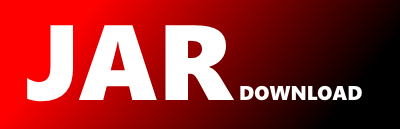
com.jelastic.api.system.persistence.SoftwarePackage Maven / Gradle / Ivy
The newest version!
/*Server class MD5: aff713497c496480f00ea15c945ff1d2*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SoftwarePackage extends ArrayItem {
public static final String ICON_URL = "iconurl";
public static final String DESCRIPTION = "description";
public static final String DOCUMENTATION_URL = "documentationurl";
public static final String KEYWORD_FTP = "ftp";
public static final String KEYWORD = "keyword";
public static final String IS_INSTALLED = "isInstalled";
public static final String NAME = "name";
private String name;
private List templates;
private Set actions;
private Set requirements;
private String packageName;
private String keyword;
private String user;
private String emailTemplate;
private String iconUrl;
private String description;
private String documentationUrl;
private boolean reconfigureFirewall;
private boolean isInstalled;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getTemplates() {
return templates;
}
public void setTemplates(List templates) {
this.templates = templates;
}
public Set getActions() {
return actions;
}
public void setActions(Set actions) {
this.actions = actions;
}
public Set getRequirements() {
return requirements;
}
public void setRequirements(Set requirements) {
this.requirements = requirements;
}
public String getPackageName() {
return packageName;
}
public void setPackageName(String packageName) {
this.packageName = packageName;
}
public String getKeyword() {
return keyword;
}
public void setKeyword(String keyword) {
this.keyword = keyword;
}
public boolean isInstalled() {
return isInstalled;
}
public void setInstalled(boolean installed) {
isInstalled = installed;
}
public String getUser() {
return user;
}
public void setUser(String user) {
this.user = user;
}
public String getEmailTemplate() {
return emailTemplate;
}
public void setEmailTemplate(String emailTemplate) {
this.emailTemplate = emailTemplate;
}
public String getIconUrl() {
return iconUrl;
}
public void setIconUrl(String iconUrl) {
this.iconUrl = iconUrl;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getDocumentationUrl() {
return documentationUrl;
}
public void setDocumentationUrl(String documentationUrl) {
this.documentationUrl = documentationUrl;
}
public boolean isReconfigureFirewall() {
return reconfigureFirewall;
}
public void setReconfigureFirewall(boolean reconfigureFirewall) {
this.reconfigureFirewall = reconfigureFirewall;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("id", id);
json.put("name", name);
if (documentationUrl != null) {
json.put(DOCUMENTATION_URL, documentationUrl);
}
if (description != null) {
json.put(DESCRIPTION, description);
}
if (iconUrl != null) {
json.put(ICON_URL, iconUrl);
}
if (actions != null && !actions.isEmpty()) {
JSONArray jsonArray = new JSONArray();
for (SoftwarePackageAction action : actions) {
jsonArray.put(action.toJSON());
}
json.put("actions", jsonArray);
}
if (requirements != null && !requirements.isEmpty()) {
JSONArray jsonArray = new JSONArray();
for (SoftwarePackageRequirement requirement : requirements) {
jsonArray.put(requirement.toJSON());
}
json.put("requirements", jsonArray);
}
json.put(KEYWORD, keyword);
json.put(IS_INSTALLED, isInstalled);
return json;
}
@Override
public SoftwarePackage _fromJSON(JSONObject json) throws JSONException {
if (json.has(ICON_URL)) {
iconUrl = json.getString(ICON_URL);
}
if (json.has(DESCRIPTION)) {
description = json.getString(DESCRIPTION);
}
if (json.has(DOCUMENTATION_URL)) {
documentationUrl = json.getString(DOCUMENTATION_URL);
}
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has("name")) {
this.name = json.getString("name");
}
if (json.has("actions")) {
actions = new HashSet();
JSONArray jsonArray = json.getJSONArray("actions");
for (int i = 0; i < jsonArray.length(); i++) {
actions.add(new SoftwarePackageAction().fromJSON(jsonArray.getJSONObject(i)));
}
}
if (json.has("requirements")) {
requirements = new HashSet();
JSONArray jsonArray = json.getJSONArray("requirements");
for (int i = 0; i < jsonArray.length(); i++) {
requirements.add(new SoftwarePackageRequirement().fromJSON(jsonArray.getJSONObject(i)));
}
}
if (json.has(KEYWORD)) {
this.keyword = json.getString(KEYWORD);
}
if (json.has(IS_INSTALLED)) {
isInstalled = json.getBoolean(IS_INSTALLED);
}
return this;
}
public SoftwarePackage cloneTo(SoftwarePackage cloned) {
cloned.setName(this.name);
cloned.setActions(this.actions);
cloned.setDescription(this.description);
cloned.setDocumentationUrl(this.documentationUrl);
cloned.setEmailTemplate(this.emailTemplate);
cloned.setKeyword(this.keyword);
cloned.setIconUrl(this.iconUrl);
cloned.setInstalled(this.isInstalled);
cloned.setReconfigureFirewall(this.reconfigureFirewall);
return cloned;
}
@Override
public SoftwarePackage clone() {
SoftwarePackage cloned = new SoftwarePackage();
return cloneTo(cloned);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy