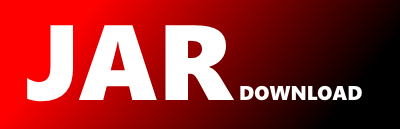
com.jelastic.api.system.persistence.pricing.TariffOptionValue Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 670a9ac02d6c9fa004ef1a7a9092f214*/
package com.jelastic.api.system.persistence.pricing;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.Constants;
import com.jelastic.api.common.annotation.Transform;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Date;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TariffOptionValue extends ArrayItem implements Comparable {
public static final String OPTION = "option";
public static final String VALUE = "value";
public static final String NAME = "name";
@Transform(required = true)
private String value;
private TariffOption option;
private Date createdOn;
private Date updatedOn;
@Transform(skip = true)
private boolean isDeleted;
public String getName() {
return this.getOption().getName();
}
public TariffOptionValue() {
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
public TariffOption getOption() {
return option;
}
public void setOption(TariffOption option) {
this.option = option;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public boolean isDeleted() {
return isDeleted;
}
public void setDeleted(boolean deleted) {
isDeleted = deleted;
}
public TariffOptionValue fromString(String stringJson) throws JSONException {
return (TariffOptionValue) super._fromString(stringJson);
}
@Override
public ArrayItem _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (option == null) {
option = new TariffOption();
}
if (json.has(NAME)) {
option.setName(json.getString(NAME));
}
if (json.has(VALUE)) {
this.value = json.getString(VALUE);
}
return this;
}
public String getOptionValueAsString() {
return this.getOption().getName() + Constants.COLON_SEPARATOR + this.getValue();
}
@Override
public JSONObject _toJSON() throws JSONException {
super._toJSON();
JSONObject json = new JSONObject();
json.put(NAME, getName());
json.put(VALUE, getValue());
return json;
}
public TariffOptionValue clone() {
TariffOptionValue optionValue = new TariffOptionValue();
optionValue.setValue(this.value);
optionValue.setOption(new TariffOption(this.option.getName()));
return optionValue;
}
@Override
public String toString() {
return this.getName() + Constants.COLON_SEPARATOR + this.getValue();
}
@Override
public int compareTo(TariffOptionValue optionValue) {
return this.getOption().getName().compareTo(optionValue.getOption().getName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy