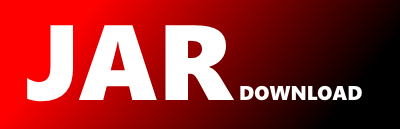
com.jelastic.api.system.persistence.pricing.TariffGridItem Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 2972d4175b06baffab1b3ebd07a82f22*/
package com.jelastic.api.system.persistence.pricing;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.Constants;
import com.jelastic.api.common.annotation.Transform;
import com.jelastic.api.system.persistence.Reseller;
import com.jelastic.api.system.persistence.TariffPlan;
import com.jelastic.api.utils.MD5;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TariffGridItem extends ArrayItem implements Comparable {
public static final String NAME = "name";
public static final String TARIFF_PLAN = "plan";
public static final String TARIFF_OPTIONS = "options";
public static final String RESELLER = "reseller";
@Transform(required = true)
private String name;
private String displayName;
private String description;
private Set options = new HashSet<>();
private TariffPlan plan;
@Transform(skip = true)
private boolean isDeleted;
private Reseller reseller;
private Date createdOn;
private Date updatedOn;
private Integer order;
public TariffGridItem() {
}
public Reseller getReseller() {
return reseller;
}
public void setReseller(Reseller reseller) {
this.reseller = reseller;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set getOptions() {
return options;
}
public void setOptions(Set options) {
this.options = options;
}
public void setOptions(List options) {
setOptions(new HashSet<>(options));
}
public TariffPlan getPlan() {
return plan;
}
public void setPlan(TariffPlan plan) {
this.plan = plan;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public Integer getOrder() {
return order;
}
public void setOrder(Integer order) {
this.order = order;
}
public void overrideFrom(TariffGridItem item) {
this.setName(item.getName() != null ? item.getName() : this.getName());
this.setDisplayName(item.getDisplayName() != null ? item.getDisplayName() : this.getDisplayName());
this.setOptions(item.getOptions());
this.setPlan(item.getPlan());
this.setDescription(item.getDescription() != null ? item.getDescription() : this.description);
this.setOrder(item.getOrder() != null ? item.getOrder() : this.order);
}
public JSONArray getNameAsJSONArray() {
JSONArray array = new JSONArray();
array.put(this.name);
return array;
}
public static JSONArray getNameAsJSONArray(String name) {
JSONArray array = new JSONArray();
array.put(name);
return array;
}
public TariffGridItem clone() {
TariffGridItem item = new TariffGridItem();
item.setDisplayName(this.displayName);
item.setName(this.name);
item.setDescription(this.description);
Set clonedOptions = new HashSet<>();
for (TariffOptionValue option : options) {
clonedOptions.add(option.clone());
}
item.setOptions(clonedOptions);
TariffPlan clonedPlan = new TariffPlan();
clonedPlan.setUniqueName(plan.getUniqueName());
item.setPlan(clonedPlan);
item.setOrder(this.order);
return item;
}
public String getOptionsAsString() {
SortedSet sorted = new TreeSet<>(options);
List ops = new ArrayList<>();
for (TariffOptionValue tariffOptionValue : sorted) {
ops.add(tariffOptionValue.getOptionValueAsString());
}
return String.join(Constants.COMMA_SEPARATOR, ops);
}
public String getMD5ID() {
return new MD5().get(getOptionsAsString());
}
public JSONObject getAsJSONObject() throws JSONException {
JSONObject object = new JSONObject();
object.put(NAME, this.name);
JSONObject optsJSONObject = new JSONObject();
for (TariffOptionValue optVal : options) {
optsJSONObject.put(optVal.getOption().getName(), optVal.getValue());
}
object.put(TARIFF_OPTIONS, optsJSONObject);
return object;
}
@Override
public int compareTo(TariffGridItem item) {
if (item.getOrder() == null && this.getOrder() == null) {
return 0;
} else if (this.order == null) {
return -1;
} else if (item.order == null) {
return 1;
} else {
return this.order.compareTo(item.getOrder());
}
}
public static TariffGridItem fromJSON(JSONObject json) throws JSONException {
TariffGridItem item = new TariffGridItem();
if (json.has(NAME)) {
item.setName(json.getString(NAME));
}
if (json.has(TARIFF_OPTIONS)) {
JSONObject jsonOptions = json.getJSONObject(TARIFF_OPTIONS);
Set options = new HashSet<>();
for (Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy