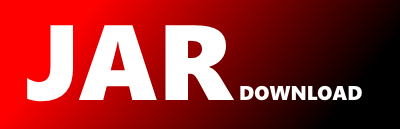
com.jelastic.api.system.persistence.Trigger Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 824e9e5830828e0cb81cb59c9f5e8275*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.data.po.VType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class Trigger extends ArrayItem implements Serializable {
public static final String ID = "id";
public static final String NAME = "name";
public static final String NODE_GROUP = "nodeGroup";
public static final String STATE = "state";
public static final String PERIOD = "period";
public static final String CONDITION = "condition";
public static final String IS_ENABLED = "isEnabled";
public static final String IS_DELETED = "isDeleted";
public static final String ACTIONS = "actions";
public static final String CREATED_ON = "createdOn";
public static final String UPDATED_ON = "updatedOn";
public static final String DELETED_ON = "deletedOn";
public static final String LAST_EXECUTED_ON = "lastExecutedOn";
public static final String V_TYPES = "vTypes";
public interface Add {
}
public interface Edit {
}
private String name;
private String nodeGroup;
private AppNodes appNode;
private TriggerState state;
private Integer period;
private TriggerCondition condition;
private boolean isEnabled = true;
private boolean isDeleted = false;
private Date createdOn;
private Date updatedOn;
private Date deletedOn;
private Date lastExecutedOn;
private Set actions = new HashSet();
private Date firstExecutionInSequence;
private Integer sequentialExecCount = 0;
private Set vTypes = new HashSet<>(Collections.singletonList(VType.CT));
public String getNodeGroup() {
return nodeGroup;
}
public void setNodeGroup(String nodeGroup) {
this.nodeGroup = nodeGroup;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public AppNodes getAppNode() {
return appNode;
}
public void setAppNode(AppNodes appNode) {
this.appNode = appNode;
}
public TriggerState getState() {
return state;
}
public void setState(TriggerState state) {
this.state = state;
}
public int getPeriod() {
return period;
}
public void setPeriod(int period) {
this.period = period;
}
public TriggerCondition getCondition() {
return condition;
}
public void setCondition(TriggerCondition condition) {
this.condition = condition;
}
public boolean isEnabled() {
return isEnabled;
}
public void setEnabled(boolean isEnabled) {
this.isEnabled = isEnabled;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public Date getDeletedOn() {
return deletedOn;
}
public void setDeletedOn(Date deletedOn) {
this.deletedOn = deletedOn;
}
public Date getLastExecutedOn() {
return lastExecutedOn;
}
public void setLastExecutedOn(Date lastExecutedOn) {
this.lastExecutedOn = lastExecutedOn;
}
public Set getActions() {
return actions;
}
public void setActions(Set actions) {
this.actions = actions;
}
public void setDefaultName() {
String resourceType = condition.getResourceType().toString().toLowerCase();
this.name = "auto_alert_" + resourceType;
}
public Date getFirstExecutionInSequence() {
return firstExecutionInSequence;
}
public void setFirstExecutionInSequence(Date firstExecutionInSequence) {
this.firstExecutionInSequence = firstExecutionInSequence;
}
public Integer getSequentialExecCount() {
return sequentialExecCount;
}
public void setSequentialExecCount(Integer sequentialExecCount) {
this.sequentialExecCount = sequentialExecCount;
}
public Set getvTypes() {
return vTypes;
}
public void setvTypes(Set vTypes) {
this.vTypes = vTypes;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put(ID, id);
json.put(NAME, name);
json.put(NODE_GROUP, nodeGroup);
json.put(STATE, state);
json.put(PERIOD, period);
if (condition != null) {
json.put(CONDITION, condition._toJSON());
}
json.put(IS_ENABLED, isEnabled);
json.put(IS_DELETED, isDeleted);
if (createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(createdOn));
}
if (updatedOn != null) {
json.put(UPDATED_ON, DateUtils.formatSqlDateTime(updatedOn));
}
if (deletedOn != null) {
json.put(DELETED_ON, DateUtils.formatSqlDateTime(deletedOn));
}
if (lastExecutedOn != null) {
json.put(LAST_EXECUTED_ON, DateUtils.formatSqlDateTime(lastExecutedOn));
}
if (actions != null) {
JSONArray actionsArray = new JSONArray();
for (TriggerAction action : actions) {
actionsArray.put(action._toJSON());
}
json.put(ACTIONS, actionsArray);
}
return json;
}
@Override
public Trigger _fromJSON(JSONObject json) throws JSONException {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(NODE_GROUP)) {
this.nodeGroup = json.getString(NODE_GROUP);
}
if (json.has(STATE)) {
this.state = TriggerState.valueOf(TriggerState.class, json.getString(STATE));
}
if (json.has(PERIOD)) {
this.period = json.getInt(PERIOD);
}
if (json.has(CONDITION)) {
this.condition = new TriggerCondition()._fromJSON(json.getJSONObject(CONDITION));
}
if (json.has(IS_ENABLED)) {
this.isEnabled = json.getBoolean(IS_ENABLED);
}
if (json.has(V_TYPES)) {
JSONArray vTypesArray = json.getJSONArray(V_TYPES);
for (int i = 0; i < vTypesArray.length(); i++) {
this.vTypes.add(VType.valueOf(vTypesArray.getString(i)));
}
}
if (json.has(ACTIONS)) {
actions = new HashSet();
JSONArray array = json.getJSONArray(ACTIONS);
for (int i = 0; i < array.length(); i++) {
actions.add(new TriggerAction()._fromJSON(array.getJSONObject(i)));
}
}
return this;
}
@Override
public String toString() {
try {
return this._toJSON().toString();
} catch (JSONException e) {
e.printStackTrace();
return null;
}
}
@Override
public Trigger clone() {
Trigger trigger = new Trigger();
trigger.setName(this.name);
trigger.setNodeGroup(this.nodeGroup);
trigger.setAppNode(this.appNode);
trigger.setState(TriggerState.IDLE);
trigger.setPeriod(this.period);
trigger.setCondition(this.condition.clone());
trigger.setEnabled(this.isEnabled);
trigger.setDeleted(this.isDeleted);
trigger.setCreatedOn(new Date());
trigger.setUpdatedOn(null);
trigger.setDeletedOn(this.deletedOn);
trigger.setLastExecutedOn(this.lastExecutedOn);
trigger.setFirstExecutionInSequence(this.firstExecutionInSequence);
trigger.setSequentialExecCount(this.sequentialExecCount);
Set clonedActions = new HashSet();
for (TriggerAction action : actions) {
TriggerAction clonedAction = action.clone();
clonedAction.setTrigger(trigger);
clonedActions.add(clonedAction);
}
trigger.setActions(clonedActions);
return trigger;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy