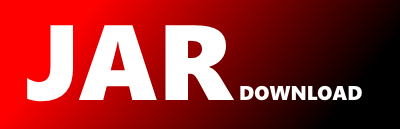
com.jelastic.api.system.persistence.Template Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 7d384d3e191a8baddcaaa951a32e9897*/
package com.jelastic.api.system.persistence;
import com.google.gson.Gson;
import com.jelastic.api.common.annotation.Transform;
import com.jelastic.api.common.Constants;
import com.jelastic.api.core.utils.StringUtils;
import com.jelastic.api.data.po.VType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.*;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Template extends ArrayItem {
public static final String TEMPLATE_COLUMN = "template";
public static final String CARTRIDGE_MANIFEST_KEY = "manifest";
public static final String IMAGE_16_URL_KEY = "image16Url";
public static final String IMAGE_32_URL_KEY = "image32Url";
public static final String IMAGE_64_URL_KEY = "image64Url";
public static final String ID = "id";
public static final String NODE_TYPE = "nodeType";
public static final String DESCRIPTION = "description";
public static final String NAME = "name";
public static final String NODE_MISSION = "nodeMission";
public static final String ENGINES = "engines";
public static final String HN = "hn";
public static final String VERSION = "version";
public static final String TYPE = "type";
public static final String IMAGES_DATA = "imagesData";
public static final String ENABLED = "enabled";
public static final String PUBLISHED = "published";
public static final String MANIFEST_URL = "manifestUrl";
public static final String OS_TYPE = "osType";
public static final String HOST_OS_TYPE = "hostOSType";
public static final String GUEST_OS_TYPE = "guestOSType";
public static final String IS_EXTERNAL_IP_REQUIRED = "isExternalIpRequired";
public static final String REPOSITORY = "repository";
public static final String REGISTRY = "registry";
public static final String TAGS = "tags";
public static final String ENGINE_TYPE = "engineType";
public static final String REGISTRY_ID = "registryId";
public static final String AUTO_UPDATE = "autoUpdate";
public static final String KEEP_SELECTED_TAGS = "keepSelectedTags";
public static final String UPDATE_DEFAULT_TAG = "updateDefaultTag";
public static final String SCALING_MODE = "scalingMode";
public static final String NODE_TYPE_ALIASES = "nodeTypeAliases";
public static final String V_TYPE = "vType";
public static final String MIN_DISK_LIMIT = "minDiskLimit";
public static final String DISTRIBUTION = "distribution";
public static final String AVAILABLE_REGIONS = "availableRegions";
public static final String CUSTOM_DATA = "customData";
protected HardwareNode hn;
protected boolean cartridge;
protected String version;
private String nodeType;
private String description;
@Transform(skip = true)
private OsNode osNode;
private String name;
private String nodeMission;
private String packageName;
private String appTemplate;
private String os;
private String features;
private String config;
private List engines = new ArrayList<>();
private boolean enabled = true;
private String imagesData;
private TemplateType type;
private String customData;
private boolean published = true;
private OSType hostOSType = OSType.LINUX;
private OSType guestOSType = OSType.LINUX;
private VType vType = VType.CT;
private Boolean isExternalIpRequired;
private String repository;
@Transform(skip = true)
private TemplateRegistry registry;
private Set tags = new HashSet<>();
private String engineType;
private String distribution;
private boolean userViewMode;
private boolean autoUpdate;
private boolean keepSelectedTags;
private boolean updateDefaultTag;
private Integer minDiskLimit;
private int registryId;
private Set availableRegions = new HashSet<>();
private Set supportedFeatures = new HashSet<>();
public Template(String nodeType, String description, OsNode templateNode) {
this.nodeType = nodeType;
this.description = description;
this.osNode = templateNode;
}
public Template() {
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getNodeType() {
return nodeType;
}
public void setNodeType(String nodeType) {
this.nodeType = nodeType;
}
public OsNode getOsNode() {
return osNode;
}
public void setOsNode(OsNode osNode) {
this.osNode = osNode;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNodeMission() {
return nodeMission;
}
public void setNodeMission(String nodeMission) {
this.nodeMission = nodeMission;
}
public List getEngines() {
return engines;
}
public void setEngines(List engines) {
this.engines = engines;
}
public HardwareNode getHn() {
return hn;
}
public void setHn(HardwareNode hn) {
this.hn = hn;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getPackageName() {
return packageName;
}
public void setPackageName(String packageName) {
this.packageName = packageName;
}
public String getOs() {
return os;
}
public void setOs(String os) {
this.os = os;
}
public String getConfig() {
return config;
}
public void setConfig(String config) {
this.config = config;
}
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public boolean isCartridge() {
return cartridge;
}
public void setCartridge(boolean cartridge) {
this.cartridge = cartridge;
}
public String getImagesData() {
return imagesData;
}
public void setImagesData(String imagesData) {
this.imagesData = imagesData;
}
public boolean isPublished() {
return published;
}
public void setPublished(boolean published) {
this.published = published;
}
public void setImagesDataMap(Map imagesDataMap) {
Gson gson = new Gson();
this.imagesData = gson.toJson(imagesDataMap);
}
public Map getImagesDataMap() {
if (imagesData == null || imagesData.isEmpty()) {
return new HashMap<>();
}
Gson gson = new Gson();
Map imagesDataMap = new HashMap();
imagesDataMap = (Map) gson.fromJson(imagesData, imagesDataMap.getClass());
return imagesDataMap;
}
public TemplateType getType() {
return type;
}
public void setType(TemplateType type) {
this.type = type;
}
public String getCustomData() {
return customData;
}
public void setCustomData(String customData) {
this.customData = customData;
}
public OSType getHostOSType() {
return hostOSType;
}
public void setHostOSType(OSType osType) {
this.hostOSType = osType;
}
public String getAppTemplate() {
return appTemplate;
}
public void setAppTemplate(String appTemplate) {
this.appTemplate = appTemplate;
}
public String getFeatures() {
return features;
}
public void setFeatures(String features) {
this.features = features;
}
public VType getVType() {
return vType;
}
public void setVType(VType vType) {
this.vType = vType;
}
public Map getCustomDataMap() {
if (customData == null || customData.isEmpty()) {
return new HashMap();
}
switch(type) {
case CARTRIDGE:
Gson gson = new Gson();
Map customDataMap = new HashMap();
customDataMap = (Map) gson.fromJson(customData, customDataMap.getClass());
return customDataMap;
}
return null;
}
public void setCustomDataMap(Map customDataMap) {
switch(type) {
case CARTRIDGE:
Gson gson = new Gson();
this.customData = gson.toJson(customDataMap);
break;
}
}
public String getCartridgeManifest() {
return getCustomDataMap().get(CARTRIDGE_MANIFEST_KEY);
}
public void setCartridgeManifest(String manifest) {
Map customDataMap = getCustomDataMap();
customDataMap.put(CARTRIDGE_MANIFEST_KEY, manifest);
setCustomDataMap(customDataMap);
}
public void setIsExternalIpRequired(Boolean isExternalIpRequired) {
this.isExternalIpRequired = isExternalIpRequired;
}
public String getRepository() {
return repository;
}
public void setRepository(String repository) {
this.repository = repository;
}
public TemplateRegistry getRegistry() {
return registry;
}
public void setRegistry(TemplateRegistry registry) {
this.registry = registry;
}
public Set getTags() {
return tags;
}
public Set getNotDeletedTags() {
Set notDeletedTags = new HashSet<>();
for (TemplateTag tag : tags) {
if (tag.isDeleted()) {
continue;
}
notDeletedTags.add(tag);
}
return notDeletedTags;
}
public void setTags(Set tags) {
this.tags = tags;
}
public String getEngineType() {
return engineType;
}
public void setEngineType(String engineType) {
this.engineType = engineType;
}
public boolean isUserViewMode() {
return userViewMode;
}
public void setUserViewMode(boolean userViewMode) {
this.userViewMode = userViewMode;
}
public boolean isAutoUpdate() {
return autoUpdate;
}
public void setAutoUpdate(boolean autoUpdate) {
this.autoUpdate = autoUpdate;
}
public boolean isKeepSelectedTags() {
return keepSelectedTags;
}
public void setKeepSelectedTags(boolean keepSelectedTags) {
this.keepSelectedTags = keepSelectedTags;
}
public boolean isUpdateDefaultTag() {
return updateDefaultTag;
}
public void setUpdateDefaultTag(boolean updateDefaultTag) {
this.updateDefaultTag = updateDefaultTag;
}
public Set getSupportedFeatures() {
return supportedFeatures;
}
public void setSupportedFeatures(Set supportedFeatures) {
this.supportedFeatures = supportedFeatures;
}
public int getRegistryId() {
return registryId;
}
public void setRegistryId(int registryId) {
this.registryId = registryId;
}
public OSType getGuestOSType() {
return guestOSType;
}
public void setGuestOSType(OSType guestOsType) {
this.guestOSType = guestOsType;
}
public TemplateTag getDefaultTag() {
if (tags == null || tags.isEmpty()) {
return null;
}
for (TemplateTag tag : tags) {
if (tag.isDefault()) {
return tag;
}
}
return null;
}
public ScalingMode getScalingMode() {
return ScalingMode.getDefaultByNodeMission(nodeMission);
}
public Integer getMinDiskLimit() {
return minDiskLimit;
}
public void setMinDiskLimit(Integer minDiskLimit) {
this.minDiskLimit = minDiskLimit;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NODE_TYPE, nodeType);
json.put(DESCRIPTION, description);
json.put(NAME, name);
json.put(NODE_MISSION, nodeMission);
json.put(V_TYPE, vType.toString());
if (engines != null && !engines.isEmpty()) {
JSONArray array = new JSONArray();
for (Engine engine : engines) {
array.put(engine._toJSON());
}
json.put(ENGINES, array);
}
if (hn != null) {
json.put(HN, hn._toJSON());
}
json.put(VERSION, version);
json.put(TYPE, this.type != null ? this.type.toString() : null);
if (imagesData != null) {
json.put(IMAGES_DATA, new JSONObject(imagesData));
}
json.put(ENABLED, enabled);
json.put(PUBLISHED, published);
if (isExternalIpRequired == null) {
if (osNode != null) {
SoftwareNode node = osNode.getSoftNode();
if (node != null) {
isExternalIpRequired = node.getProperties().isExternalIpRequired();
}
}
}
json.put(IS_EXTERNAL_IP_REQUIRED, isExternalIpRequired);
if (hostOSType != null) {
json.put(HOST_OS_TYPE, hostOSType.toString());
}
if (guestOSType != null) {
json.put(GUEST_OS_TYPE, guestOSType.toString());
}
if (!userViewMode) {
json.put(REPOSITORY, repository);
if (registry != null) {
json.put(REGISTRY_ID, registry.getId());
} else if (registryId > 0) {
json.put(REGISTRY_ID, registryId);
}
json.put(AUTO_UPDATE, autoUpdate);
json.put(KEEP_SELECTED_TAGS, keepSelectedTags);
json.put(UPDATE_DEFAULT_TAG, updateDefaultTag);
}
if (!tags.isEmpty()) {
Set nodeTypeAliases = new HashSet<>();
JSONArray tagsArray = new JSONArray();
for (TemplateTag tag : tags) {
if (StringUtils.isNotBlank(tag.getNodeTypeAlias())) {
nodeTypeAliases.addAll(Arrays.asList(tag.getNodeTypeAlias().split(Constants.SEMICOLON_SEPARATOR)));
}
if (userViewMode && !tag.isEnabled()) {
continue;
}
tagsArray.put(tag._toJSON());
}
json.put(TAGS, tagsArray);
if (nodeTypeAliases.size() > 0) {
json.put(NODE_TYPE_ALIASES, nodeTypeAliases);
}
}
json.put(ENGINE_TYPE, engineType);
json.put(SCALING_MODE, getScalingMode());
if (minDiskLimit != null) {
json.put(MIN_DISK_LIMIT, minDiskLimit);
}
if (!userViewMode) {
if (this.distribution != null) {
json.put(DISTRIBUTION, this.distribution);
}
}
JSONArray regions = new JSONArray();
for (String availableRegion : availableRegions) {
regions.put(availableRegion);
}
json.put(AVAILABLE_REGIONS, regions);
return json;
}
@Override
public Template _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NODE_TYPE)) {
this.nodeType = json.getString(NODE_TYPE);
}
if (json.has(DESCRIPTION)) {
this.description = json.getString(DESCRIPTION);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(NODE_MISSION)) {
this.nodeMission = json.getString(NODE_MISSION);
}
if (json.has(ENGINES)) {
engines = new ArrayList();
JSONArray array = json.getJSONArray(ENGINES);
for (int i = 0; i < array.length(); i++) {
engines.add(new Engine()._fromJSON(array.getJSONObject(i)));
}
}
if (json.has(HN)) {
this.hn = new HardwareNode()._fromJSON(json.getJSONObject(HN));
}
if (json.has(TYPE)) {
try {
this.type = TemplateType.valueOf(TemplateType.class, json.getString(TYPE).toUpperCase());
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
if (json.has(IMAGES_DATA)) {
this.imagesData = json.getJSONObject(IMAGES_DATA).toString();
}
if (json.has(VERSION)) {
this.version = json.getString(VERSION);
}
if (json.has(ENABLED)) {
this.enabled = json.getBoolean(ENABLED);
}
if (json.has(PUBLISHED)) {
this.published = json.getBoolean(PUBLISHED);
}
if (json.has(OS_TYPE)) {
hostOSType = OSType.valueOf(OSType.class, json.getString(OS_TYPE));
}
if (json.has(HOST_OS_TYPE)) {
hostOSType = OSType.valueOf(OSType.class, json.getString(HOST_OS_TYPE));
}
if (json.has(GUEST_OS_TYPE)) {
guestOSType = OSType.valueOf(OSType.class, json.getString(GUEST_OS_TYPE));
}
if (json.has(REGISTRY)) {
this.registry = new TemplateRegistry()._fromJSON(json.getJSONObject(REGISTRY));
}
if (json.has(REGISTRY_ID)) {
this.registryId = json.getInt(REGISTRY_ID);
}
if (json.has(TAGS)) {
tags = new HashSet<>();
JSONArray tagsArray = json.getJSONArray(TAGS);
for (int i = 0; i < tagsArray.length(); i++) {
tags.add(new TemplateTag()._fromJSON(tagsArray.getJSONObject(i)));
}
}
if (json.has(ENGINE_TYPE)) {
this.engineType = json.getString(ENGINE_TYPE);
}
if (json.has(REPOSITORY)) {
repository = json.getString(REPOSITORY);
}
if (json.has(ENGINE_TYPE)) {
this.engineType = json.getString(ENGINE_TYPE);
}
if (json.has(AUTO_UPDATE)) {
this.autoUpdate = json.getBoolean(AUTO_UPDATE);
}
if (json.has(KEEP_SELECTED_TAGS)) {
this.keepSelectedTags = json.getBoolean(KEEP_SELECTED_TAGS);
}
if (json.has(UPDATE_DEFAULT_TAG)) {
this.updateDefaultTag = json.getBoolean(UPDATE_DEFAULT_TAG);
}
if (json.has(V_TYPE)) {
this.vType = VType.valueOf(json.getString(V_TYPE));
}
if (json.has(MIN_DISK_LIMIT)) {
this.minDiskLimit = json.getInt(MIN_DISK_LIMIT);
}
if (json.has(DISTRIBUTION)) {
this.distribution = json.getString(DISTRIBUTION);
}
if (json.has(AVAILABLE_REGIONS)) {
JSONArray regions = json.getJSONArray(AVAILABLE_REGIONS);
this.availableRegions.clear();
for (int i = 0; i < regions.length(); i++) {
this.availableRegions.add(regions.getString(i));
}
}
return this;
}
public String getDistribution() {
return this.distribution;
}
public void setDistribution(String distribution) {
this.distribution = distribution;
}
public HWType getRequiredHWType() {
return this.getVType() == VType.VM ? HWType.BARE_METAL : null;
}
public Set getAvailableRegions() {
return availableRegions;
}
public void setAvailableRegions(Set availableRegions) {
this.availableRegions = availableRegions;
}
public boolean isTagExist(String tag) {
boolean isValidTag = false;
for (TemplateTag templateTag : this.getTags()) {
if (templateTag.getName().equals(tag)) {
isValidTag = true;
break;
}
}
return isValidTag;
}
}