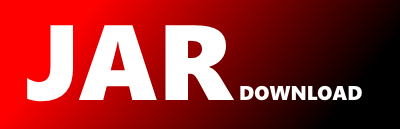
com.jelastic.api.system.persistence.TariffPlan Maven / Gradle / Ivy
/*Server class MD5: e7331bed42bba4d9665ac9024e447c7a*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.pricing.strategy.CalculatingStrategyType;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.annotation.Transform;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.system.persistence.pricing.TariffGrid;
import com.jelastic.api.system.persistence.pricing.TariffGridItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.math.BigDecimal;
import java.text.ParseException;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TariffPlan extends ArrayItem implements Serializable {
public static final String IS_DELETED_FILTER = "isDeletedFilter";
public static final String IS_DELETED_FILTER_PARAM = "isDeleted";
public static final String UNIQUE_NAME = "uniqueName";
public static final String NAME = "name";
private static final String CURRENCY = "currency";
public static final String RESELLER = "reseller";
private static final String MINIMUM_FEE = "minimumFee";
private static final String CONVERTED_MINIMUM_FEE = "convertedMinimumFee";
private static final String MINIMUM_FEE_RESOURCE_AMOUNT = "minimumFeeResourceAmount";
private static final String DOC_URL = "docUrl";
private static final String OVERRIDES_TYPES = "overridesTypes";
private static final String RECURRING_INTERVAL = "recurringInterval";
private static final String SKU = "sku";
private String uniqueName;
private String keyword;
@Transform(required = true)
private String name;
@Transform(required = true)
private String description;
@Transform(required = true)
private TariffPlanType type;
@Transform
private List overridesTypes;
private Date createdOn;
private Date updatedOn;
private boolean isDeleted;
@Transform(required = true)
private Set tiers;
@Transform(required = true)
private int minCloudletCount;
@Transform(required = true)
private int cloudletIncrement;
private Reseller reseller;
private CalculatingStrategyType strategy;
private CalculatingStrategyData strategyData;
private Resource resource;
private ChargeScope freeResourceScope = ChargeScope.ENVIRONMENT;
private ChargeScope billableResourceScope = ChargeScope.ENVIRONMENT;
private Boolean chargeOnlyRunning;
private BigDecimal minimumFee;
private BigDecimal convertedMinimumFee;
private Double minimumFeeResourceAmount;
private String docUrl;
private Currency currency;
private PriceType priceType = PriceType.RECURRING;
private RecurringInterval recurringInterval = RecurringInterval.HOUR;
private int recurringIntervalCount = 1;
private double cloudletPrice;
private double capacityPrice;
private int freeCapacity;
private int freeCloudlet;
private int pricePeriod;
private int billingPeriod;
private String currencyISO;
private String baseCurrency;
private TariffGrid tariffGrid;
private TariffGridItem tariffGridItem;
private String sku;
public String getUniqueName() {
return uniqueName;
}
public void setUniqueName(String uniqueName) {
this.uniqueName = uniqueName;
}
public void generateUniqName() {
this.uniqueName = UUID.randomUUID().toString();
}
public int getBillingPeriod() {
return billingPeriod;
}
public void setBillingPeriod(int billingPeriod) {
this.billingPeriod = billingPeriod;
}
public double getCapacityPrice() {
return capacityPrice;
}
public void setCapacityPrice(double capacityPrice) {
this.capacityPrice = capacityPrice;
}
public double getCloudletPrice() {
return cloudletPrice;
}
public int getFreeCapacity() {
return freeCapacity;
}
public void setFreeCapacity(int freeCapacity) {
this.freeCapacity = freeCapacity;
}
public int getFreeCloudlet() {
return freeCloudlet;
}
public void setFreeCloudlet(int freeCloudlet) {
this.freeCloudlet = freeCloudlet;
}
public void setCloudletPrice(double cloudletPrice) {
this.cloudletPrice = cloudletPrice;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getKeyword() {
return keyword;
}
public String getConsumptionKeyword() {
if (strategy.isBasedOnItem()) {
if (strategyData != null && strategyData.getSecondary() != null) {
return strategyData.getSecondary().getName();
}
}
return keyword;
}
public void setKeyword(String keyword) {
this.keyword = keyword;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getPricePeriod() {
return pricePeriod;
}
public void setPricePeriod(int pricePeriod) {
this.pricePeriod = pricePeriod;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public Set getTiers() {
return tiers;
}
public void setTiers(Set tiers) {
this.tiers = tiers;
}
public TariffPlanType getType() {
return type;
}
public void setType(TariffPlanType type) {
this.type = type;
}
public int getMinCloudletCount() {
return minCloudletCount;
}
public void setMinCloudletCount(int minCloudletCount) {
this.minCloudletCount = minCloudletCount;
}
public int getCloudletIncrement() {
return cloudletIncrement;
}
public void setCloudletIncrement(int cloudletIncrement) {
this.cloudletIncrement = cloudletIncrement;
}
public String getBaseCurrency() {
return baseCurrency;
}
public void setBaseCurrency(String baseCurrency) {
this.baseCurrency = baseCurrency;
}
public Reseller getReseller() {
return reseller;
}
public void setReseller(Reseller reseller) {
this.reseller = reseller;
}
public CalculatingStrategyType getStrategyType() {
return this.strategy;
}
public void setStrategy(CalculatingStrategyType type) {
this.strategy = type;
}
public void initStrategy() {
if (this.getStrategyType() == null) {
this.strategy = CalculatingStrategyType.VOLUME;
}
}
public void setOptStrategy(CalculatingStrategyType type) {
if (type != null) {
this.strategy = type;
}
}
public Resource getResource() {
return resource;
}
public Resource getSecondaryResource() {
if (strategyData != null && strategyData.getSecondary() != null) {
return strategyData.getSecondary();
}
return null;
}
public Resource getBillableResource() {
if (getSecondaryResource() != null) {
return getSecondaryResource();
}
return getResource();
}
public void setResource(Resource resource) {
this.resource = resource;
}
public void setOptResource(Resource resource) {
if (resource != null) {
this.resource = resource;
}
}
public ChargeScope getChargeScope() {
if (billableResourceScope == freeResourceScope) {
return billableResourceScope;
}
return ChargeScope.ENVIRONMENT;
}
public void setChargeScope(ChargeScope chargeScope) {
this.billableResourceScope = chargeScope;
this.freeResourceScope = chargeScope;
}
public ChargeScope getFreeResourceScope() {
return freeResourceScope;
}
public void setFreeResourceScope(ChargeScope freeResourceScope) {
this.freeResourceScope = freeResourceScope;
}
public ChargeScope getBillableResourceScope() {
return billableResourceScope;
}
public void setBillableResourceScope(ChargeScope billableResourceScope) {
this.billableResourceScope = billableResourceScope;
}
public CalculatingStrategyData getStrategyData() {
return strategyData;
}
public void setStrategyData(CalculatingStrategyData strategyData) {
this.strategyData = strategyData;
}
public boolean isSecondaryResourceExist() {
return getStrategyData() != null && getStrategyData().getSecondary() != null;
}
public Currency getCurrency() {
return currency;
}
public void setCurrency(Currency currency) {
this.currency = currency;
}
public String getCurrencyISO() {
return currencyISO;
}
public void setCurrencyISO(String currencyISO) {
this.currencyISO = currencyISO;
}
public BigDecimal getMinimumFee() {
return minimumFee;
}
public void setMinimumFee(BigDecimal minimumFee) {
this.minimumFee = minimumFee;
}
public void setOptMinimumFee(BigDecimal minimumFee) {
if (minimumFee != null) {
this.minimumFee = minimumFee;
}
}
public BigDecimal getConvertedMinimumFee() {
return convertedMinimumFee;
}
public void setConvertedMinimumFee(BigDecimal convertedMinimumFee) {
this.convertedMinimumFee = convertedMinimumFee;
}
public Double getMinimumFeeResourceAmount() {
return minimumFeeResourceAmount;
}
public void setMinimumFeeResourceAmount(Double minimumFeeResourceAmount) {
this.minimumFeeResourceAmount = minimumFeeResourceAmount;
}
public void setOptMinimumFeeResourceAmount(Double minimumFeeResourceAmount) {
if (minimumFeeResourceAmount != null) {
this.minimumFeeResourceAmount = minimumFeeResourceAmount;
}
}
public String getDocUrl() {
return docUrl;
}
public void nullifyFields() {
if (StringUtils.isEmpty(this.docUrl)) {
this.docUrl = null;
}
}
public String getBillingHistoryName() {
return tariffGridItem != null ? tariffGridItem.getDisplayName() : null;
}
public TariffGrid getTariffGrid() {
return tariffGrid;
}
public void setTariffGrid(TariffGrid tariffGrid) {
this.tariffGrid = tariffGrid;
}
public TariffGridItem getTariffGridItem() {
return tariffGridItem;
}
public void setTariffGridItem(TariffGridItem tariffGridItem) {
this.tariffGridItem = tariffGridItem;
}
public boolean isInArray(Set array) {
for (TariffPlan tariffPlan : array) {
if (tariffPlan.getId() == this.id) {
return true;
}
}
return false;
}
public void setDocUrl(String docUrl) {
this.docUrl = docUrl;
}
public PriceType getPriceType() {
return priceType;
}
public void setPriceType(PriceType priceType) {
this.priceType = priceType;
}
public RecurringInterval getRecurringInterval() {
return recurringInterval;
}
public void setRecurringInterval(RecurringInterval recurringInterval) {
this.recurringInterval = recurringInterval;
}
public int getRecurringIntervalCount() {
return recurringIntervalCount;
}
public void setRecurringIntervalCount(int recurringIntervalCount) {
this.recurringIntervalCount = recurringIntervalCount;
}
public String getSku() {
return sku;
}
public void setSku(String sku) {
this.sku = sku;
}
@Override
public TariffPlan clone() {
TariffPlan tariffPlan = new TariffPlan();
tariffPlan.setKeyword(this.keyword);
tariffPlan.setUniqueName(this.uniqueName);
tariffPlan.setName(this.name);
tariffPlan.setDescription(this.description);
tariffPlan.setCloudletIncrement(this.cloudletIncrement);
tariffPlan.setMinCloudletCount(this.minCloudletCount);
tariffPlan.setType(this.type);
tariffPlan.setCreatedOn(new Date());
if (this.overridesTypes != null) {
tariffPlan.setOverridesTypes(new ArrayList<>(this.overridesTypes));
}
Set tiers = new HashSet();
for (Tier tier : this.tiers) {
tiers.add(tier.clone());
}
tariffPlan.setTiers(tiers);
tariffPlan.setReseller(this.reseller);
tariffPlan.strategy = this.strategy;
if (this.strategyData != null) {
tariffPlan.strategyData = this.strategyData.clone();
}
tariffPlan.setResource(this.getResource().clone());
tariffPlan.setChargeScope(this.getChargeScope());
tariffPlan.setOptChargeOnlyRunning(this.chargeOnlyRunning);
tariffPlan.setMinimumFee(this.getMinimumFee());
tariffPlan.setMinimumFeeResourceAmount(this.getMinimumFeeResourceAmount());
tariffPlan.setCurrency(this.getCurrency());
tariffPlan.setDocUrl(this.getDocUrl());
tariffPlan.setPriceType(this.getPriceType());
tariffPlan.setRecurringInterval(this.getRecurringInterval());
tariffPlan.setRecurringIntervalCount(this.getRecurringIntervalCount());
return tariffPlan;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
if (!super.equals(o))
return false;
TariffPlan that = (TariffPlan) o;
if (billingPeriod != that.billingPeriod)
return false;
if (Double.compare(that.capacityPrice, capacityPrice) != 0)
return false;
if (cloudletIncrement != that.cloudletIncrement)
return false;
if (Double.compare(that.cloudletPrice, cloudletPrice) != 0)
return false;
if (freeCapacity != that.freeCapacity)
return false;
if (freeCloudlet != that.freeCloudlet)
return false;
if (isDeleted != that.isDeleted)
return false;
if (minCloudletCount != that.minCloudletCount)
return false;
if (pricePeriod != that.pricePeriod)
return false;
if (createdOn != null ? !createdOn.equals(that.createdOn) : that.createdOn != null)
return false;
if (description != null ? !description.equals(that.description) : that.description != null)
return false;
if (keyword != null ? !keyword.equals(that.keyword) : that.keyword != null)
return false;
if (name != null ? !name.equals(that.name) : that.name != null)
return false;
if (type != that.type)
return false;
if (uniqueName != null ? !uniqueName.equals(that.uniqueName) : that.uniqueName != null)
return false;
if (updatedOn != null ? !updatedOn.equals(that.updatedOn) : that.updatedOn != null)
return false;
if (!Objects.equals(sku, that.sku))
return false;
return true;
}
@Override
public int hashCode() {
int result = super.hashCode();
long temp;
result = 31 * result + (uniqueName != null ? uniqueName.hashCode() : 0);
result = 31 * result + (keyword != null ? keyword.hashCode() : 0);
result = 31 * result + (name != null ? name.hashCode() : 0);
result = 31 * result + (description != null ? description.hashCode() : 0);
result = 31 * result + (type != null ? type.hashCode() : 0);
temp = cloudletPrice != +0.0d ? Double.doubleToLongBits(cloudletPrice) : 0L;
result = 31 * result + (int) (temp ^ (temp >>> 32));
temp = capacityPrice != +0.0d ? Double.doubleToLongBits(capacityPrice) : 0L;
result = 31 * result + (int) (temp ^ (temp >>> 32));
result = 31 * result + freeCapacity;
result = 31 * result + freeCloudlet;
result = 31 * result + pricePeriod;
result = 31 * result + billingPeriod;
result = 31 * result + (createdOn != null ? createdOn.hashCode() : 0);
result = 31 * result + (updatedOn != null ? updatedOn.hashCode() : 0);
result = 31 * result + (isDeleted ? 1 : 0);
result = 31 * result + minCloudletCount;
result = 31 * result + cloudletIncrement;
result = 31 * result + (sku != null ? sku.hashCode() : 0);
return result;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(UNIQUE_NAME, uniqueName);
json.put("id", this.id);
json.put("keyword", this.keyword);
json.put("name", this.name);
json.put("description", this.description);
json.put("type", this.type);
if (this.createdOn != null) {
json.put("createdOn", DateUtils.formatSqlDateTime(this.createdOn));
}
if (this.updatedOn != null) {
json.put("updatedOn", DateUtils.formatSqlDateTime(this.updatedOn));
}
if (tiers != null && !tiers.isEmpty()) {
JSONArray jsonArray = new JSONArray();
List sortedTiers = tiers.stream().sorted(Comparator.comparingDouble(Tier::getValue)).collect(Collectors.toList());
for (Tier selectedTier : sortedTiers) {
jsonArray.put(selectedTier._toJSON());
}
json.put("tiers", jsonArray);
}
json.put("minCloudletCount", minCloudletCount);
json.put("cloudletIncrement", cloudletIncrement);
if (this.resource != null) {
json.put("resource", resource._toJSON());
}
if (this.strategy != null) {
json.put("strategy", strategy);
}
if (this.strategyData != null) {
json.put("strategyData", strategyData._toJSON());
}
json.put("freeResourceScope", this.freeResourceScope);
json.put("billableResourceScope", this.billableResourceScope);
json.put("chargeOnlyRunning", chargeOnlyRunning);
if (this.currency != null) {
json.put(CURRENCY, currency._toJSON());
}
if (this.reseller != null) {
json.put(RESELLER, reseller._toJSON());
}
if (this.baseCurrency != null) {
json.put("baseCurrency", baseCurrency);
}
json.put(MINIMUM_FEE, getMinimumFee());
json.put(CONVERTED_MINIMUM_FEE, getConvertedMinimumFee());
json.put(MINIMUM_FEE_RESOURCE_AMOUNT, getMinimumFeeResourceAmount());
json.put(DOC_URL, getDocUrl());
if (overridesTypes != null && !overridesTypes.isEmpty()) {
JSONArray jsonArray = new JSONArray();
for (TariffPlanType overridesType : overridesTypes) {
jsonArray.put(overridesType.toString());
}
json.put(OVERRIDES_TYPES, jsonArray);
}
if (recurringInterval != null) {
json.put(RECURRING_INTERVAL, recurringInterval.toString());
}
if (sku != null) {
json.put(SKU, sku);
}
return json;
}
public Boolean isChargeOnlyRunning() {
return chargeOnlyRunning;
}
public void setChargeOnlyRunning(Boolean chargeOnlyRunning) {
this.chargeOnlyRunning = chargeOnlyRunning;
}
public void initChargeOnlyRunning() {
if (this.chargeOnlyRunning == null) {
this.chargeOnlyRunning = false;
}
}
public void setOptChargeOnlyRunning(Boolean chargeOnlyRunning) {
if (chargeOnlyRunning != null) {
this.chargeOnlyRunning = chargeOnlyRunning;
}
}
@Override
public TariffPlan _fromJSON(JSONObject json) throws JSONException {
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has(UNIQUE_NAME)) {
this.uniqueName = json.getString(UNIQUE_NAME);
}
if (json.has("keyword")) {
this.keyword = json.getString("keyword");
}
if (json.has("name")) {
this.name = json.getString("name");
}
if (json.has("description")) {
this.description = json.getString("description");
}
if (json.has("type")) {
this.type = TariffPlanType.valueOf(json.getString("type").toUpperCase());
}
if (json.has("createdOn")) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString("createdOn"));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has("updatedOn")) {
try {
this.updatedOn = DateUtils.parseSqlDateTime(json.getString("updatedOn"));
} catch (ParseException ex) {
ex.printStackTrace();
}
}
if (json.has("tiers")) {
tiers = new HashSet();
JSONArray jsonArray = json.getJSONArray("tiers");
for (int i = 0; i < jsonArray.length(); i++) {
Tier tier = new Tier()._fromJSON(jsonArray.getJSONObject(i));
tiers.add(tier);
}
}
if (json.has("minCloudletCount")) {
minCloudletCount = json.getInt("minCloudletCount");
}
if (json.has("cloudletIncrement")) {
cloudletIncrement = json.getInt("cloudletIncrement");
}
if (json.has("resource")) {
Object object = json.get("resource");
if (object instanceof String) {
resource = new Resource((String) object, null, null);
} else {
resource = new Resource()._fromJSON(json.getJSONObject("resource"));
}
}
if (json.has("strategy")) {
strategy = CalculatingStrategyType.valueOf(json.getString("strategy").toUpperCase());
}
if (json.has("strategyData")) {
strategyData = (CalculatingStrategyData) new CalculatingStrategyData()._fromJSON(json.getJSONObject("strategyData"));
}
if (json.has("chargeScope")) {
setChargeScope(ChargeScope.valueOf(json.getString("chargeScope").toUpperCase()));
}
if (json.has("billableResourceScope")) {
setBillableResourceScope(ChargeScope.valueOf(json.getString("billableResourceScope").toUpperCase()));
}
if (json.has("freeResourceScope")) {
setFreeResourceScope(ChargeScope.valueOf(json.getString("freeResourceScope").toUpperCase()));
}
if (json.has("chargeOnlyRunning")) {
this.chargeOnlyRunning = json.getBoolean("chargeOnlyRunning");
}
if (json.has(CURRENCY)) {
Object data = json.get(CURRENCY);
if (data instanceof String) {
currencyISO = json.getString(CURRENCY);
} else {
currency = (Currency) new Currency()._fromJSON(json.getJSONObject(CURRENCY));
currencyISO = currency.getCode();
}
}
if (json.has(MINIMUM_FEE)) {
this.minimumFee = BigDecimal.valueOf(json.getDouble(MINIMUM_FEE));
}
if (json.has(CONVERTED_MINIMUM_FEE)) {
this.convertedMinimumFee = BigDecimal.valueOf(json.getDouble(CONVERTED_MINIMUM_FEE));
}
if (json.has(MINIMUM_FEE_RESOURCE_AMOUNT)) {
this.minimumFeeResourceAmount = json.getDouble(MINIMUM_FEE_RESOURCE_AMOUNT);
}
if (json.has(DOC_URL)) {
this.docUrl = json.getString(DOC_URL);
}
if (json.has(OVERRIDES_TYPES)) {
this.overridesTypes = new ArrayList<>();
JSONArray jsonArray = json.getJSONArray(OVERRIDES_TYPES);
for (int i = 0; i < jsonArray.length(); i++) {
TariffPlanType overridesType = TariffPlanType.valueOf(jsonArray.getString(i));
overridesTypes.add(overridesType);
}
}
if (json.has(RECURRING_INTERVAL)) {
this.recurringInterval = RecurringInterval.valueOf(json.getString(RECURRING_INTERVAL));
}
if (json.has(SKU)) {
this.sku = json.getString(SKU);
}
return this;
}
public boolean hasConvertedFee() {
return this.getConvertedMinimumFee() != null && this.getConvertedMinimumFee().compareTo(BigDecimal.ZERO) > 0;
}
public boolean hasFee() {
return this.getMinimumFee() != null && this.getMinimumFee().compareTo(BigDecimal.ZERO) > 0;
}
public boolean hasSecondary() {
return this.getStrategyData() != null && this.getStrategyData().getSecondary() != null;
}
public List getOverridesTypes() {
return overridesTypes;
}
public void setOverridesTypes(List overridesTypes) {
this.overridesTypes = overridesTypes;
}
public void setOptOverridesTypes(List overridesTypes) {
if (overridesTypes != null) {
this.overridesTypes = overridesTypes;
}
}
public void overrideFrom(TariffPlan tariff) {
this.setName(tariff.getName());
this.setDescription(tariff.getDescription());
this.setMinCloudletCount(tariff.getMinCloudletCount());
this.setCloudletIncrement(tariff.getCloudletIncrement());
this.setTiers(tariff.getTiers());
this.setFreeResourceScope(tariff.getFreeResourceScope());
this.setBillableResourceScope(tariff.getBillableResourceScope());
this.setDocUrl(tariff.getDocUrl());
this.setOptResource(tariff.getResource());
this.setOptChargeOnlyRunning(tariff.isChargeOnlyRunning());
this.setOptMinimumFee(tariff.getMinimumFee());
this.setOptMinimumFeeResourceAmount(tariff.getMinimumFeeResourceAmount());
this.setOptStrategy(tariff.getStrategyType());
this.setOptOverridesTypes(tariff.getOverridesTypes());
this.setRecurringInterval(tariff.getRecurringInterval());
this.setRecurringIntervalCount(tariff.getRecurringIntervalCount());
this.setSku(tariff.getSku());
}
@Override
public String toString() {
return "TariffPlan{" + "keyword='" + keyword + '\'' + ", name='" + name + '\'' + ", type=" + type + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy