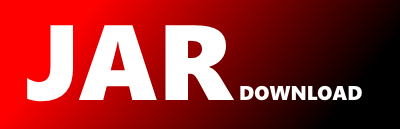
com.jelastic.api.system.persistence.pricing.TariffGrid Maven / Gradle / Ivy
The newest version!
/*Server class MD5: f66673c14eeb7f05263b64279c2313f8*/
package com.jelastic.api.system.persistence.pricing;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.annotation.Transform;
import org.json.JSONException;
import org.json.JSONObject;
import com.jelastic.api.system.persistence.*;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TariffGrid extends ArrayItem {
@Transform(skip = true)
public static final String NAME = "name";
@Transform(skip = true)
public static final String RESELLER = "reseller";
@Transform(required = true)
private String name;
@Transform(required = true)
private String displayName;
private String nodeType;
private TariffGridBillStrategy billStrategy = TariffGridBillStrategy.ALL;
private String description;
@Transform(sort = true)
private Set tariffItems = new HashSet<>();
@Transform(sort = true)
private Set items = new HashSet<>();
@Transform(skip = true)
private boolean isDeleted;
private Reseller reseller;
private Date createdOn;
private Date updatedOn;
public TariffGrid() {
}
public Reseller getReseller() {
return reseller;
}
public void setReseller(Reseller reseller) {
this.reseller = reseller;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNodeType() {
return nodeType;
}
public void setNodeType(String nodeType) {
this.nodeType = nodeType;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Set getTransientItems() {
return items;
}
public Set getTariffItems() {
return tariffItems;
}
public Set getItems() {
Set gridItems = new HashSet<>();
tariffItems.forEach(i -> {
TariffGridItem item = i.getTariffGridItem();
if (i.getTariffPlan() != null) {
item.setPlan(i.getTariffPlan());
}
gridItems.add(item);
});
return gridItems;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public void setItems(Set items) {
this.items = items;
}
public void setItems(List items) {
this.items = new HashSet<>(items);
}
public String getDisplayName() {
return displayName;
}
public TariffGridBillStrategy getBillStrategy() {
return billStrategy;
}
public void setBillStrategy(TariffGridBillStrategy billStrategy) {
this.billStrategy = billStrategy;
}
public void overrideFrom(TariffGrid grid, Set transientItems) {
this.setName(grid.getName() != null ? grid.getName() : this.getName());
this.setDisplayName(grid.getDisplayName() != null ? grid.getDisplayName() : this.getDisplayName());
this.setItems(transientItems);
this.setDescription(grid.getDescription() != null ? grid.getDescription() : this.description);
this.setNodeType(grid.getNodeType() != null ? grid.getNodeType() : this.nodeType);
this.setBillStrategy(grid.getBillStrategy() != null ? grid.getBillStrategy() : this.billStrategy);
}
@Override
public JSONObject _toJSON() throws JSONException {
Set tmpItems = getItems();
if (tmpItems != null && !tmpItems.isEmpty()) {
items = tmpItems;
}
return super._toJSON();
}
public JSONObject _toJSONWithTransientItems() throws JSONException {
return super._toJSON();
}
@Override
public String toString() {
return "TariffGrid{" + "name='" + name + '\'' + ", displayName='" + displayName + '\'' + ", nodeType='" + nodeType + '\'' + ", billStrategy=" + billStrategy + ", description='" + description + '\'' + ", items=" + getItems() + ", isDeleted=" + isDeleted + ", reseller=" + reseller + ", createdOn=" + createdOn + ", updatedOn=" + updatedOn + '}';
}
public TariffGrid clone() {
TariffGrid grid = new TariffGrid();
grid.setDescription(this.description);
grid.setName(this.name);
grid.setDisplayName(this.displayName);
grid.setNodeType(this.nodeType);
Set clonedItems = new HashSet<>();
for (TariffGridItem item : getItems()) {
clonedItems.add(item.clone());
}
grid.setItems(clonedItems);
return grid;
}
}