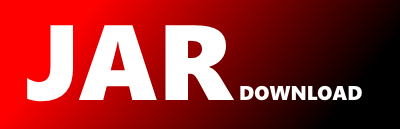
com.jelastic.api.system.statistic.persistence.StatElement Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 91e30a142b61048ab0beed7f2dddb266*/
package com.jelastic.api.system.statistic.persistence;
import com.jelastic.api.common.SystemUtils;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.data.po.VType;
import com.jelastic.api.system.statistic.StatElementInterface;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.text.ParseException;
import java.util.Date;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class StatElement extends AbstractStatEntity implements Comparable, StatElementInterface, Serializable {
public static final String CPU_MHZ = "cpumhz";
public static final String CPU = "cpu";
public static final String NET = "net";
public static final String NET_IN_INT = "in_int";
public static final String NET_OUT_INT = "out_int";
public static final String NET_IN_EXT = "in_ext";
public static final String NET_OUT_EXT = "out_ext";
public static final String MEM = "mem";
public static final String MEM_TOTAL = "memTotal";
public static final String CPU_TOTAL = "cpuTotal";
public static final String DISK = "disk";
public static final String DISK_LIMIT = "disk_limit";
public static final String DURATION = "duration";
public static final String START = "start";
public static final String IOPS_USED = "iops_used";
public static final String IOPS_LIMIT = "iops_limit";
public static final String DISK_IO_USED = "disk_io_used";
public static final String DISK_IO_LIMIT = "disk_io_limit";
public static final String TRAFFIC_LIMIT = "traffic_limit";
public static final String CHANKS_USED = "chanksused";
public static final String CLOUDLETS_USED = "cloudletsused";
public static final String CAPACITY = "capacity";
public static final String V_TYPE = "vType";
public static final String CPU_LOAD = "cpuLoad";
public static final String OS_TEMPLATE = "osTemplate";
private int id = 104857600;
private Date startTime;
private int duration;
private Integer cpuUsed;
private Integer cpuLoad;
private Integer cpuMHz;
private double cpuLimit;
private Integer memUsed;
private Integer memTotal = 0;
private Integer diskUsed;
private Integer diskTotal;
private Integer diskInodesTotal;
private Integer diskInodesUsed;
private Integer diskIopsUsed;
private Integer diskIopsLimit;
private Integer diskIoUsed;
private Integer diskIoLimit;
private Long netInInt;
private Long netOutInt;
private Long netInExt;
private Long netOutExt;
private Integer chanksUsed = -1;
private Integer cloudletsUsed = -1;
private int capacity;
private Integer trafficLimit;
private VType vType = VType.CT;
private String osTemplate;
public StatElement(int duration, int cpuUsed, Integer memUsed, Integer memTotal, Integer diskUsed, Integer diskTotal, Integer diskInodesTotal, Integer diskInodesUsed, Long netInInt, Long netOutInt, Long netInExt, Long netOutExt, Integer diskIopsUsed, Integer diskIopsLimit, Integer trafficLimit, Integer diskIoUsed, Integer diskIoLimit) {
this.duration = duration;
this.cpuUsed = cpuUsed;
this.memUsed = memUsed;
this.diskUsed = diskUsed;
this.netInInt = netInInt;
this.netOutInt = netOutInt;
this.netInExt = netInExt;
this.netOutExt = netOutExt;
this.memTotal = memTotal;
this.diskTotal = diskTotal;
this.diskInodesTotal = diskInodesTotal;
this.diskInodesUsed = diskInodesUsed;
this.diskIopsUsed = diskIopsUsed;
this.diskIopsLimit = diskIopsLimit;
this.diskIoUsed = diskIoUsed;
this.diskIoLimit = diskIoLimit;
this.trafficLimit = trafficLimit;
}
public StatElement(Date startTime, int duration, int cpuUsed, Integer memUsed, Integer memTotal, Integer diskUsed, Integer diskTotal, Integer diskInodesTotal, Integer diskInodesUsed, Long netInInt, Long netOutInt, Long netInExt, Long netOutExt, Integer diskIopsUsed, Integer diskIopsLimit, Integer trafficLimit, Integer diskIoUsed, Integer diskIoLimit) {
this(duration, cpuUsed, memUsed, memTotal, diskUsed, diskTotal, diskInodesTotal, diskInodesUsed, netInInt, netOutInt, netInExt, netOutExt, diskIopsUsed, diskIopsLimit, trafficLimit, diskIoUsed, diskIoLimit);
this.startTime = startTime;
}
public StatElement() {
}
public Integer getCpuUsed() {
return cpuUsed;
}
public void setCpuUsed(int cpuUsed) {
this.cpuUsed = cpuUsed;
}
public void addCpuUsed(int cpuUsed) {
if (this.cpuUsed == null) {
setCpuUsed(cpuUsed);
} else {
this.cpuUsed += cpuUsed;
}
}
public Integer getDiskUsed() {
return diskUsed;
}
public Integer getMemTotal() {
return memTotal;
}
public void setMemTotal(Integer memTotal) {
this.memTotal = memTotal;
}
public Integer getDiskTotal() {
return diskTotal;
}
public void setDiskTotal(Integer diskTotal) {
this.diskTotal = diskTotal;
}
public Integer getDiskInodesTotal() {
return diskInodesTotal;
}
public void setDiskInodesTotal(Integer diskInodesTotal) {
this.diskInodesTotal = diskInodesTotal;
}
public Integer getDiskInodesUsed() {
return diskInodesUsed;
}
public void setDiskInodesUsed(Integer diskInodesUsed) {
this.diskInodesUsed = diskInodesUsed;
}
public Long getNetInInt() {
return netInInt;
}
public void setNetInInt(Long netInInt) {
this.netInInt = netInInt;
}
public Long getNetOutInt() {
return netOutInt;
}
public void setNetOutInt(Long netOutInt) {
this.netOutInt = netOutInt;
}
public Long getNetInExt() {
return netInExt;
}
public void setNetInExt(Long netInExt) {
this.netInExt = netInExt;
}
public Long getNetOutExt() {
return netOutExt;
}
public void setNetOutExt(Long netOutExt) {
this.netOutExt = netOutExt;
}
public void setDiskUsed(int diskUsed) {
this.diskUsed = diskUsed;
}
public int getDuration() {
return duration;
}
public void setDuration(int duration) {
this.duration = duration;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public Integer getMemUsed() {
return memUsed;
}
public void setMemUsed(int memUsed) {
this.memUsed = memUsed;
}
public Integer getTrafficLimit() {
return trafficLimit;
}
public void setTrafficLimit(Integer trafficLimit) {
this.trafficLimit = trafficLimit;
}
public void addNetInInt(Long netInInt) {
if (this.netInInt == null) {
setNetInInt(netInInt);
} else {
this.netInInt += netInInt;
}
}
public void addNetOutInt(Long netOutInt) {
if (this.netOutInt == null) {
setNetOutInt(netOutInt);
} else {
this.netOutInt += netOutInt;
}
}
public void addNetInExt(Long netInExt) {
if (this.netInExt == null) {
setNetInExt(netInExt);
} else {
this.netInExt += netInExt;
}
}
public void addNetOutExt(Long netOutExt) {
if (this.netOutExt == null) {
setNetOutExt(netOutExt);
} else {
this.netOutExt += netOutExt;
}
}
public Date getStartTime() {
return startTime;
}
public void setStartTime(Date startTime) {
this.startTime = startTime;
}
public Integer getChanksUsed() {
return chanksUsed;
}
public void setChanksUsed(Integer chanksUsed) {
this.chanksUsed = chanksUsed;
}
public Integer getCloudletsUsed() {
return cloudletsUsed;
}
public void setCloudletsUsed(Integer cloudletsUsed) {
this.cloudletsUsed = cloudletsUsed;
}
public Integer getCpuMHz() {
this.cpuMHz = 0;
if (this.cpuUsed != null && this.duration > 0 && this.vType == VType.CT) {
this.cpuMHz = Math.round(this.cpuUsed / (float) this.duration);
}
return cpuMHz;
}
public Integer getCapacity() {
return capacity;
}
public void setCapacity(Integer capacity) {
this.capacity = capacity;
}
public void calcCloudletsUsed(int maxCloudlets, int memPerCloudlet, int cpuPerCloudlet) {
int memCloudlets = 0;
int cpuCloudlets = 0;
if (this.memUsed != null) {
memCloudlets = SystemUtils.getCloudletsCountByMemory(memUsed, memPerCloudlet * 1024l);
}
cpuCloudlets = SystemUtils.getCloudletsCountByCPU(this.getCpuMHz(), cpuPerCloudlet);
if (memCloudlets > cpuCloudlets) {
this.chanksUsed = memCloudlets;
this.cloudletsUsed = memCloudlets;
} else {
this.chanksUsed = cpuCloudlets;
this.cloudletsUsed = cpuCloudlets;
}
if (maxCloudlets > -1 && this.chanksUsed > maxCloudlets) {
this.chanksUsed = maxCloudlets;
this.cloudletsUsed = maxCloudlets;
}
}
public boolean isCompleted() {
if (cpuUsed != null && memUsed != null && diskUsed != null && netInInt != null && netOutInt != null && netInExt != null && netOutExt != null && memTotal != null && diskTotal != null && diskInodesTotal != null && diskInodesUsed != null && diskIopsUsed != null && diskIopsLimit != null && diskIoUsed != null && diskIoLimit != null && trafficLimit != null) {
return true;
} else {
return false;
}
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
json.put(CPU_MHZ, this.getCpuMHz());
if (cpuUsed != null) {
json.put(CPU, cpuUsed);
if (vType == VType.VM && cpuLoad == null) {
cpuLoad = cpuUsed;
}
} else {
json.put(CPU, 0);
}
if (cpuLoad != null) {
json.put(CPU_LOAD, cpuLoad);
}
if (memUsed != null) {
json.put(MEM, memUsed);
} else {
json.put(MEM, 0);
}
if (memTotal != null) {
json.put(MEM_TOTAL, memTotal);
}
if (chanksUsed == null) {
json.put(CHANKS_USED, 0);
json.put(CLOUDLETS_USED, 0);
} else if (chanksUsed != -1) {
json.put(CHANKS_USED, chanksUsed);
json.put(CLOUDLETS_USED, cloudletsUsed);
}
if (diskUsed != null) {
json.put(DISK, diskUsed);
json.put(CAPACITY, SystemUtils.getCapacityCount(diskUsed));
} else {
json.put(DISK, 0);
}
if (diskIopsUsed != null) {
json.put(IOPS_USED, diskIopsUsed);
} else {
json.put(IOPS_USED, 0);
}
JSONObject netJson = new JSONObject();
if (netInInt != null) {
netJson.put(NET_IN_INT, netInInt);
} else {
netJson.put(NET_IN_INT, 0);
}
if (netOutInt != null) {
netJson.put(NET_OUT_INT, netOutInt);
} else {
netJson.put(NET_OUT_INT, 0);
}
if (netInExt != null) {
netJson.put(NET_IN_EXT, netInExt);
} else {
netJson.put(NET_IN_EXT, 0);
}
if (netOutExt != null) {
netJson.put(NET_OUT_EXT, netOutExt);
} else {
netJson.put(NET_OUT_EXT, 0);
}
json.put(NET, netJson);
if (duration > 0) {
json.put(DURATION, duration);
} else {
json.put(DURATION, 0);
}
if (startTime != null) {
json.put(START, DateUtils.formatSqlDateTime(startTime));
} else {
json.put(START, "");
}
if (diskIoUsed != null) {
json.put(DISK_IO_USED, diskIoUsed);
} else {
json.put(DISK_IO_USED, 0);
}
if (vType != null) {
json.put(V_TYPE, vType);
}
if (osTemplate != null) {
json.put(OS_TEMPLATE, osTemplate);
}
return json;
}
@Override
public StatElement _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(CPU_MHZ)) {
cpuMHz = json.getInt(CPU_MHZ);
}
if (json.has(CPU)) {
cpuUsed = json.getInt(CPU);
}
if (json.has(CPU_LOAD)) {
cpuUsed = json.getInt(CPU_LOAD);
}
if (json.has(MEM)) {
memUsed = json.getInt(MEM);
}
if (json.has(MEM_TOTAL)) {
memTotal = json.getInt(MEM_TOTAL);
}
if (json.has(CHANKS_USED)) {
chanksUsed = json.getInt(CHANKS_USED);
}
if (json.has(CLOUDLETS_USED)) {
cloudletsUsed = json.getInt(CLOUDLETS_USED);
}
if (json.has(CAPACITY)) {
capacity = json.getInt(CAPACITY);
}
if (json.has(DISK)) {
diskUsed = json.getInt(DISK);
}
if (json.has(IOPS_USED)) {
diskIopsUsed = json.getInt(IOPS_USED);
}
if (json.has(NET)) {
JSONObject jsonNet = json.getJSONObject(NET);
if (jsonNet.has(NET_IN_INT)) {
netInInt = jsonNet.getLong(NET_IN_INT);
}
if (jsonNet.has(NET_OUT_INT)) {
netOutInt = jsonNet.getLong(NET_OUT_INT);
}
if (jsonNet.has(NET_IN_EXT)) {
netInExt = jsonNet.getLong(NET_IN_EXT);
}
if (jsonNet.has(NET_OUT_EXT)) {
netOutExt = jsonNet.getLong(NET_OUT_EXT);
}
}
if (json.has(DURATION)) {
duration = json.getInt(DURATION);
}
if (json.has(START) && !json.getString(START).isEmpty()) {
try {
startTime = DateUtils.parseSqlDateTime(json.getString(START));
} catch (ParseException ex) {
throw new JSONException(ex);
}
}
if (json.has(DISK_IO_USED)) {
diskIoUsed = json.getInt(DISK_IO_USED);
}
if (json.has(V_TYPE)) {
vType = VType.valueOf(json.getString(V_TYPE));
}
if (json.has(OS_TEMPLATE)) {
osTemplate = json.getString(OS_TEMPLATE);
}
return this;
}
public void merge(StatElementInterface stat) {
StatElement element = (StatElement) stat;
this.duration += element.duration;
if (this.vType == VType.VM) {
this.cpuUsed = (this.cpuUsed + element.getCpuUsed()) / 2;
} else {
this.cpuUsed += element.getCpuUsed();
}
this.memUsed = (this.memUsed + element.getMemUsed()) / 2;
this.memTotal = (this.memTotal + element.getMemTotal()) / 2;
this.diskUsed = (this.diskUsed + element.getDiskUsed()) / 2;
this.netInInt += element.getNetInInt();
this.netOutInt += element.getNetOutInt();
this.netInExt += element.getNetInExt();
this.netOutExt += element.getNetOutExt();
this.diskIopsUsed = (this.diskIopsUsed + element.getDiskIopsUsed()) / 2;
this.diskIoUsed = (this.diskIoUsed + element.getDiskIoUsed());
}
public void mergePeak(StatElement element) {
this.duration += element.duration;
this.cpuUsed = (this.cpuUsed + element.getCpuUsed()) / 2;
this.memUsed = this.memUsed > element.getMemUsed() ? this.memUsed : element.getMemUsed();
this.diskUsed = this.diskUsed > element.getDiskUsed() ? this.diskUsed : element.getDiskUsed();
this.netInInt += element.getNetInInt();
this.netOutInt += element.getNetOutInt();
this.netInExt += element.getNetInExt();
this.netOutExt += element.getNetOutExt();
this.diskIopsUsed += element.getDiskIopsUsed();
this.diskIoUsed += element.getDiskIoUsed();
}
public void addition(StatElementInterface stat) {
StatElement element = (StatElement) stat;
this.duration = (this.duration + element.duration) / 2;
if (this.vType == VType.VM) {
this.cpuUsed = (this.cpuUsed + element.getCpuUsed()) / 2;
} else {
this.cpuUsed += element.getCpuUsed();
}
this.memUsed += element.getMemUsed();
this.memTotal += element.getMemTotal();
this.diskUsed += element.getDiskUsed();
this.netInInt += element.getNetInInt();
this.netOutInt += element.getNetOutInt();
this.netInExt += element.getNetInExt();
this.netOutExt += element.getNetOutExt();
this.diskIopsUsed += element.getDiskIopsUsed();
this.diskIoUsed += element.getDiskIoUsed();
}
public void fillWithZero() {
fillWithZero(false);
}
public void fillWithZero(boolean isReplace) {
if (isReplace || this.memUsed == null) {
this.memUsed = 0;
}
if (isReplace || this.cpuUsed == null) {
this.cpuUsed = 0;
}
if (isReplace || this.duration == -1) {
this.duration = 0;
}
if (isReplace || this.netInInt == null) {
this.netInInt = 0l;
}
if (isReplace || this.netOutInt == null) {
this.netOutInt = 0l;
}
if (isReplace || this.netInExt == null) {
this.netInExt = 0l;
}
if (isReplace || this.netOutExt == null) {
this.netOutExt = 0l;
}
if (isReplace || this.diskUsed == null) {
this.diskUsed = 0;
}
if (isReplace || this.diskTotal == null) {
this.diskTotal = 0;
}
if (isReplace || this.memTotal == null) {
this.memTotal = 0;
}
if (isReplace || this.diskInodesTotal == null) {
this.diskInodesTotal = 0;
}
if (isReplace || this.diskInodesUsed == null) {
this.diskInodesUsed = 0;
}
if (isReplace || this.diskIopsUsed == null) {
this.diskIopsUsed = 0;
}
if (isReplace || this.diskIopsLimit == null) {
this.diskIopsLimit = 0;
}
if (isReplace || this.trafficLimit == null) {
this.trafficLimit = 0;
}
if (isReplace || this.diskIoUsed == null) {
this.diskIoUsed = 0;
}
}
public int compareTo(StatElement t) {
return this.startTime.compareTo(t.getStartTime());
}
@Override
public StatElement clone() {
StatElement statElement = new StatElement(this.duration, this.cpuUsed, this.memUsed, this.memTotal, this.diskUsed, this.diskTotal, this.diskInodesTotal, this.diskInodesUsed, this.netInInt, this.netOutInt, this.netInExt, this.netOutExt, this.diskIopsUsed, this.diskIopsLimit, this.trafficLimit, this.diskIoUsed, this.diskIoLimit);
statElement.setId(this.id);
statElement.setStartTime(this.startTime);
statElement.setChanksUsed(this.chanksUsed);
statElement.setCloudletsUsed(this.cloudletsUsed);
statElement.setEnvId(super.getEnvId());
statElement.setvType(this.getvType());
statElement.setOsTemplate(this.getOsTemplate());
return statElement;
}
@Override
public Date getCollectDate() {
return getStartTime();
}
@Override
public void setCollectDate(Date collectDate) {
setStartTime(collectDate);
}
public void setDiskIopsUsed(Integer diskIopsUsed) {
this.diskIopsUsed = diskIopsUsed;
}
public void setDiskIopsLimit(Integer diskIopsLimit) {
this.diskIopsLimit = diskIopsLimit;
}
public Integer getDiskIopsLimit() {
return diskIopsLimit;
}
public Integer getDiskIopsUsed() {
return diskIopsUsed;
}
public Integer getDiskIoUsed() {
return diskIoUsed;
}
public void setDiskIoUsed(Integer diskIoUsed) {
this.diskIoUsed = diskIoUsed;
}
public Integer getDiskIoLimit() {
return diskIoLimit;
}
public void setDiskIoLimit(Integer diskIoLimit) {
this.diskIoLimit = diskIoLimit;
}
public double getCpuLimit() {
return cpuLimit;
}
public void setCpuLimit(double cpuLimit) {
this.cpuLimit = cpuLimit;
}
public VType getvType() {
return vType;
}
public void setvType(VType vType) {
this.vType = vType;
}
public String getOsTemplate() {
return osTemplate;
}
public void setOsTemplate(String osTemplate) {
this.osTemplate = osTemplate;
}
@Override
public String toString() {
return "StatElement{" + "id=" + id + ", envId=" + getEnvId() + ", startTime=" + startTime + ", duration=" + duration + ", cpuUsed=" + cpuUsed + ", cpuLoad=" + cpuLoad + ", cpuMHz=" + cpuMHz + ", cpuLimit=" + cpuLimit + ", memUsed=" + memUsed + ", memTotal=" + memTotal + ", diskUsed=" + diskUsed + ", diskTotal=" + diskTotal + ", diskInodesTotal=" + diskInodesTotal + ", diskInodesUsed=" + diskInodesUsed + ", diskIopsUsed=" + diskIopsUsed + ", diskIopsLimit=" + diskIopsLimit + ", diskIoUsed=" + diskIoUsed + ", diskIoLimit=" + diskIoLimit + ", netInInt=" + netInInt + ", netOutInt=" + netOutInt + ", netInExt=" + netInExt + ", netOutExt=" + netOutExt + ", chanksUsed=" + chanksUsed + ", cloudletsUsed=" + cloudletsUsed + ", capacity=" + capacity + ", trafficLimit=" + trafficLimit + ", vType=" + vType + ", osTemplate=" + osTemplate + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy