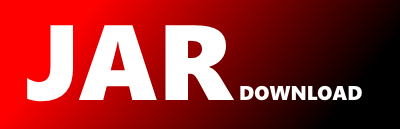
com.jelastic.api.system.persistence.TemplateRegistry Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 5491d9fa9b0caf1ef7dd8611435f2496*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TemplateRegistry extends ArrayItem {
public static final int MIN_PASSWORD_LENGTH = 6;
public static final String ID = "id";
public static final String URL = "url";
public static final String NAME = "name";
public static final String USER_NAME = "userName";
public static final String PASSWORD = "password";
public static final String IS_DEFAULT = "isDefault";
private String url;
private String userName;
private String password = "";
private String name;
private boolean isDefault;
private boolean isDeleted = false;
public TemplateRegistry() {
}
public TemplateRegistry(String url, String userName, String password) {
this.url = url;
this.userName = userName;
this.password = password;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public boolean isDefault() {
return isDefault;
}
public void setDefault(boolean aDefault) {
isDefault = aDefault;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(URL, url);
json.put(USER_NAME, userName);
json.put(NAME, name);
json.put(IS_DEFAULT, isDefault);
return json;
}
@Override
public TemplateRegistry _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(URL)) {
this.url = json.getString(URL);
}
if (json.has(USER_NAME)) {
this.userName = json.getString(USER_NAME);
}
if (json.has(PASSWORD)) {
this.password = json.getString(PASSWORD);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(IS_DEFAULT)) {
this.isDefault = json.getBoolean(IS_DEFAULT);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy