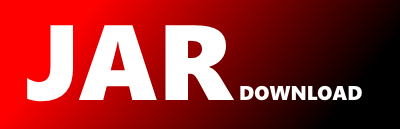
com.jelastic.api.system.persistence.SoftwareNode Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 525016de3885add37925f571348ffa6e*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.data.po.BuildCustomData;
import com.jelastic.api.data.po.DBItem;
import com.jelastic.api.data.po.DockerMetadata;
import com.jelastic.api.data.po.VType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import com.jelastic.api.system.service.utils.EnvironmentStatus;
import com.jelastic.api.system.service.utils.NodeUtils;
import com.jelastic.HibernatePBEStringEncryptor;
import com.jelastic.EncryptionOperationNotPossibleException;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SoftwareNode extends ArrayItem {
public static final String URL = "url";
public static final String STATUS = "status";
private static final Logger logger = Logger.getLogger(SoftwareNode.class.getName());
public static final String SOFT_NODE_COLUMN = "softNode";
public static final String HAS_PACKAGES = "hasPackages";
public static final String IS_WEB_ACCESS = "isWebAccess";
public static final String ADMIN_URL = "adminUrl";
public static final String USER = "user";
public static final String ADMIN_URLS = "adminUrls";
public static final String NODE_GROUP = "nodeGroup";
public static final String ADDONS = "addons";
public static final String DISK_IO_LIMIT = "diskIoLimit";
public static final String FILTER_IPS = "ips";
public static final String CUSTOM_ITEM = "customitem";
public static final String BUILD_CUSTOM_DATA = "buildCustomData";
public static final String IS_VCS_SUPPORT = "isVcsSupport";
public static final String IS_HIGH_AVAILABILITY = "isHighAvailability";
public static final String ENGINE_TYPE = "engineType";
public static final String CLOUDLETS_MIN_COUNT = "cloudletsMinCount";
public static final String NODE_TYPE = "nodeType";
public static final String NODE_TYPE_ALIAS = "nodeTypeAlias";
public static final String FEATURES = "features";
public static final String NAME = "name";
public static final String CTID = "ctid";
public static final String VTYPE = "vType";
public static final String IS_CUSTOM_SSL_SUPPORT = "isCustomSslSupport";
public static final String IS_CLUSTER_SUPPORT = "isClusterSupport";
public static final String MESSAGES = "messages";
public static final String ENV_ID = "envId";
public static final String MEM = "mem";
public static final String HDD = "hdd";
public static final String ENV_NAME = "envName";
public static final String VEID = "veid";
public static final String FLEXIBLE_CLOUDLETS = "flexibleCloudlets";
public static final String FIXED_CLOUDLETS = "fixedCloudlets";
public static final String DISPLAY_NAME = "displayName";
public static final String ENV = "env";
public static final String ENDPOINTS = "endpoints";
public static final String ENGINES = "engines";
public static final String PACKAGES = "packages";
public static final String CONTEXT_VALIDATOR_REGEX = "contextValidatorRegex";
public static final String SINGLE_CONTEXT = "singleContext";
public static final String TYPE = "type";
public static final String IS_RESET_PASSWORD = "isResetPassword";
public static final String IS_EXTERNAL_IP_REQUIRED = "isExternalIpRequired";
public static final String DOMAINSUFFIX = "domainsuffix";
public static final String NODEMISSION = "nodemission";
public static final String CAN_BE_EXPORTED = "canBeExported";
public static final String VERSION = "version";
public static final String PORT = "port";
public static final String INT_IP = "intIP";
public static final String ADDRESS = "address";
public static final String MAXCHANKS = "maxchanks";
public static final String ISMASTER = "ismaster";
public static final String ID = "id";
public static final String HN = "hn";
public static final String ACTIVE_ENGINE = "activeEngine";
public static final String CLUSTERGROUPID = "clustergroupid";
public static final String EXT_IPS = "extIPs";
public static final String EXTIPS = "extips";
public static final String EXTIPSV_6 = "extipsv6";
public static final String BANDWIDTH_LIMIT = "bandwidthLimit";
public static final String DISK_LIMIT = "diskLimit";
public static final String DISK_IOPS_LIMIT = "diskIopsLimit";
public static final String OS_TYPE = "osType";
public static final String GUEST_OS_TYPE = "guestOSType";
public static final String HOST_OS_TYPE = "hostOSType";
public static final String NODE_ID = "nodeId";
public static final String NODE_NAME = "nodeName";
public static final String CREDENTIAL = "credential";
public static final String VM_MEMORY = "vmMemory";
public static final String VM_VCPU = "vmVCPU";
public static final String CUSTOM_DATA = "customData";
public static final String SUBDOMAIN = "subdomain";
public static final String CROSS_SERVICE_IPS = "crossNetworkIps";
private HibernatePBEStringEncryptor hibernateStringEncryptor;
private Set filesPermissions = new HashSet<>();
protected boolean isMaster;
protected SoftwareNode origNode;
protected Set packages;
protected int maxchanks = -1;
private boolean isDeleted;
private Date deletedOn;
protected SoftNodeProperties properties;
private String customItem;
private VCSBranch branch;
private boolean isUpToDate;
private Date createdOn;
private int flexibleCloudlets;
private int fixedCloudlets;
private Integer bandwidthLimit;
private Integer diskLimit;
private Integer diskIopsLimit;
private Integer diskIoLimit;
private String displayName;
private AppNodes env;
private ClusterGroup clusterGroup;
private OsNode osNode;
private Set engines;
private Set features = new HashSet<>();
private Engine activeEngine;
protected String address;
protected String intIP;
protected HardwareNode hn;
protected List extIps = new ArrayList<>();
protected Set crossServiceIps = new HashSet<>();
protected String url;
protected Integer status;
protected Long mem;
protected Long hdd;
protected Boolean hasPackages;
protected Long veid;
protected String envName;
protected OSType osType;
protected OSType guestOSType;
protected OSType hostOSType;
protected Double vmMemory;
protected Integer vmVCPU;
private Set endpoints = new HashSet();
private String adminUrl;
private String adminUrls;
private String nodeGroup;
private Set addons = new HashSet<>();
private SoftNodeGroup softNodeGroup;
private String buildCustomData;
protected String engineType;
protected String nodeTypeAlias;
private ExtIpAddress primaryIpv4;
private ExtIpAddress primaryIpv6;
private Integer ctid;
private VType vType;
private Set messages = new HashSet<>();
private Set subdomains = new HashSet<>();
private String envId;
private Credential credential;
private Integer uid;
private String hostGroupUniqName;
private String hostGroupDisplayName;
private Project project;
public SoftwareNode() {
}
public SoftwareNode(int id) {
this.id = id;
}
public String getHostGroupUniqName() {
return hostGroupUniqName;
}
public void setHostGroupUniqName(String hostGroupUniqName) {
this.hostGroupUniqName = hostGroupUniqName;
}
public String getHostGroupDisplayName() {
return hostGroupDisplayName;
}
public void setHostGroupDisplayName(String hostGroupDisplayName) {
this.hostGroupDisplayName = hostGroupDisplayName;
}
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public Set getEndpoints() {
return endpoints;
}
public void setEndpoints(Set endpoints) {
this.endpoints = endpoints;
}
public Boolean isHasPackages() {
return hasPackages;
}
public void setHasPackages(Boolean hasPackages) {
this.hasPackages = hasPackages;
}
public Set getMountedFilePermissions() {
return getMountedFilePermissions(false);
}
public OsNode getOsNode() {
return osNode;
}
public void setOsNode(OsNode osNode) {
this.osNode = osNode;
}
public Set getMountedFilePermissions(boolean excludeExternal) {
Set filePermissions = new HashSet();
for (FilePermissions filePermission : this.filesPermissions) {
if (filePermission.isMounted()) {
if (excludeExternal && filePermission.isExternalMount()) {
continue;
}
filePermissions.add(filePermission);
}
}
return filePermissions;
}
public Set getFavoriteFilePermissions() {
Set filePermissions = new HashSet<>();
for (FilePermissions filePermission : this.filesPermissions) {
if (!filePermission.isMounted()) {
filePermissions.add(filePermission);
}
}
return filePermissions;
}
public Set getFilesPermissions() {
return filesPermissions;
}
public void setFilesPermissions(Set filesPermissions) {
this.filesPermissions = filesPermissions;
}
public int getMaxchanks() {
return maxchanks;
}
public void setMaxchanks(int maxchanks) {
this.maxchanks = maxchanks;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
this.intIP = address;
}
public void setIntIP(String intIP) {
this.intIP = intIP;
}
public String getIntIP() {
return intIP;
}
public boolean isMaster() {
return isMaster;
}
public void setIsMaster(boolean isMaster) {
this.isMaster = isMaster;
}
public Set getPackages() {
return packages;
}
public void setPackages(Set packages) {
this.packages = packages;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
}
public Date getDeletedOn() {
return deletedOn;
}
public void setDeletedOn(Date deletedOn) {
this.deletedOn = deletedOn;
}
public SoftNodeProperties getProperties() {
return properties;
}
public void setProperties(SoftNodeProperties properties) {
this.properties = properties;
}
public HardwareNode getHn() {
return hn;
}
public void setHn(HardwareNode hn) {
this.hn = hn;
}
public ClusterGroup getClusterGroup() {
return clusterGroup;
}
public void setClusterGroup(ClusterGroup clusterGroup) {
this.clusterGroup = clusterGroup;
}
public VCSBranch getBranch() {
return branch;
}
public void setBranch(VCSBranch branch) {
this.branch = branch;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Credential getCredential() {
return credential;
}
public void setCredential(Credential credential) {
this.credential = credential;
}
public Engine getActiveEngine() {
return activeEngine;
}
public void setActiveEngine(Engine activeEngine) {
this.activeEngine = activeEngine;
}
public BuildCustomData getBuildCustomData() {
if (buildCustomData == null) {
return null;
}
return new BuildCustomData(buildCustomData);
}
public void setBuildCustomData(BuildCustomData buildCustomData) {
this.buildCustomData = buildCustomData != null ? buildCustomData.toString() : null;
}
public Integer getDiskIopsLimit() {
return diskIopsLimit;
}
public void setDiskIopsLimit(Integer diskIopsLimit) {
this.diskIopsLimit = diskIopsLimit;
}
public void setDockerCustomItem(DBItem dbItem) {
this.setDockerMetadata(dbItem);
}
public void setCustomItem(String customItem) {
this.customItem = customItem;
}
public void setDockerMetadata(DBItem metadata) {
DockerMetadata dockerMetadata = (DockerMetadata) metadata;
if (dockerMetadata.getHubPassword() != null) {
try {
hibernateStringEncryptor.decrypt(dockerMetadata.getHubPassword());
} catch (EncryptionOperationNotPossibleException e) {
dockerMetadata.setHubPassword(hibernateStringEncryptor.encrypt(dockerMetadata.getHubPassword()));
}
}
this.customItem = dockerMetadata.getRaw();
}
public DBItem getDockerMetadata() {
if (customItem == null) {
return null;
}
try {
DockerMetadata dockerMetadata = new DockerMetadata(customItem);
if (dockerMetadata.getHubPassword() != null) {
try {
dockerMetadata.setHubPassword(hibernateStringEncryptor.decrypt(dockerMetadata.getHubPassword()));
} catch (EncryptionOperationNotPossibleException e) {
}
}
return dockerMetadata;
} catch (JSONException e) {
return null;
}
}
public void setRawBuildCustomData(String buildCustomData) {
this.buildCustomData = buildCustomData;
}
public DBItem getCustomItem() {
try {
return new DockerMetadata(customItem);
} catch (JSONException e) {
return null;
}
}
public String getRawCustomItem() {
return this.customItem;
}
public boolean isUpToDate() {
return isUpToDate;
}
public void setIsUpToDate(boolean isUpToDate) {
this.isUpToDate = isUpToDate;
}
public void setExtIps(List extIps) {
this.extIps = extIps;
for (ExtIpAddress extIpAddress : extIps) {
extIpAddress.setShortInfoMode(true);
}
}
public void setCrossServiceIps(Set crossServiceIps) {
this.crossServiceIps = crossServiceIps;
}
public List getExtIps() {
return extIps;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public void setStatus(EnvironmentStatus status) {
this.status = status.getValue();
}
public Long getMem() {
return mem;
}
public void setMem(Long mem) {
this.mem = mem;
}
public Long getHdd() {
return hdd;
}
public void setHdd(Long hdd) {
this.hdd = hdd;
}
public int getFixedCloudlets() {
return fixedCloudlets;
}
public void setFixedCloudlets(int fixedCloudlets) {
this.fixedCloudlets = fixedCloudlets;
}
public int getFlexibleCloudlets() {
return flexibleCloudlets;
}
public void setFlexibleCloudlets(int flexibleCloudlets) {
this.flexibleCloudlets = flexibleCloudlets;
}
public List getAllExtIpAddresses() {
return extIps;
}
public boolean isExtIpPresent() {
return extIps != null && !extIps.isEmpty();
}
public List getExtIpV6Addresses() {
List ips = new ArrayList<>();
for (ExtIpAddress ip : extIps) {
if (ip.getType() == IpType.IPV6) {
if (primaryIpv6 != null && primaryIpv6.getId() == ip.getId()) {
ips.add(0, ip);
} else {
ips.add(ip);
}
}
}
return ips;
}
public List getExtIpV4Addresses() {
List ips = new ArrayList<>();
for (ExtIpAddress ip : extIps) {
if (ip.getType() == IpType.IPV4) {
if (primaryIpv4 != null && primaryIpv4.getId() == ip.getId()) {
ips.add(0, ip);
} else {
ips.add(ip);
}
}
}
return ips;
}
public List getAllIps() {
List ips = new ArrayList<>();
if (this.getAddress() != null) {
ips.add(this.getAddress());
}
ips.addAll(getAllExtIpAddresses().stream().map(ExtIpAddress::getIpAddress).collect(Collectors.toList()));
return ips;
}
public Long getVeid() {
return veid;
}
public void setVeid(Long veid) {
this.veid = veid;
}
public String getEnvName() {
return envName;
}
public void setEnvName(String envName) {
this.envName = envName;
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public OSType getOsType() {
return osType;
}
public void setOsType(OSType osType) {
this.osType = osType;
}
public void setAdminUrl(String adminUrl) {
this.adminUrl = adminUrl;
}
public String getAdminUrl() {
return adminUrl;
}
public String getAdminUrls() {
return adminUrls;
}
public void setAdminUrls(String adminUrls) {
this.adminUrls = adminUrls;
}
public Integer getBandwidthLimit() {
return bandwidthLimit;
}
public void setBandwidthLimit(Integer bandwidthLimit) {
this.bandwidthLimit = bandwidthLimit;
}
public Integer getDiskLimit() {
return diskLimit;
}
public void setDiskLimit(Integer diskLimit) {
this.diskLimit = diskLimit;
}
public SoftwareNode getOrigNode() {
return origNode;
}
public void setOrigNode(SoftwareNode origNode) {
this.origNode = origNode;
}
public String getNodeGroup() {
return nodeGroup;
}
public void setNodeGroup(String nodeGroup) {
this.nodeGroup = nodeGroup;
}
public Set getAddons() {
return addons;
}
public void setAddons(Set addons) {
this.addons = addons;
}
public Integer getDiskIoLimit() {
return diskIoLimit;
}
public void setDiskIoLimit(Integer diskIoLimit) {
this.diskIoLimit = diskIoLimit;
}
public SoftNodeGroup getSoftNodeGroup() {
return softNodeGroup;
}
public void setSoftNodeGroup(SoftNodeGroup softNodeGroup) {
this.softNodeGroup = softNodeGroup;
}
public String getEngineType() {
return engineType;
}
public void setEngineType(String engineType) {
this.engineType = engineType;
}
public String getNodeTypeAlias() {
return nodeTypeAlias;
}
public void setNodeTypeAlias(String nodeTypeAlias) {
this.nodeTypeAlias = nodeTypeAlias;
}
public ExtIpAddress getPrimaryIpv4() {
return primaryIpv4;
}
public void setPrimaryIpv4(ExtIpAddress primaryIpv4) {
this.primaryIpv4 = primaryIpv4;
}
public ExtIpAddress getPrimaryIpv6() {
return primaryIpv6;
}
public void setPrimaryIpv6(ExtIpAddress primaryIpv6) {
this.primaryIpv6 = primaryIpv6;
}
public boolean isCertificated() {
return (getProperties().getType() == TemplateType.NATIVE || getProperties().getType() == TemplateType.CARTRIDGE || getProperties().getType() == TemplateType.DOCKERIZED) && !NodeUtils.NODE_MISSION_DOCKER.equals(getProperties().getNodeMission());
}
public boolean isFirewallSupport() {
return features.stream().anyMatch(f -> f.getName() == FeatureType.FIREWALL);
}
public Integer getCtid() {
return ctid;
}
public void setCtid(Integer ctid) {
this.ctid = ctid;
}
public String getEnvId() {
return envId;
}
public void setEnvId(String envId) {
this.envId = envId;
}
public Set getMessages() {
return messages;
}
public void setMessages(Set messages) {
this.messages = messages;
}
public Set getSubdomains() {
return subdomains;
}
public void setSubdomains(Set subdomains) {
this.subdomains = subdomains;
}
public Set getAllSubdomains() {
Set allSubdomains = new HashSet<>(subdomains);
allSubdomains.addAll(softNodeGroup.getSubdomains());
return allSubdomains;
}
public Set getAllSubdomains(boolean topLevel) {
return getAllSubdomains().stream().filter(s -> s.isTopLevel() == topLevel).collect(Collectors.toSet());
}
public SoftwareNode cloneTo(SoftwareNode cloned) {
cloned.setMaxchanks(this.maxchanks);
cloned.setAddress(this.address);
cloned.setProperties(this.properties);
cloned.setBranch(this.branch);
cloned.setIsUpToDate(this.isUpToDate);
cloned.setCustomItem(this.customItem);
cloned.setBandwidthLimit(this.bandwidthLimit);
cloned.setDiskLimit(this.diskLimit);
cloned.setDiskIopsLimit(this.diskIopsLimit);
cloned.setDiskIoLimit(this.diskIoLimit);
cloned.setCreatedOn(new Date());
cloned.setRawBuildCustomData(this.buildCustomData);
cloned.setNodeTypeAlias(this.nodeTypeAlias);
for (Addon addon : this.getAddons()) {
cloned.getAddons().add(new Addon(addon.getUniqueName(), addon.getAppTemplateId()));
}
return cloned;
}
@Override
public SoftwareNode clone() {
SoftwareNode cloned = new SoftwareNode();
return cloneTo(cloned);
}
public JSONObject toJSON(boolean isExport) throws JSONException {
JSONObject json = new JSONObject();
json.putOpt(ID, id);
json.putOpt(ISMASTER, isMaster);
if (address != null) {
json.putOpt(ADDRESS, address);
}
if (intIP != null) {
json.putOpt(INT_IP, intIP);
}
json.putOpt(HAS_PACKAGES, hasPackages);
if (maxchanks != -1 && vType != VType.VM) {
json.putOpt(MAXCHANKS, maxchanks);
}
if (properties != null) {
if (properties.getPort() != -1) {
json.putOpt("port", properties.getPort());
}
if (StringUtils.isBlank(nodeTypeAlias)) {
json.putOpt(NODE_TYPE, properties.getNodeType());
} else {
json.putOpt(NODE_TYPE, nodeTypeAlias);
}
json.putOpt(NODEMISSION, properties.getNodeMission());
json.putOpt(VERSION, properties.getVersion());
json.putOpt(NAME, properties.getName());
json.putOpt(DOMAINSUFFIX, properties.getDomainSuffix());
json.putOpt(SINGLE_CONTEXT, properties.isSingleContext());
json.putOpt(TYPE, properties.getType() != null ? properties.getType().toString() : null);
json.putOpt(IS_CUSTOM_SSL_SUPPORT, properties.isCustomSslSupport());
json.putOpt(IS_CLUSTER_SUPPORT, NodeUtils.isClusterSupport(this));
json.putOpt(IS_EXTERNAL_IP_REQUIRED, properties.isExternalIpRequired());
json.putOpt(IS_RESET_PASSWORD, properties.isResetPassword());
json.putOpt(CAN_BE_EXPORTED, properties.canBeExported());
json.putOpt(IS_WEB_ACCESS, properties.isWebAccess());
json.putOpt(USER, properties.getUser());
String contextValidatorRegex = properties.getContextValidatorRegex();
json.putOpt(CONTEXT_VALIDATOR_REGEX, contextValidatorRegex != null ? contextValidatorRegex : SoftNodeProperties.DEFAULT_CONTEXT_VALIDATOR);
boolean isBuildNodeWithBuildData = NodeUtils.NODE_MISSION_BUILD.equals(properties.getNodeMission()) && getBuildCustomData() != null;
JSONObject buildCustomDataJsonObject = null;
if (isBuildNodeWithBuildData) {
buildCustomDataJsonObject = getBuildCustomData()._toJSON();
}
if (customItem != null && getDockerMetadata() != null) {
if (NodeUtils.NODE_MISSION_DOCKER.equals(properties.getNodeMission()) || this.properties.getType() == TemplateType.DOCKERIZED) {
try {
DockerMetadata dockerMetadata = new DockerMetadata(customItem);
if (NodeUtils.NODE_MISSION_DOCKER.equals(properties.getNodeMission())) {
json.putOpt(NAME, dockerMetadata.getName());
}
json.putOpt(VERSION, dockerMetadata.getTag());
} catch (JSONException e) {
}
}
JSONObject customItemJsonObject = ((DockerMetadata) getDockerMetadata()).toJSON(isExport);
if (buildCustomDataJsonObject != null) {
merge(buildCustomDataJsonObject, customItemJsonObject);
}
json.putOpt(CUSTOM_ITEM, customItemJsonObject);
} else if (isBuildNodeWithBuildData) {
json.putOpt(CUSTOM_ITEM, buildCustomDataJsonObject);
}
json.putOpt(IS_VCS_SUPPORT, properties.isVcsSupport());
json.putOpt(IS_HIGH_AVAILABILITY, properties.isHighAvailability());
json.putOpt(CLOUDLETS_MIN_COUNT, properties.getCloudletsMinCount());
}
if (packages != null) {
JSONArray jsonPcks = new JSONArray();
for (SoftwarePackage pack : packages) {
pack.setInstalled(true);
jsonPcks.put(pack._toJSON());
}
json.putOpt(PACKAGES, jsonPcks);
}
if (engines != null && !engines.isEmpty()) {
JSONArray jsonEngines = new JSONArray();
for (Engine engine : engines) {
jsonEngines.put(engine._toJSON());
}
json.putOpt(ENGINES, jsonEngines);
}
if (activeEngine != null) {
json.putOpt(ACTIVE_ENGINE, activeEngine._toJSON());
}
if (endpoints != null) {
JSONArray jsonEndpoints = new JSONArray();
for (Endpoint endpoint : endpoints) {
jsonEndpoints.put(endpoint._toJSON());
}
json.putOpt(ENDPOINTS, jsonEndpoints);
}
if (hn != null) {
json.putOpt(HN, hn._toJSON());
}
if (clusterGroup != null) {
json.putOpt(CLUSTERGROUPID, clusterGroup.getId());
}
if (osType != null) {
json.putOpt(OS_TYPE, osType.toString());
}
if (guestOSType != null) {
json.putOpt(GUEST_OS_TYPE, guestOSType.toString());
}
if (hostOSType != null) {
json.putOpt(HOST_OS_TYPE, hostOSType.toString());
}
if (vmMemory != null) {
json.put(VM_MEMORY, vmMemory);
}
if (vmVCPU != null) {
json.put(VM_VCPU, vmVCPU);
}
if (getExtIpV4Addresses() != null && getExtIpV4Addresses().size() > 0) {
JSONArray ips = new JSONArray();
for (ExtIpAddress extIp : getExtIpV4Addresses()) {
ips.put(extIp.getIpAddress());
}
json.putOpt(EXTIPS, ips);
json.putOpt(EXT_IPS, ips);
}
if (getExtIpV6Addresses() != null && getExtIpV6Addresses().size() > 0) {
JSONArray ips = new JSONArray();
for (ExtIpAddress extIp : getExtIpV6Addresses()) {
ips.put(extIp.getIpAddress());
}
json.putOpt(EXTIPSV_6, ips);
}
if (crossServiceIps != null) {
JSONArray jsonEngines = new JSONArray();
for (CrossNetworkIpAddress crossNetworkIpAddress : crossServiceIps) {
jsonEngines.put(crossNetworkIpAddress.getIpAddress());
}
json.putOpt(CROSS_SERVICE_IPS, jsonEngines);
}
if (this.bandwidthLimit != null) {
json.putOpt(BANDWIDTH_LIMIT, bandwidthLimit);
}
if (this.diskLimit != null) {
json.putOpt(DISK_LIMIT, diskLimit);
}
if (this.diskIopsLimit != null) {
json.putOpt(DISK_IOPS_LIMIT, diskIopsLimit);
}
if (this.diskIoLimit != null) {
json.putOpt(DISK_IO_LIMIT, diskIoLimit);
}
json.putOpt(URL, url);
json.putOpt(STATUS, status);
json.putOpt(MEM, mem);
json.putOpt(HDD, hdd);
json.putOpt(ENV_NAME, envName);
json.putOpt(VEID, veid);
json.putOpt(FLEXIBLE_CLOUDLETS, flexibleCloudlets);
json.putOpt(FIXED_CLOUDLETS, fixedCloudlets);
json.putOpt(DISPLAY_NAME, displayName);
json.putOpt(ADMIN_URL, adminUrl);
json.putOpt(ADMIN_URLS, adminUrls);
json.putOpt(NODE_GROUP, nodeGroup);
if (credential != null) {
json.put(CREDENTIAL, credential._toJSON());
}
if (addons != null) {
JSONArray jsonAddons = new JSONArray();
for (Addon addon : addons) {
jsonAddons.put(addon._toJSON());
}
json.putOpt(ADDONS, jsonAddons);
}
json.putOpt(ENGINE_TYPE, engineType);
json.putOpt(CTID, ctid);
if (vType != null) {
json.put(VTYPE, vType.toString());
}
if (messages != null) {
JSONArray array = new JSONArray();
for (Message message : messages) {
array.put(message._toJSON());
}
json.putOpt(MESSAGES, array);
}
if (!features.isEmpty()) {
JSONArray featuresJSONArray = new JSONArray();
for (Feature feature : features) {
featuresJSONArray.put(feature.getName().toString());
}
json.putOpt(FEATURES, featuresJSONArray);
}
if (isExport) {
json.putOpt(ENV_ID, envId);
}
return json;
}
public JSONObject _toJSON() throws JSONException {
return toJSON(false);
}
private static JSONObject merge(JSONObject from, JSONObject to) throws JSONException {
Iterator keys = from.keys();
while (keys.hasNext()) {
String name = keys.next();
to.putOpt(name, from.get(name));
}
return to;
}
public Set getEngines() {
return engines;
}
public void setEngines(Set engines) {
this.engines = engines;
}
public SoftwareNode _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(HAS_PACKAGES)) {
hasPackages = json.getBoolean(HAS_PACKAGES);
}
if (json.has(ISMASTER)) {
this.isMaster = json.getBoolean(ISMASTER);
}
if (json.has(MAXCHANKS)) {
this.maxchanks = json.getInt(MAXCHANKS);
}
if (json.has(ADDRESS)) {
this.address = json.getString(ADDRESS);
}
if (json.has(INT_IP)) {
this.intIP = json.getString(INT_IP);
}
this.properties = new SoftNodeProperties();
if (json.has(PORT)) {
this.properties.setPort(json.getInt(PORT));
}
if (json.has(VERSION)) {
this.properties.setVersion(json.getString(VERSION));
}
if (json.has(NODE_TYPE)) {
this.properties.setNodeType(json.getString(NODE_TYPE));
}
if (json.has(CAN_BE_EXPORTED)) {
this.properties.setCanBeExported(json.getBoolean(CAN_BE_EXPORTED));
}
if (json.has(NODEMISSION)) {
this.properties.setNodeMission(json.getString(NODEMISSION));
}
if (json.has(DOMAINSUFFIX)) {
this.properties.setDomainSuffix(json.getString(DOMAINSUFFIX));
}
if (json.has(NAME)) {
this.properties.setName(json.getString(NAME));
}
if (json.has(USER)) {
this.properties.setUser(json.getString(USER));
}
if (json.has(IS_CLUSTER_SUPPORT)) {
this.properties.setClusterSupport(json.getBoolean(IS_CLUSTER_SUPPORT));
}
if (json.has(IS_CUSTOM_SSL_SUPPORT)) {
this.properties.setCustomSslSupport(json.getBoolean(IS_CUSTOM_SSL_SUPPORT));
}
if (json.has(IS_EXTERNAL_IP_REQUIRED)) {
this.properties.setExternalIpRequired(json.getBoolean(IS_EXTERNAL_IP_REQUIRED));
}
if (json.has(IS_RESET_PASSWORD)) {
this.properties.setResetPassword(json.getBoolean(IS_RESET_PASSWORD));
}
if (json.has(IS_VCS_SUPPORT)) {
this.properties.setVcsSupport(json.getBoolean(IS_VCS_SUPPORT));
}
if (json.has(IS_HIGH_AVAILABILITY)) {
this.properties.setHighAvailability(json.getBoolean(IS_HIGH_AVAILABILITY));
}
if (json.has(CLOUDLETS_MIN_COUNT)) {
this.properties.setCloudletsMinCount(json.getInt(CLOUDLETS_MIN_COUNT));
}
if (json.has(TYPE)) {
try {
this.properties.setType(TemplateType.valueOf(TemplateType.class, json.getString(TYPE).toUpperCase()));
} catch (IllegalArgumentException e) {
logger.log(Level.WARNING, "Can't parse template type '{0}'", json.get(TYPE));
}
}
if (json.has(SINGLE_CONTEXT)) {
this.properties.setSingleContext(json.getBoolean(SINGLE_CONTEXT));
}
if (json.has(CONTEXT_VALIDATOR_REGEX)) {
this.properties.setContextValidatorRegex(json.getString(CONTEXT_VALIDATOR_REGEX));
}
if (json.has(CUSTOM_ITEM)) {
JSONObject jsonObject = json.getJSONObject(CUSTOM_ITEM);
if (TemplateType.DOCKERIZED.equals(properties.getType()) || NodeUtils.NODE_MISSION_DOCKER.equals(properties.getNodeMission())) {
setDockerCustomItem(new DockerMetadata(jsonObject));
}
if (NodeUtils.NODE_MISSION_BUILD.equals(properties.getNodeMission())) {
setBuildCustomData(new BuildCustomData(jsonObject));
}
}
if (json.has(IS_WEB_ACCESS)) {
this.properties.setWebAccess(json.getBoolean(IS_WEB_ACCESS));
}
if (json.has(PACKAGES)) {
this.packages = new HashSet();
JSONArray pcks = json.getJSONArray(PACKAGES);
for (int i = 0; i < pcks.length(); i++) {
JSONObject jsonObj = (JSONObject) pcks.get(i);
this.packages.add(new SoftwarePackage()._fromJSON(jsonObj));
}
}
if (json.has(ENDPOINTS)) {
this.endpoints = new HashSet();
JSONArray jsonEndpoints = json.getJSONArray(ENDPOINTS);
for (int i = 0; i < jsonEndpoints.length(); i++) {
JSONObject jsonObj = (JSONObject) jsonEndpoints.get(i);
this.endpoints.add(new Endpoint()._fromJSON(jsonObj));
}
}
if (json.has(ENGINES)) {
this.engines = new HashSet();
JSONArray jsonEngines = json.getJSONArray(ENGINES);
for (int i = 0; i < jsonEngines.length(); i++) {
JSONObject jsonObj = (JSONObject) jsonEngines.get(i);
this.engines.add(new Engine()._fromJSON(jsonObj));
}
}
if (json.has(HN)) {
this.hn = new HardwareNode()._fromJSON(json.getJSONObject(HN));
}
if (json.has(ACTIVE_ENGINE)) {
this.activeEngine = new Engine()._fromJSON(json.getJSONObject(ACTIVE_ENGINE));
}
if (json.has(CLUSTERGROUPID)) {
this.clusterGroup = new ClusterGroup();
this.clusterGroup.setId(json.getInt(CLUSTERGROUPID));
}
if (json.has(EXTIPS) || json.has(EXT_IPS)) {
JSONArray ips = json.has(EXTIPS) ? json.getJSONArray(EXTIPS) : json.getJSONArray(EXT_IPS);
for (int i = 0; i < ips.length(); i++) {
ExtIpAddress ip = new ExtIpAddress(ips.getString(i), true);
ip.setType(IpType.IPV4);
extIps.add(ip);
}
}
if (json.has(EXTIPSV_6)) {
JSONArray ips = json.getJSONArray(EXTIPSV_6);
for (int i = 0; i < ips.length(); i++) {
ExtIpAddress ip = new ExtIpAddress(ips.getString(i), true);
ip.setType(IpType.IPV6);
extIps.add(ip);
}
}
if (json.has(CROSS_SERVICE_IPS)) {
JSONArray ips = json.getJSONArray(CROSS_SERVICE_IPS);
for (int i = 0; i < ips.length(); i++) {
CrossNetworkIpAddress crossNetworkIpAddress = new CrossNetworkIpAddress();
crossNetworkIpAddress.setIpAddress(ips.getString(i));
crossServiceIps.add(crossNetworkIpAddress);
}
}
if (json.has(URL)) {
this.url = json.getString(URL);
}
if (json.has(STATUS)) {
this.status = json.getInt(STATUS);
}
if (json.has(MEM)) {
this.mem = json.getLong(MEM);
}
if (json.has(HDD)) {
this.hdd = json.getLong(HDD);
}
if (json.has(VEID)) {
this.veid = json.getLong(VEID);
}
if (json.has(ENV_NAME)) {
this.envName = json.getString(ENV_NAME);
}
if (json.has(FLEXIBLE_CLOUDLETS)) {
flexibleCloudlets = json.getInt(FLEXIBLE_CLOUDLETS);
}
if (json.has(FIXED_CLOUDLETS)) {
fixedCloudlets = json.getInt(FIXED_CLOUDLETS);
}
if (json.has(BANDWIDTH_LIMIT)) {
bandwidthLimit = json.getInt(BANDWIDTH_LIMIT);
}
if (json.has(DISK_LIMIT)) {
diskLimit = json.getInt(DISK_LIMIT);
}
if (json.has(DISK_IOPS_LIMIT)) {
diskIopsLimit = json.getInt(DISK_IOPS_LIMIT);
}
if (json.has(DISK_IO_LIMIT)) {
diskIoLimit = json.getInt(DISK_IO_LIMIT);
}
if (json.has(DISPLAY_NAME)) {
displayName = json.getString(DISPLAY_NAME);
}
if (json.has(OS_TYPE)) {
osType = OSType.valueOf(OSType.class, json.getString(OS_TYPE));
}
if (json.has(GUEST_OS_TYPE)) {
guestOSType = OSType.valueOf(OSType.class, json.getString(GUEST_OS_TYPE));
}
if (json.has(HOST_OS_TYPE)) {
osType = OSType.valueOf(OSType.class, json.getString(HOST_OS_TYPE));
}
if (json.has(VM_MEMORY)) {
vmMemory = json.getDouble(VM_MEMORY);
}
if (json.has(VM_VCPU)) {
vmVCPU = json.getInt(VM_VCPU);
}
if (json.has(ADMIN_URL)) {
adminUrl = json.getString(ADMIN_URL);
}
if (json.has(NODE_GROUP)) {
nodeGroup = json.getString(NODE_GROUP);
}
if (json.has(CREDENTIAL)) {
credential = (Credential) new Credential()._fromJSON(json.getJSONObject(CREDENTIAL));
}
if (json.has(ADDONS)) {
this.addons = new HashSet();
JSONArray jsonAddons = json.getJSONArray(ADDONS);
for (int i = 0; i < jsonAddons.length(); i++) {
JSONObject jsonObj = (JSONObject) jsonAddons.get(i);
this.addons.add(new Addon()._fromJSON(jsonObj));
}
}
if (json.has(ENGINE_TYPE)) {
engineType = json.getString(ENGINE_TYPE).toLowerCase();
}
if (json.has(FEATURES)) {
this.features = new HashSet();
JSONArray jsonFeatures = json.getJSONArray(FEATURES);
for (int i = 0; i < jsonFeatures.length(); i++) {
String featureName = jsonFeatures.getString(i);
FeatureType type = FeatureType.valueOf(FeatureType.class, featureName.toUpperCase());
this.features.add(new Feature(type));
}
}
if (json.has(CTID)) {
this.ctid = json.getInt(CTID);
}
if (json.has(VTYPE)) {
this.vType = VType.valueOf(json.getString(VTYPE));
}
if (json.has(ENV_ID)) {
this.envId = json.getString(ENV_ID);
}
return this;
}
public JSONObject toJSONWithFilters(String... filters) throws JSONException {
JSONObject json = new JSONObject();
json.putOpt(ID, id);
for (String filter : filters) {
switch(filter) {
case FILTER_IPS:
json.putOpt(ADDRESS, address);
json.putOpt(INT_IP, intIP);
if (getExtIpV4Addresses() != null && getExtIpV4Addresses().size() > 0) {
JSONArray ips = new JSONArray();
for (ExtIpAddress extIp : getExtIpV4Addresses()) {
ips.put(extIp.getIpAddress());
}
json.putOpt(EXT_IPS, ips);
}
if (getExtIpV6Addresses() != null && getExtIpV6Addresses().size() > 0) {
JSONArray ips = new JSONArray();
for (ExtIpAddress extIp : getExtIpV6Addresses()) {
ips.put(extIp.getIpAddress());
}
json.putOpt(EXTIPSV_6, ips);
}
break;
default:
break;
}
}
return json;
}
public Double getVmMemory() {
return vmMemory;
}
public void setVmMemory(Double vmMemory) {
this.vmMemory = vmMemory;
}
public Integer getVmVCPU() {
return vmVCPU;
}
public void setVmVCPU(Integer vmVCPU) {
this.vmVCPU = vmVCPU;
}
public VType getvType() {
return vType;
}
public void setvType(VType vType) {
this.vType = vType;
}
public Set getFeatures() {
return features;
}
public void setFeatures(Set features) {
this.features = features;
}
public AppNodes getEnv() {
return env;
}
public void setEnv(AppNodes env) {
this.env = env;
}
public OSType getGuestOSType() {
return guestOSType;
}
public void setGuestOSType(OSType guestOSType) {
this.guestOSType = guestOSType;
}
public OSType getHostOSType() {
return hostOSType;
}
public void setHostOSType(OSType hostOSType) {
this.hostOSType = hostOSType;
}
public Project getProject() {
return project;
}
public void setProject(Project project) {
this.project = project;
}
@Override
public String toString() {
return "SoftwareNode{" + "displayName='" + displayName + '\'' + ", nodeGroup='" + nodeGroup + '\'' + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy