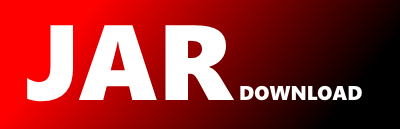
com.jelastic.api.system.persistence.SoftNodeGroup Maven / Gradle / Ivy
/*Server class MD5: 5932b83b8d9a470cfb4e6054ecc112ee*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.data.po.VType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import java.text.MessageFormat;
import java.util.stream.Collectors;
import org.json.*;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SoftNodeGroup extends ArrayItem {
public static final int PROPERTY_MAX_COUNT = 64;
public static final int PROPERTY_NAME_MAX_LENGTH = 64;
public static final int PROPERTY_VALUE_MAX_LENGTH = 8192;
public static final int DISPLAY_NAME_MAX_LENGTH = 255;
public static final int NODE_GROUP_NAME_LENGTH = 255;
public static final String NODE_GROUP_NAME_REGEXP = "^[a-z0-9._\\-+]+$";
public static final int GROUP_OPERATION_DELAY_MAX_VALUE = 5 * 60;
public static final int GROUP_OPERATION_DELAY_MIN_VALUE = -1;
public static final String SOFT_NODE_GROUP_COLUMN = "softNodeGroup";
public static final String ID = "id";
public static final String NAME = "name";
public static final String TARIFF_OPTIONS = "options";
public static final String REDEPLOY_CONTAINER_DELAY = "redeployContainerDelay";
public static final String RESTART_NODE_DELAY = "restartNodeDelay";
public static final String RESTART_CONTAINER_DELAY = "restartContainerDelay";
public static final String REDEPLOY_CONTEXT_DELAY = "redeployContextDelay";
public static final String TEMPLATE_TYPE = "templateType";
public static final String ENGINE_TYPE = "engineType";
public static final String DEPLOYMENTS = "deployments";
public static final String DISPLAY_NAME = "displayName";
public static final String IS_SEQUENTIAL_DEPLOY = "isSequentialDeploy";
public static final String FEATURES = "features";
public static final String FIREWALL_RULES = "firewallRules";
public static final String PROPERTIES = "properties";
public static final String SCALING_MODE = "scalingMode";
public static final String CLUSTER_PROPERTY = "cluster";
public static final String SKIP_NODE_EMAILS_PROPERTY = "skipNodeEmails";
public static final String CLUSTER_SUPPORT_PROPERTY = "isClusterSupport";
public static final String REDEPLOY_SUPPORT_PROPERTY = "isRedeploySupport";
public static final String ADMIN_URL_PROPERTY = "adminUrl";
public static final String DEPLOY_SUPPORT_PROPERTY = "isDeploySupport";
public static final String DISTRIBUTION_PROPERTY = "distribution";
public static final String BILLING_OPTIONS = "billingOptions";
public static final String IS_SLB_ACCESS_ENABLED = "isSLBAccessEnabled";
private String name;
private Set softwareNodes = new HashSet<>();
private Integer redeployContainerDelay;
private Integer restartNodeDelay;
private Integer restartContainerDelay;
private Integer redeployContextDelay;
private boolean isSequentialDeploy;
private TemplateType templateType;
private Set contexts = new HashSet<>();
private String engineType;
private Set rules = new HashSet<>();
private String displayName;
private Set properties = new HashSet<>();
private ScalingMode scalingMode;
private Set subdomains = new HashSet<>();
private boolean isSLBAccessEnabled = true;
private Map propertiesMap = new HashMap<>();
private VType vType;
public SoftNodeGroup() {
}
public SoftNodeGroup(String name, int redeployContainerDelay, int restartNodeDelay, int restartContainerDelay, int redeployContextDelay) {
this.name = name;
this.redeployContainerDelay = redeployContainerDelay;
this.restartNodeDelay = restartNodeDelay;
this.restartContainerDelay = restartContainerDelay;
this.redeployContextDelay = redeployContextDelay;
}
public static SoftNodeGroup createSoftNodeGroupByDefault(String groupName) {
return new SoftNodeGroup(groupName, 30, 0, 0, 30);
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set getSoftwareNodes() {
return softwareNodes;
}
public void setSoftwareNodes(Set softwareNodes) {
this.softwareNodes = softwareNodes;
}
public int getRedeployContainerDelay() {
return redeployContainerDelay;
}
public void setRedeployContainerDelay(int redeployContainerDelay) {
this.redeployContainerDelay = normalizeDelay(redeployContainerDelay);
}
public int getRestartNodeDelay() {
return restartNodeDelay;
}
public void setRestartNodeDelay(int restartNodeDelay) {
this.restartNodeDelay = normalizeDelay(restartNodeDelay);
}
public int getRestartContainerDelay() {
return restartContainerDelay;
}
public void setRestartContainerDelay(int restartContainerDelay) {
this.restartContainerDelay = normalizeDelay(restartContainerDelay);
}
public int getRedeployContextDelay() {
return redeployContextDelay;
}
public void setRedeployContextDelay(int redeployContextDelay) {
this.redeployContextDelay = normalizeDelay(redeployContextDelay);
}
public TemplateType getTemplateType() {
return templateType;
}
public void setTemplateType(TemplateType templateType) {
this.templateType = templateType;
}
public String getEngineType() {
return engineType;
}
public void setEngineType(String engineType) {
this.engineType = engineType;
}
public Set getContexts() {
return contexts;
}
public void setContexts(Set contexts) {
this.contexts = contexts;
}
public boolean getSequentialDeploy() {
return isSequentialDeploy;
}
public void setSequentialDeploy(Boolean sequentialDeploy) {
isSequentialDeploy = sequentialDeploy == null || sequentialDeploy;
}
public boolean isSLBAccessEnabled() {
return isSLBAccessEnabled;
}
public void setSLBAccessEnabled(boolean isSLBAccessEnabled) {
this.isSLBAccessEnabled = isSLBAccessEnabled;
}
public Set getActiveRules() {
return rules.stream().filter((p) -> !p.isDeleted()).collect(Collectors.toSet());
}
public Set getRules() {
return rules;
}
public Set getRulesByDirection(FirewallRuleDirection direction) {
if (direction == null) {
return getActiveRules();
}
Set ruleSet = new HashSet<>();
for (FirewallRule rule : getActiveRules()) {
if (rule.getDirection() == direction) {
ruleSet.add(rule);
}
}
return ruleSet;
}
public FirewallRule getEqualFirewallRule(FirewallRule firewallRule) {
for (FirewallRule rule : getActiveRules()) {
if (rule.compare(firewallRule)) {
return rule;
}
}
return null;
}
public void setRules(Set rules) {
this.rules = rules;
}
public boolean removeRule(FirewallRule rule) {
return this.getRules().remove(rule);
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public ScalingMode getScalingMode() {
return scalingMode;
}
public void setScalingMode(ScalingMode scalingMode) {
this.scalingMode = scalingMode;
}
public void applyData(JSONObject data) throws JSONException {
setProperties(data, true);
setCustomProperties(data);
}
public void setBillingOptions(JSONArray data) {
SoftNodeGroupProperty licOptionsProperty = getPropertyByName(BILLING_OPTIONS);
if (licOptionsProperty == null) {
licOptionsProperty = new SoftNodeGroupProperty(BILLING_OPTIONS, data.toString(), SoftNodeGroupPropertyType.JSON_ARRAY);
} else {
properties.remove(licOptionsProperty);
licOptionsProperty.setValue(data.toString());
}
properties.add(licOptionsProperty);
}
public void setStringBillingOptions(String data) {
SoftNodeGroupProperty licOptionsProperty = getPropertyByName(BILLING_OPTIONS);
if (licOptionsProperty == null) {
licOptionsProperty = new SoftNodeGroupProperty(BILLING_OPTIONS, data, SoftNodeGroupPropertyType.JSON_ARRAY);
} else {
properties.remove(licOptionsProperty);
licOptionsProperty.setValue(data);
}
properties.add(licOptionsProperty);
}
public boolean hasBillingOptions() {
return getBillingOptions().length() > 0;
}
public JSONArray getBillingOptions() {
SoftNodeGroupProperty options = getPropertyByName(BILLING_OPTIONS);
if (options != null) {
try {
return new JSONArray(options.getValue());
} catch (JSONException e) {
e.printStackTrace();
}
}
return new JSONArray();
}
public Response validateData(JSONObject data) {
if (data.has(ID)) {
return new Response(Response.INVALID_PARAM, MessageFormat.format("The property [{0}] is readonly", ID));
}
if (data.has(NAME)) {
return new Response(Response.INVALID_PARAM, MessageFormat.format("The property [{0}] is readonly", NAME));
}
if (data.has(DEPLOYMENTS)) {
return new Response(Response.INVALID_PARAM, MessageFormat.format("The property [{0}] is readonly", DEPLOYMENTS));
}
if (data.has(BILLING_OPTIONS)) {
return new Response(Response.INVALID_PARAM, String.format("The property [%s] is readonly", BILLING_OPTIONS));
}
if (data.has(SCALING_MODE)) {
if (data.isNull(SCALING_MODE)) {
return new Response(Response.INVALID_PARAM, MessageFormat.format("The property [{0}] cannot be NULL", SCALING_MODE));
}
try {
ScalingMode.valueOf(ScalingMode.class, data.getString(SCALING_MODE).toUpperCase());
} catch (Exception ex) {
return new Response(Response.INVALID_PARAM, MessageFormat.format("The property [{0}] is invalid. Available values of {1}", SCALING_MODE, ScalingMode.availableValues()));
}
}
return Response.OK();
}
public Response validate() throws JSONException {
int propertiesCount = getProperties().size();
if (propertiesCount > SoftNodeGroup.PROPERTY_MAX_COUNT) {
return new Response(Response.INVALID_PARAM, MessageFormat.format("Parameter [data] contains {0} properties. Maximum properties count is {1}", propertiesCount, SoftNodeGroup.PROPERTY_MAX_COUNT));
}
for (SoftNodeGroupProperty softNodeGroupProperty : getProperties()) {
if (softNodeGroupProperty.getName().length() > SoftNodeGroup.PROPERTY_NAME_MAX_LENGTH) {
return new Response(Response.VALUE_IS_TOO_LONG, MessageFormat.format("Property [name] is too long. Maximum length is {0} symbols", SoftNodeGroup.PROPERTY_NAME_MAX_LENGTH));
}
if (softNodeGroupProperty.getValue() != null && softNodeGroupProperty.getValue().length() > SoftNodeGroup.PROPERTY_VALUE_MAX_LENGTH) {
return new Response(Response.VALUE_IS_TOO_LONG, MessageFormat.format("Property [value] is too long. Maximum length is {0} symbols", SoftNodeGroup.PROPERTY_VALUE_MAX_LENGTH));
}
}
if (getDisplayName() != null && getDisplayName().length() > SoftNodeGroup.DISPLAY_NAME_MAX_LENGTH) {
return new Response(Response.VALUE_IS_TOO_LONG, MessageFormat.format("Display name is too long. Maximum length is {0} symbols", SoftNodeGroup.DISPLAY_NAME_MAX_LENGTH));
}
return Response.OK();
}
public Set getProperties() {
return properties;
}
public Set getSubdomains() {
return subdomains;
}
public void setSubdomains(Set subdomains) {
this.subdomains = subdomains;
}
public VType getvType() {
return vType;
}
public void setvType(VType vType) {
this.vType = vType;
}
public JSONObject toJSON(boolean isExport) throws JSONException {
JSONObject json = new JSONObject();
if (isExport) {
JSONArray propertiesJSONArray = new JSONArray();
for (SoftNodeGroupProperty property : properties) {
propertiesJSONArray.put(property.toJSON(isExport));
}
json.put(PROPERTIES, propertiesJSONArray);
} else {
putAllProperties(json);
}
json.put(NAME, name);
json.put(REDEPLOY_CONTAINER_DELAY, redeployContainerDelay);
json.put(RESTART_NODE_DELAY, restartNodeDelay);
json.put(RESTART_CONTAINER_DELAY, restartContainerDelay);
json.put(REDEPLOY_CONTEXT_DELAY, redeployContextDelay);
json.put(TEMPLATE_TYPE, templateType);
json.put(ENGINE_TYPE, engineType);
json.put(DISPLAY_NAME, displayName);
json.put(IS_SEQUENTIAL_DEPLOY, isSequentialDeploy);
json.put(SCALING_MODE, scalingMode);
json.put(IS_SLB_ACCESS_ENABLED, isSLBAccessEnabled);
if (vType != null) {
json.put(SoftwareNode.VTYPE, vType.toString());
}
if (contexts != null) {
JSONArray contextsJson = new JSONArray();
for (AppContext context : contexts) {
JSONObject contextJson = context._toJSON();
contextsJson.put(contextJson);
}
json.put(DEPLOYMENTS, contextsJson);
}
if (!softwareNodes.isEmpty()) {
JSONArray features = new JSONArray();
for (Feature feature : softwareNodes.iterator().next().getFeatures()) {
features.put(feature.getName());
}
json.put(FEATURES, features);
}
if (isExport) {
if (!rules.isEmpty()) {
JSONArray rulesJSONArray = new JSONArray();
for (FirewallRule rule : rules) {
rulesJSONArray.put(rule._toJSON());
}
json.put(FIREWALL_RULES, rulesJSONArray);
}
}
for (Map.Entry keyValue : propertiesMap.entrySet()) {
json.put(keyValue.getKey(), keyValue.getValue());
}
return json;
}
public void putAllProperties(JSONObject json) throws JSONException {
for (SoftNodeGroupProperty property : properties) {
if (property.getType() == SoftNodeGroupPropertyType.STRING) {
json.put(property.getName(), property.getValue());
} else if (property.getType() == SoftNodeGroupPropertyType.JSON_OBJECT) {
json.put(property.getName(), new JSONObject(property.getValue()));
} else if (property.getType() == SoftNodeGroupPropertyType.JSON_ARRAY) {
json.put(property.getName(), new JSONArray(property.getValue()));
} else if (property.getType() == SoftNodeGroupPropertyType.BOOLEAN) {
json.put(property.getName(), Boolean.valueOf(property.getValue()));
} else if (property.getType() == SoftNodeGroupPropertyType.NUMBER) {
try {
json.put(property.getName(), Long.valueOf(property.getValue()));
} catch (NumberFormatException ex) {
json.put(property.getName(), Double.valueOf(property.getValue()));
}
}
}
}
@Override
public JSONObject _toJSON() throws JSONException {
return toJSON(false);
}
private void setCustomProperty(String name, Object value) {
Optional propertyOptional = properties.stream().filter(p -> p.getName().equals(name)).findFirst();
SoftNodeGroupPropertyType type = SoftNodeGroupPropertyType.NUMBER;
if (value instanceof String) {
type = SoftNodeGroupPropertyType.STRING;
} else if (value instanceof Boolean) {
type = SoftNodeGroupPropertyType.BOOLEAN;
} else if (value instanceof JSONObject) {
type = SoftNodeGroupPropertyType.JSON_OBJECT;
} else if (value instanceof JSONArray) {
type = SoftNodeGroupPropertyType.JSON_ARRAY;
}
if (propertyOptional.isPresent()) {
propertyOptional.get().setType(type);
propertyOptional.get().setValue(value.toString());
} else {
properties.add(new SoftNodeGroupProperty(name, value.toString(), type));
}
}
private void removeCustomProperty(String name) {
properties.removeIf(p -> p.getName().equals(name));
}
private void setCustomProperties(JSONObject data) throws JSONException {
Iterator iterator = data.keys();
while (iterator.hasNext()) {
String key = (String) iterator.next();
if (StringUtils.isBlank(key)) {
continue;
}
Object value = data.isNull(key) ? null : data.get(key);
if (value == null) {
removeCustomProperty(key);
continue;
}
setCustomProperty(key, value);
}
}
private void setProperties(JSONObject data) throws JSONException {
setProperties(data, false);
}
private void setProperties(JSONObject data, boolean removeKeys) throws JSONException {
if (data.has(REDEPLOY_CONTAINER_DELAY)) {
setRedeployContainerDelay(data.getInt(REDEPLOY_CONTAINER_DELAY));
if (removeKeys) {
data.remove(REDEPLOY_CONTAINER_DELAY);
}
}
if (data.has(RESTART_NODE_DELAY)) {
setRestartNodeDelay(data.getInt(RESTART_NODE_DELAY));
if (removeKeys) {
data.remove(RESTART_NODE_DELAY);
}
}
if (data.has(RESTART_CONTAINER_DELAY)) {
setRestartContainerDelay(data.getInt(RESTART_CONTAINER_DELAY));
if (removeKeys) {
data.remove(RESTART_CONTAINER_DELAY);
}
}
if (data.has(REDEPLOY_CONTEXT_DELAY)) {
setRedeployContextDelay(data.getInt(REDEPLOY_CONTEXT_DELAY));
if (removeKeys) {
data.remove(REDEPLOY_CONTEXT_DELAY);
}
}
if (data.has(DISPLAY_NAME)) {
this.displayName = data.getString(DISPLAY_NAME);
if (removeKeys) {
data.remove(DISPLAY_NAME);
}
}
if (data.has(SCALING_MODE) && !data.isNull(SCALING_MODE)) {
this.scalingMode = ScalingMode.valueOf(ScalingMode.class, data.getString(SCALING_MODE).toUpperCase());
if (removeKeys) {
data.remove(SCALING_MODE);
}
}
if (data.has(IS_SLB_ACCESS_ENABLED) && !data.isNull(IS_SLB_ACCESS_ENABLED)) {
this.isSLBAccessEnabled = data.getBoolean(IS_SLB_ACCESS_ENABLED);
if (removeKeys) {
data.remove(IS_SLB_ACCESS_ENABLED);
}
}
}
@Override
public SoftNodeGroup _fromJSON(JSONObject json) throws JSONException {
setProperties(json);
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(TEMPLATE_TYPE)) {
this.templateType = TemplateType.valueOf(TemplateType.class, json.getString(TEMPLATE_TYPE).toUpperCase());
}
if (json.has(ENGINE_TYPE)) {
this.engineType = json.getString(ENGINE_TYPE).toLowerCase();
}
if (json.has(IS_SEQUENTIAL_DEPLOY)) {
this.isSequentialDeploy = json.getBoolean(IS_SEQUENTIAL_DEPLOY);
}
if (json.has(SoftwareNode.VTYPE)) {
this.vType = VType.valueOf(json.getString(SoftwareNode.VTYPE));
}
if (json.has(DEPLOYMENTS)) {
JSONArray contextsJson = json.getJSONArray(DEPLOYMENTS);
for (int i = 0; i < contextsJson.length(); i++) {
JSONObject contextJson = contextsJson.getJSONObject(i);
AppContext context = new AppContext()._fromJSON(contextJson);
contexts.add(context);
}
}
if (json.has(SCALING_MODE) && !json.isNull(SCALING_MODE)) {
this.scalingMode = ScalingMode.valueOf(ScalingMode.class, json.getString(SCALING_MODE).toUpperCase());
}
if (json.has(PROPERTIES)) {
JSONArray propertiesJSONArray = json.getJSONArray(PROPERTIES);
for (int i = 0; i < propertiesJSONArray.length(); i++) {
JSONObject propertyJson = propertiesJSONArray.getJSONObject(i);
SoftNodeGroupProperty property = new SoftNodeGroupProperty()._fromJSON(propertyJson);
properties.add(property);
}
}
if (json.has(FIREWALL_RULES)) {
JSONArray rulesJSONArray = json.getJSONArray(FIREWALL_RULES);
for (int i = 0; i < rulesJSONArray.length(); i++) {
JSONObject ruleJson = rulesJSONArray.getJSONObject(i);
FirewallRule rule = new FirewallRule()._fromJSON(ruleJson);
rules.add(rule);
}
}
if (json.has(IS_SLB_ACCESS_ENABLED)) {
this.isSLBAccessEnabled = json.getBoolean(IS_SLB_ACCESS_ENABLED);
}
Iterator iterator = json.keys();
while (iterator.hasNext()) {
String fieldName = iterator.next().toString();
Object fieldValue = null;
if (!json.isNull(fieldName)) {
try {
fieldValue = json.get(fieldName);
} catch (JSONException ignored) {
}
}
propertiesMap.put(fieldName, fieldValue);
}
return this;
}
public SoftNodeGroup cloneTo(SoftNodeGroup cloned) {
cloned.setName(this.name);
if (this.redeployContainerDelay != null) {
cloned.setRedeployContainerDelay(this.redeployContainerDelay);
}
if (this.redeployContextDelay != null) {
cloned.setRedeployContextDelay(this.redeployContextDelay);
}
if (this.restartContainerDelay != null) {
cloned.setRestartContainerDelay(this.restartContainerDelay);
}
if (this.restartNodeDelay != null) {
cloned.setRestartNodeDelay(this.restartNodeDelay);
}
if (this.templateType != null) {
cloned.setTemplateType(this.templateType);
}
cloned.setSequentialDeploy(this.isSequentialDeploy);
if (this.displayName != null) {
cloned.setDisplayName(this.displayName);
}
if (!properties.isEmpty()) {
HashSet clonedProperties = new HashSet<>();
for (SoftNodeGroupProperty property : properties) {
clonedProperties.add(new SoftNodeGroupProperty(property.getName(), property.getValue(), property.getType()));
}
cloned.properties.clear();
cloned.properties.addAll(clonedProperties);
}
cloned.setScalingMode(this.scalingMode);
cloned.setSLBAccessEnabled(this.isSLBAccessEnabled);
return cloned;
}
@Override
public SoftNodeGroup clone() {
SoftNodeGroup cloned = new SoftNodeGroup();
return cloneTo(cloned);
}
public static int normalizeDelay(int delay) {
if (delay < 0) {
return GROUP_OPERATION_DELAY_MIN_VALUE;
} else if (delay > GROUP_OPERATION_DELAY_MAX_VALUE) {
return GROUP_OPERATION_DELAY_MAX_VALUE;
}
return delay;
}
public SoftNodeGroupProperty getPropertyByName(String name) {
return getProperties().stream().filter(p -> StringUtils.equals(p.getName(), name)).findAny().orElse(null);
}
public String getPropertyValueByNameOrEmpty(String name) {
String result = null;
if (DISPLAY_NAME.equalsIgnoreCase(name)) {
result = getDisplayName();
} else {
SoftNodeGroupProperty softNodeGroupProperty = getPropertyByName(name);
if (softNodeGroupProperty != null) {
result = softNodeGroupProperty.getValue();
}
}
return result == null ? "" : result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy