowerCase();
}
if (json.has(DISPLAY_NAME)) {
this.displayName = json.getString(DISPLAY_NAME);
}
if (json.has(COMMENT)) {
this.comment = json.getString(COMMENT);
}
if (json.has(STATUS)) {
this.status = RegionStatus.valueOf(RegionStatus.class, json.getString(STATUS));
}
if (json.has(SUBNET)) {
this.subnet = json.getString(SUBNET);
}
if (json.has(DOMAIN)) {
this.domain = json.getString(DOMAIN).toLowerCase();
}
if (json.has(IS_DEFAULT)) {
this.isDefault = json.getBoolean(IS_DEFAULT);
}
if (json.has(IS_MIGRATION_ALLOWED)) {
this.isMigrationAllowed = json.getBoolean(IS_MIGRATION_ALLOWED);
}
if (json.has(IP_RANGE)) {
this.ipRange = json.getString(IP_RANGE);
}
if (json.has(DOCKER_HOST)) {
this.dockerHost = json.getString(DOCKER_HOST);
}
if (json.has(DOCKER_LOGIN)) {
this.dockerLogin = json.getString(DOCKER_LOGIN);
}
if (json.has(DOCKER_PASSWORD)) {
this.dockerPassword = json.getString(DOCKER_PASSWORD);
}
if (json.has(DOCKER_SSH_PORT)) {
this.dockerSSHPort = json.getInt(DOCKER_SSH_PORT);
}
if (json.has(DOCKER_TCP_PORT)) {
this.dockerTCPPort = json.getInt(DOCKER_TCP_PORT);
}
if (json.has(HARDWARE_NODE_GROUPS)) {
JSONArray groups = json.getJSONArray(HARDWARE_NODE_GROUPS);
for (int i = 0; i < groups.length(); i++) {
hardwareNodeGroups.add(new HardwareNodeGroup()._fromJSON(groups.getJSONObject(i)));
}
}
if (json.has(DOCKER_SSH_KEY)) {
this.dockerSshKey = json.getString(DOCKER_SSH_KEY);
}
if (json.has(CONNECTED)) {
this.connected = json.getBoolean(CONNECTED);
}
if (json.has(STUBNETS)) {
this.stubnets = json.getString(STUBNETS);
}
if (json.has(OSPF_AREA)) {
this.ospfArea = json.getInt(OSPF_AREA);
}
if (json.has(OSPF_KEY)) {
this.ospfKey = json.getString(OSPF_KEY);
}
if (json.has(CROSS_NETWORK_DEDICATED_SUBNET)) {
this.crossNetworkDedicatedSubnet = json.getString(CROSS_NETWORK_DEDICATED_SUBNET);
}
if (json.has(CROSS_NETWORK_CONTAINERS_PREFIX)) {
this.crossNetworkContainersPrefix = json.getInt(CROSS_NETWORK_CONTAINERS_PREFIX);
}
if (json.has(IS_CROSS_NETWORK_ENABLED)) {
this.isCrossNetworkEnabled = json.getBoolean(IS_CROSS_NETWORK_ENABLED);
}
return this;
}
public String getKey(String prefix) {
String key = this.displayName;
if (prefix != null) {
key = prefix + key;
}
return key;
}
@Override
public String toString() {
try {
return this._toJSON().toString();
} catch (JSONException e) {
e.printStackTrace();
return super.toString();
}
}
public boolean isConnected() {
return connected;
}
public void setConnected(boolean connected) {
this.connected = connected;
}
@Override
public Region clone() {
return new Region(id, uniqueName, displayName, ipRange, status, subnet, domain, createdOn, deletedOn, isDefault, isMigrationAllowed, isDeleted, comment, hardwareNodeGroups, dockerSshKey, crossNetworkDedicatedSubnet, crossNetworkContainersPrefix, isCrossNetworkEnabled);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy