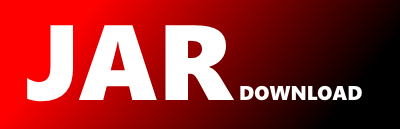
com.jelastic.api.system.persistence.TrackedAction Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.system.persistence;
import com.jelastic.api.system.po.ActionType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.Date;
/**
* @author Alexey Shponarsky
*/
public class TrackedAction extends ArrayItem implements Serializable {
public static String INSTANCE_KEY = null;
private Action action;
private String appid;
private String text;
private Integer uid;
private Date startTime;
private Date endTime;
private Integer result;
private String error;
private String actionMark;
private String args;
private ActionType actionType;
private String serviceName;
private String clientSource;
private String response;
private String requestUniqueKey;
private String instanceKey = INSTANCE_KEY;
public Action getAction() {
return action;
}
public void setAction(Action action) {
this.action = action;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
public Date getEndTime() {
return endTime;
}
public void setEndTime(Date endTime) {
this.endTime = endTime;
}
public Integer getResult() {
return result;
}
public void setResult(Integer result) {
this.result = result;
}
public String getError() {
return error;
}
public void setError(String error) {
this.error = error;
}
public Date getStartTime() {
return startTime;
}
public void setStartTime(Date satrtTime) {
this.startTime = satrtTime;
}
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public String getActionMark() {
return actionMark;
}
public void setActionMark(String actionMark) {
this.actionMark = actionMark;
}
public String getArgs() {
return args;
}
public void setArgs(String args) {
this.args = args;
}
public ActionType getActionType() {
return actionType;
}
public void setActionType(ActionType actionType) {
this.actionType = actionType;
}
public String getServiceName() {
return serviceName;
}
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
public String getClientSource() {
return clientSource;
}
public void setClientSource(String clientSource) {
this.clientSource = clientSource;
}
public String getResponse() {
return response;
}
public void setResponse(String response) {
this.response = response;
}
public String getRequestUniqueKey() {
return requestUniqueKey;
}
public void setRequestUniqueKey(String requestUniqueKey) {
this.requestUniqueKey = requestUniqueKey;
}
public String getInstanceKey() {
return instanceKey;
}
public void setInstanceKey(String instanceKey) {
this.instanceKey = instanceKey;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("id", id);
if (text != null) {
json.put("action", text);
}
if (uid != null) {
json.put("uid", uid);
}
if (result != null) {
json.put("result", result);
}
if (action != null) {
json.put("action", action.getId());
}
if (error != null) {
json.put("error", error);
}
if (text != null) {
json.put("text", new JSONObject(this.text));
}
if (actionMark != null) {
json.put("actionkey", this.actionMark);
}
if (serviceName != null) {
json.put("service_name", this.serviceName);
}
if (startTime != null) {
json.put("starttime", startTime.getTime());
if (endTime != null) {
long duration = endTime.getTime() - startTime.getTime();
json.put("duration", duration);
}
}
return json;
}
@Override
public TrackedAction _fromJSON(JSONObject json) throws JSONException {
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has("action")) {
action = new Action();
action.setId(json.getInt("action"));
}
if (json.has("result")) {
this.result = json.getInt("result");
}
if (json.has("uid")) {
this.uid = json.getInt("uid");
}
if (json.has("error")) {
this.error = json.getString("error");
}
if (json.has("starttime")) {
this.startTime = new Date(json.getLong("starttime"));
}
if (json.has("actionkey")) {
this.actionMark = json.getString("actionkey");
}
if (json.has("service_name")) {
this.serviceName = json.getString("service_name");
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy