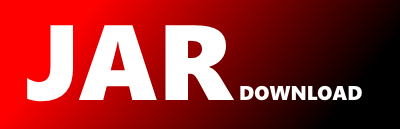
com.jelastic.api.system.statistic.persistence.CurrentNodeStatisticInfo Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 6f8353280bc87816acab2a050bddadc4*/
package com.jelastic.api.system.statistic.persistence;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.data.po.VType;
import org.json.JSONException;
import org.json.JSONObject;
import java.text.ParseException;
import java.util.Date;
/**
* @author Oleksandr Kotvytskyi
*/
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class CurrentNodeStatisticInfo {
public static final String CPU = "cpu";
public static final String CPU_LOAD = "cpuLoad";
public static final String CPU_LIMIT = "cpuLimit";
public static final String MEMORY = "memory";
public static final String MEMORY_LIMIT = "memoryLimit";
public static final String DISK = "disk";
public static final String IOPS = "iops";
public static final String NET_OUT_EXT = "netOutExt";
public static final String NET_OUT_INT = "netOutInt";
public static final String NET_IN_EXT = "netInExt";
public static final String NET_IN_INT = "netInInt";
public static final String CLOUDLETS = "cloudlets";
public static final String DISK_LIMIT = "diskLimit";
public static final String IOPS_LIMIT = "iopsLimit";
public static final String BANDWITH_LIMIT = "bandwithLimit";
public static final String CREATED_ON = "createdOn";
public static final String UPDATED_ON = "updatedOn";
public static final String CURRENT_NODE_STAT_ID = "currentNodeStatId";
public static final String OS_TEMPLATE = "osTemplate";
private long id;
private Integer cpu;
private Integer cpuLoad;
private Integer memory;
private Integer disk;
private Integer iops;
private Long netOutExt;
private Long netOutInt;
private Long netInExt;
private Long netInInt;
private Integer cloudlets;
private Integer cpuLimit;
private Integer memoryLimit;
private Integer diskLimit;
private Integer iopsLimit;
private Integer bandwithLimit;
private Date createdOn;
private Date updatedOn;
private CurrentNodeStatistic currentNodeStatistic;
private String osTemplate;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public Integer getCpu() {
return cpu;
}
public void setCpu(Integer cpu) {
this.cpu = cpu;
}
public Integer getMemory() {
return memory;
}
public void setMemory(Integer memory) {
this.memory = memory;
}
public Integer getDisk() {
return disk;
}
public void setDisk(Integer disk) {
this.disk = disk;
}
public Integer getIops() {
return iops;
}
public void setIops(Integer iops) {
this.iops = iops;
}
public Long getNetOutExt() {
return netOutExt;
}
public void setNetOutExt(Long netOutExt) {
this.netOutExt = netOutExt;
}
public Long getNetOutInt() {
return netOutInt;
}
public void setNetOutInt(Long netOutInt) {
this.netOutInt = netOutInt;
}
public Long getNetInExt() {
return netInExt;
}
public void setNetInExt(Long netInExt) {
this.netInExt = netInExt;
}
public Long getNetInInt() {
return netInInt;
}
public void setNetInInt(Long netInInt) {
this.netInInt = netInInt;
}
public Integer getCloudlets() {
return cloudlets;
}
public void setCloudlets(Integer cloudlets) {
this.cloudlets = cloudlets;
}
public Integer getCpuLimit() {
return cpuLimit;
}
public void setCpuLimit(Integer cpuLimit) {
this.cpuLimit = cpuLimit;
}
public Integer getMemoryLimit() {
return memoryLimit;
}
public void setMemoryLimit(Integer memoryLimit) {
this.memoryLimit = memoryLimit;
}
public Integer getDiskLimit() {
return diskLimit;
}
public void setDiskLimit(Integer diskLimit) {
this.diskLimit = diskLimit;
}
public Integer getIopsLimit() {
return iopsLimit;
}
public void setIopsLimit(Integer iopsLimit) {
this.iopsLimit = iopsLimit;
}
public Integer getBandwithLimit() {
return bandwithLimit;
}
public void setBandwithLimit(Integer bandwithLimit) {
this.bandwithLimit = bandwithLimit;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedOn() {
return updatedOn;
}
public void setUpdatedOn(Date updatedOn) {
this.updatedOn = updatedOn;
}
public String getOsTemplate() {
return osTemplate;
}
public void setOsTemplate(String osTemplate) {
this.osTemplate = osTemplate;
}
public CurrentNodeStatistic getCurrentNodeStatistic() {
return currentNodeStatistic;
}
public void setCurrentNodeStatistic(CurrentNodeStatistic currentNodeStatistic) {
this.currentNodeStatistic = currentNodeStatistic;
}
public Integer getCpuLoad() {
return cpuLoad;
}
public void setCpuLoad(Integer cpuLoad) {
this.cpuLoad = cpuLoad;
}
public JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
if (cpu != null) {
json.put(CPU, cpu);
if (currentNodeStatistic.getvType() == VType.VM && cpuLoad == null) {
cpuLoad = cpu;
}
}
if (cpuLoad != null) {
json.put(CPU_LOAD, cpuLoad);
}
if (memory != null) {
json.put(MEMORY, memory);
}
if (disk != null) {
json.put(DISK, disk);
}
if (iops != null) {
json.put(IOPS, iops);
}
if (netOutExt != null) {
json.put(NET_OUT_EXT, netOutExt);
}
if (netOutInt != null) {
json.put(NET_OUT_INT, netOutInt);
}
if (netInExt != null) {
json.put(NET_IN_EXT, netInExt);
}
if (netInInt != null) {
json.put(NET_IN_INT, netInInt);
}
if (cpuLimit != null) {
json.put(CPU_LIMIT, cpuLimit);
}
if (memoryLimit != null) {
json.put(MEMORY_LIMIT, memoryLimit);
}
if (cloudlets != null) {
json.put(CLOUDLETS, cloudlets);
}
if (diskLimit != null) {
json.put(DISK_LIMIT, diskLimit);
}
if (iopsLimit != null) {
json.put(IOPS_LIMIT, iopsLimit);
}
if (bandwithLimit != null) {
json.put(BANDWITH_LIMIT, bandwithLimit);
}
if (createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(createdOn));
}
if (updatedOn != null) {
json.put(UPDATED_ON, DateUtils.formatSqlDateTime(updatedOn));
}
if (currentNodeStatistic != null) {
json.put(CURRENT_NODE_STAT_ID, currentNodeStatistic.getId());
}
if (osTemplate != null) {
json.put(OS_TEMPLATE, osTemplate);
}
return json;
}
public CurrentNodeStatisticInfo fromJSON(JSONObject jsonObject) throws JSONException {
if (jsonObject.has(CPU)) {
this.setCpu(jsonObject.getInt(CPU));
}
if (jsonObject.has(CPU_LOAD)) {
this.setCpuLoad(jsonObject.getInt(CPU_LOAD));
}
if (jsonObject.has(MEMORY)) {
this.setMemory(jsonObject.getInt(MEMORY));
}
if (jsonObject.has(DISK)) {
this.setDisk(jsonObject.getInt(DISK));
}
if (jsonObject.has(IOPS)) {
this.setIops(jsonObject.getInt(IOPS));
}
if (jsonObject.has(NET_OUT_EXT)) {
this.setNetOutExt(jsonObject.getLong(NET_OUT_EXT));
}
if (jsonObject.has(NET_OUT_INT)) {
this.setNetOutInt(jsonObject.getLong(NET_OUT_INT));
}
if (jsonObject.has(NET_IN_EXT)) {
this.setNetInExt(jsonObject.getLong(NET_IN_EXT));
}
if (jsonObject.has(NET_IN_INT)) {
this.setNetInInt(jsonObject.getLong(NET_IN_INT));
}
if (jsonObject.has(CPU_LIMIT)) {
this.setCpuLimit(jsonObject.getInt(CPU_LIMIT));
}
if (jsonObject.has(MEMORY_LIMIT)) {
this.setMemoryLimit(jsonObject.getInt(MEMORY_LIMIT));
}
if (jsonObject.has(CLOUDLETS)) {
this.setCloudlets(jsonObject.getInt(CLOUDLETS));
}
if (jsonObject.has(DISK_LIMIT)) {
this.setDiskLimit(jsonObject.getInt(DISK_LIMIT));
}
if (jsonObject.has(IOPS_LIMIT)) {
this.setIopsLimit(jsonObject.getInt(IOPS_LIMIT));
}
if (jsonObject.has(BANDWITH_LIMIT)) {
this.setBandwithLimit(jsonObject.getInt(BANDWITH_LIMIT));
}
if (jsonObject.has(CREATED_ON)) {
try {
this.setCreatedOn(DateUtils.parseSqlDateTime(jsonObject.getString(CREATED_ON)));
} catch (ParseException e) {
e.printStackTrace();
}
}
if (jsonObject.has(UPDATED_ON)) {
try {
this.setUpdatedOn(DateUtils.parseSqlDateTime(jsonObject.getString(UPDATED_ON)));
} catch (ParseException e) {
e.printStackTrace();
}
}
if (jsonObject.has(OS_TEMPLATE)) {
this.setOsTemplate(jsonObject.getString(OS_TEMPLATE));
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy