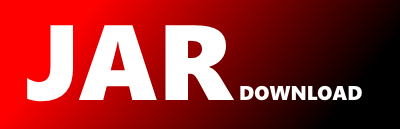
com.jelastic.api.system.utils.GridUtils Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 7d5b3540e07e11d990cd1087cc74e887*/
package com.jelastic.api.system.utils;
import com.jelastic.api.common.Constants;
import com.jelastic.api.system.persistence.pricing.TariffGrid;
import com.jelastic.api.system.persistence.pricing.TariffGridItem;
import com.jelastic.api.system.persistence.pricing.TariffOption;
import com.jelastic.api.system.persistence.pricing.TariffOptionValue;
import com.jelastic.api.utils.MD5;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class GridUtils {
public static final String ITEM_NAME = "name";
public static final String ITEM_OPTIONS = "options";
public static final String VM_MEMORY_OPTION = "vm_memory";
public static final String VM_CPU_OPTION = "vm_vcpu";
public static final String VM_EXTERNAL_RESOURCE_NAME = "VM";
public static String getOptionValueByName(TariffGridItem item, String name) {
TariffOptionValue value = item.getOptions().stream().filter(o -> o.getName().equals(name)).findFirst().orElse(null);
return value == null ? null : value.getValue();
}
public static Set parseOptions(String options) {
Set items = new HashSet<>();
try {
JSONArray optionsJSON = new JSONArray(options);
for (int i = 0; i < optionsJSON.length(); i++) {
TariffGridItem item = new TariffGridItem();
try {
JSONObject option = optionsJSON.getJSONObject(i);
if (option.has(ITEM_NAME)) {
item.setName(option.getString(ITEM_NAME));
}
JSONObject opts = null;
if (option.has(ITEM_OPTIONS)) {
opts = option.getJSONObject(ITEM_OPTIONS);
} else {
opts = option;
}
Set values = new HashSet<>();
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy