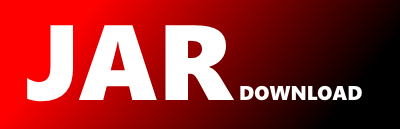
com.jelastic.api.system.persistence.Tier Maven / Gradle / Ivy
/*Server class MD5: a4471fe30139c3c90ca6040b3c58cdf0*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.annotation.Transform;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Tier extends ArrayItem implements Serializable {
private static final String VALUE = "value";
private static final String FREE = "free";
private static final String PRICE = "price";
private static final String CURRENCY_PRICE = "currencyPrice";
private static final String TRIAL_PERIOD = "trialPeriod";
@Transform(required = true)
private double value;
@Transform(required = true)
private double free;
@Transform(required = true)
private double price;
@Transform(required = true)
private Double currencyPrice;
private int trialPeriod;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getValue() {
return value;
}
public void setValue(double value) {
this.value = value;
}
public double getFree() {
return free;
}
public void setFree(double free) {
this.free = free;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Double getCurrencyPrice() {
return currencyPrice;
}
public void setCurrencyPrice(Double currencyPrice) {
this.currencyPrice = currencyPrice;
}
public int getTrialPeriod() {
return trialPeriod;
}
public void setTrialPeriod(int gracePeriod) {
this.trialPeriod = gracePeriod;
}
@Override
public Tier clone() {
Tier tier = new Tier();
tier.setValue(this.value);
tier.setPrice(this.price);
tier.setFree(this.free);
tier.setCurrencyPrice(this.currencyPrice);
tier.setTrialPeriod(this.trialPeriod);
return tier;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(VALUE, value);
json.put(FREE, free);
json.put(PRICE, price);
if (currencyPrice != null) {
json.put(CURRENCY_PRICE, currencyPrice);
}
json.put(TRIAL_PERIOD, trialPeriod);
return json;
}
@Override
public Tier _fromJSON(JSONObject json) throws JSONException {
if (json.has(VALUE)) {
value = json.getDouble(VALUE);
}
if (json.has(FREE)) {
free = json.getDouble(FREE);
}
if (json.has(PRICE)) {
price = json.getDouble(PRICE);
}
if (json.has(CURRENCY_PRICE)) {
currencyPrice = json.getDouble(CURRENCY_PRICE);
}
if (json.has(TRIAL_PERIOD)) {
trialPeriod = json.getInt(TRIAL_PERIOD);
}
return this;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
if (!super.equals(o))
return false;
Tier tier = (Tier) o;
if (Double.compare(tier.free, free) != 0)
return false;
if (Double.compare(tier.price, price) != 0)
return false;
if (Double.compare(tier.value, value) != 0)
return false;
return true;
}
@Override
public int hashCode() {
int result = super.hashCode();
long temp;
temp = value != +0.0d ? Double.doubleToLongBits(value) : 0L;
result = 31 * result + (int) (temp ^ (temp >>> 32));
temp = free != +0.0d ? Double.doubleToLongBits(free) : 0L;
result = 31 * result + (int) (temp ^ (temp >>> 32));
temp = price != +0.0d ? Double.doubleToLongBits(price) : 0L;
result = 31 * result + (int) (temp ^ (temp >>> 32));
return result;
}
@Override
public String toString() {
return "Tier{" + "value=" + value + ", free=" + free + ", price=" + price + ", currencyPrice=" + currencyPrice + ", id=" + id + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy