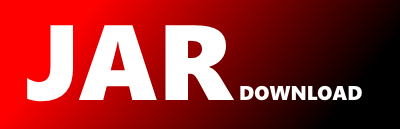
com.jelastic.api.system.persistence.Resource Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 0b2be3d1efb323359d4bfdd0ab5d9319*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.Objects;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Resource extends ArrayItem implements Serializable {
public static final String ID = "id";
public static final String NAME = "name";
public static final String DESCRIPTION = "description";
public static final String TYPE = "type";
public static final String UNIT = "unit";
public static final String IS_DELETED_FILTER = "isDeletedFilter";
public static final String IS_DELETED_FILTER_PARAM = "isDeleted";
String name;
private String description;
private boolean isDeleted;
private ResourceType type;
private ResourceUnit unit;
private boolean hidden = false;
private Reseller reseller;
public Resource() {
}
public Resource(String name, String description, ResourceType type) {
this.name = name;
this.description = description;
this.type = type;
}
public Resource(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public ResourceType getType() {
return type;
}
public void setType(ResourceType type) {
this.type = type;
}
public void setReseller(Reseller reseller) {
this.reseller = reseller;
}
public Reseller getReseller() {
return reseller;
}
public ResourceUnit getUnit() {
return unit;
}
public void setUnit(ResourceUnit unit) {
this.unit = unit;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NAME, name);
json.put(DESCRIPTION, description);
json.put(TYPE, type);
json.put(UNIT, unit);
return json;
}
@Override
public Resource _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.setName(json.getString(NAME));
}
if (json.has(TYPE)) {
this.type = ResourceType.valueOf(json.getString(TYPE).toUpperCase());
if (this.type == ResourceType.DEFAULT) {
this.type = ResourceType.CUSTOM;
}
}
if (json.has(DESCRIPTION)) {
this.setDescription(json.getString(DESCRIPTION));
}
if (json.has(UNIT)) {
this.unit = ResourceUnit.valueOf(json.getString(UNIT).toUpperCase());
}
return this;
}
@Override
public Resource clone() {
Resource resource = new Resource();
resource.name = this.name;
resource.description = this.description;
resource.type = this.type;
resource.reseller = this.reseller;
resource.unit = this.unit;
return resource;
}
protected Resource fullClone() {
Resource resource = this.clone();
resource.setId(this.getId());
return resource;
}
public Resource merge(Resource resource) {
this.setName(resource.getName());
this.setDescription(resource.description);
if (resource.getType() != null) {
this.setType(resource.type);
}
return this;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (!(o instanceof Resource))
return false;
if (!super.equals(o))
return false;
Resource resource = (Resource) o;
return isDeleted() == resource.isDeleted() && getName().equals(resource.getName()) && getReseller().equals(resource.getReseller());
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), getName(), isDeleted(), getType(), getReseller());
}
@Override
public String toString() {
return "Resource{" + "name='" + name + '\'' + ", type=" + type + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy