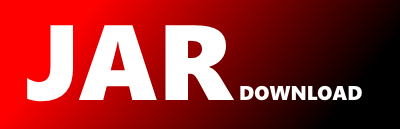
com.jelastic.api.system.persistence.TriggerAction Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 3782737b966c2de3a8e39229e6d4849d*/
package com.jelastic.api.system.persistence;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TriggerAction extends ArrayItem implements Serializable {
private Trigger trigger;
private TriggerActionType type;
private String customData;
public Trigger getTrigger() {
return trigger;
}
public void setTrigger(Trigger trigger) {
this.trigger = trigger;
}
public TriggerActionType getType() {
return type;
}
public void setType(TriggerActionType type) {
this.type = type;
}
public String getCustomData() {
return customData;
}
public void setCustomData(String customData) {
this.customData = customData;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
json.put("type", type);
if (customData != null) {
json.put("customData", new TriggerCustomData().fromString(customData)._toJSON());
}
return json;
}
@Override
public TriggerAction _fromJSON(JSONObject json) throws JSONException {
if (json.has("type")) {
this.type = TriggerActionType.valueOf(TriggerActionType.class, json.getString("type"));
}
if (json.has("customData")) {
this.customData = json.getString("customData");
}
return this;
}
@Override
protected TriggerAction clone() {
TriggerAction action = new TriggerAction();
action.setType(this.type);
action.setCustomData(this.customData);
return action;
}
}